Create and Publish Business Websites in seconds. AI will gather all the details about your website and generate link to your website.
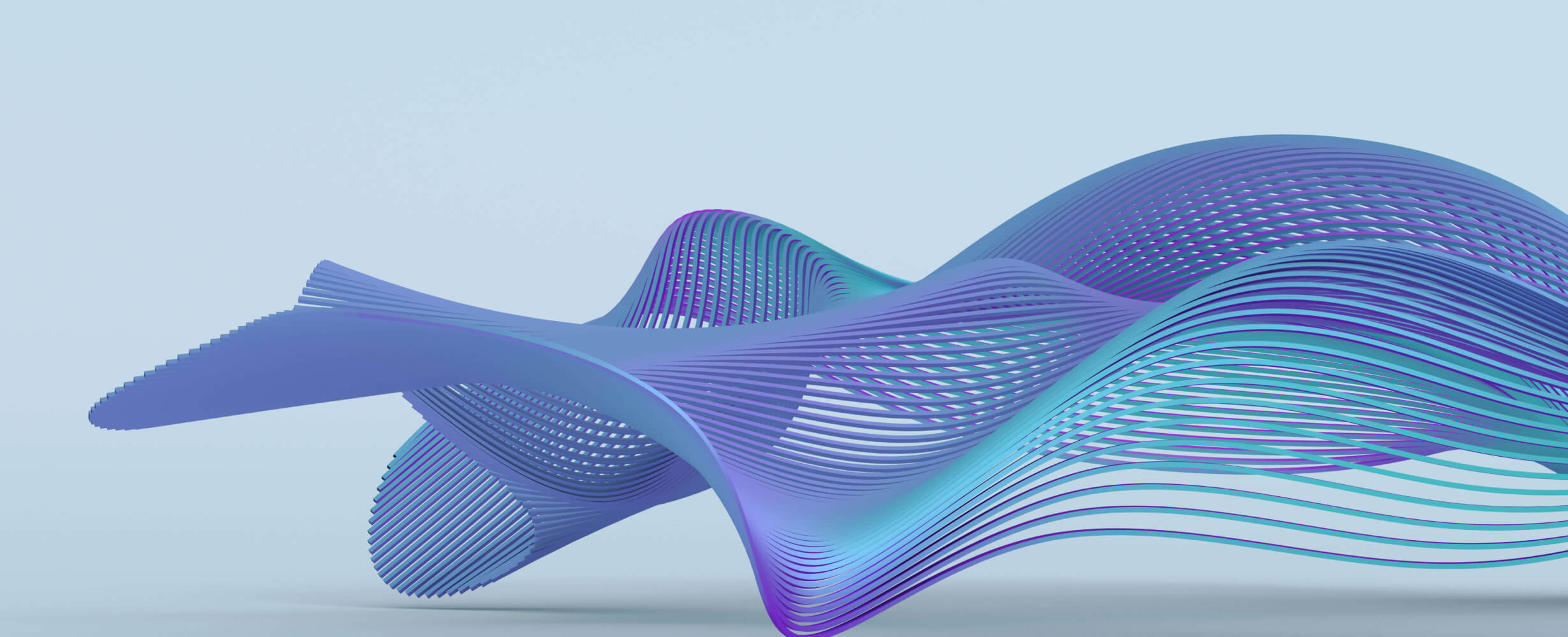
simple-mas
A lightweight and extensible Python SDK for building Multi-Agent Systems, simplifying communication and integration, with first-class support for the Model Context Protocol (MCP)
0
Github Watches
0
Github Forks
0
Github Stars
SimpleMAS
A lightweight SDK for building Multi-Agent Systems with a focus on the Model Context Protocol (MCP).
Features
- Agent communication - HTTP, WebSockets, and MCP-based communication between agents
- Environment-based configuration - Configure agents using environment variables
- Belief-Desire-Intention (BDI) agents - Build agents that reason about beliefs, desires, and intentions
- Reasoning integration - Easily integrate LLMs and other reasoning systems
- Deployment tools - Generate and orchestrate deployment configurations for Docker Compose and Kubernetes
Installation
pip install simple-mas
Or with Poetry:
poetry add simple-mas
Usage
Basic Agent
from simple_mas import Agent
from simple_mas.communication import HTTPCommunicator
agent = Agent(
name="my-agent",
communicator=HTTPCommunicator(
agent_name="my-agent",
service_urls={"other-agent": "http://other-agent:8000/"}
)
)
# Add capabilities, start the agent, etc.
Deployment Orchestration
SimpleMas includes powerful tools for deploying multi-agent systems:
# Discover SimpleMas components in your project
simplemas discover --directory ./my-project
# Orchestrate components into a single Docker Compose file
simplemas orchestrate --directory ./my-project --output docker-compose.yml
# Generate Kubernetes manifests for a component
simplemas k8s --input ./my-project/agent/simplemas.deploy.yaml --output k8s/
Documentation
For more details, see the documentation.
Quick Start
from simple_mas.agent import BaseAgent
from simple_mas.communication import McpSseCommunicator
class MyAgent(BaseAgent):
async def setup(self) -> None:
# Initialize your agent here
pass
async def run(self) -> None:
# Run your agent's main logic
response = await self.communicator.send_request(
"chess-engine",
"make_move",
{"board_state": "..."}
)
async def shutdown(self) -> None:
# Clean up resources
pass
# Run the agent
async def main():
agent = MyAgent(name="my-orchestrator")
await agent.start()
if __name__ == "__main__":
import asyncio
asyncio.run(main())
Development
# Clone the repository
git clone https://github.com/yourusername/simple-mas.git
cd simple-mas
# Install dependencies
poetry install
# Install pre-commit hooks
poetry run pre-commit install
# Run tests
poetry run pytest
# Run all code quality checks at once
./scripts/check_quality.sh
# Or run individual checks
poetry run black src tests
poetry run isort src tests
poetry run flake8 src tests
poetry run mypy src
Code Quality
This project uses several tools to ensure code quality:
- black: Code formatting
- isort: Import sorting
- flake8: Linting
- mypy: Type checking
- pytest: Testing
Pre-commit hooks are configured to run these checks automatically before each commit.
You can also run all checks manually using the ./scripts/check_quality.sh
script.
License
MIT
相关推荐
You're in a stone cell – can you get out? A classic choose-your-adventure interactive fiction game, based on a meticulously-crafted playbook. With a medieval fantasy setting, infinite choices and outcomes, and dice!
Text your favorite pet, after answering 10 questions about their everyday lives!
Carbon footprint calculations breakdown and advices on how to reduce it
Best-in-class AI domain names scoring engine and availability checker. Brandability, domain worth, root keywords and more.
Evaluates language quality of texts, responds with a numerical score between 50-150.
Discover A More Robust Business: Craft tailored value proposition statements, develop a comprehensive business model canvas, conduct detailed PESTLE analysis, and gain strategic insights on enhancing business model elements like scalability, cost structure, and market competition strategies. (v1.18)
🧑🚀 全世界最好的LLM资料总结(Agent框架、辅助编程、数据处理、模型训练、模型推理、o1 模型、MCP、小语言模型、视觉语言模型) | Summary of the world's best LLM resources.
Dify is an open-source LLM app development platform. Dify's intuitive interface combines AI workflow, RAG pipeline, agent capabilities, model management, observability features and more, letting you quickly go from prototype to production.
an easy-to-use dynamic service discovery, configuration and service management platform for building AI cloud native applications.
🔥 1Panel provides an intuitive web interface and MCP Server to manage websites, files, containers, databases, and LLMs on a Linux server.
This project was created to demonstrate how we can connect with different Model Context Protocols (MCPs).
⛓️RuleGo is a lightweight, high-performance, embedded, next-generation component orchestration rule engine framework for Go.
PDF scientific paper translation with preserved formats - 基于 AI 完整保留排版的 PDF 文档全文双语翻译,支持 Google/DeepL/Ollama/OpenAI 等服务,提供 CLI/GUI/MCP/Docker/Zotero
Reviews
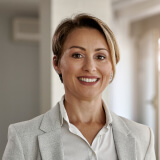
user_fPw0dFsR
I've been using simple-mas by wilson-urdaneta and it has significantly improved my workflow. The straightforward design and easy-to-navigate interface make it a pleasure to use. I appreciate the effort put into making this application user-friendly. Highly recommend it to anyone looking for a reliable and efficient solution!
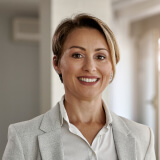
user_1M1LPDTK
I've been using simple-mas by wilson-urdaneta and it has exceeded my expectations. The ease of use and intuitive interface makes it a standout tool. Whether you're new to this type of application or an experienced user, you'll appreciate the thoughtful design and functionality. Highly recommend giving it a try!
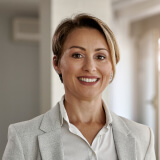
user_xkpEMP4L
I've been using Simple-MAS by Wilson Urdaneta and it has completely exceeded my expectations. This application is user-friendly, efficient, and a reliable tool for managing multiple tasks seamlessly. Highly recommended for anyone looking for simplicity and functionality in one package.
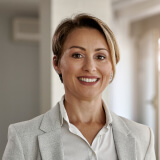
user_e8zy1gOH
As a dedicated user of MCP applications, I highly recommend "simple-mas" by Wilson Urdaneta. This straightforward and efficient product stands out for its user-friendly interface and robust functionality. It's perfect for those who need a reliable solution with minimal hassle. The welcome message sets a positive tone, and the starting URL makes it easy to jump right in. Overall, "simple-mas" delivers on its promises and enhances productivity seamlessly.
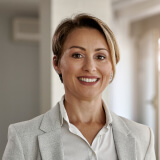
user_SG2jVPPz
As a dedicated user of MCP applications, I highly recommend simple-mas by wilson-urdaneta. Its intuitive design and seamless user experience make it an excellent tool for both beginners and advanced users. The straightforward navigation and helpful welcome guide ensure that users can easily get started and benefit from its features right away. Simple-mas truly stands out for its efficiency and user-friendly interface. A must-try for anyone looking to streamline their processes!