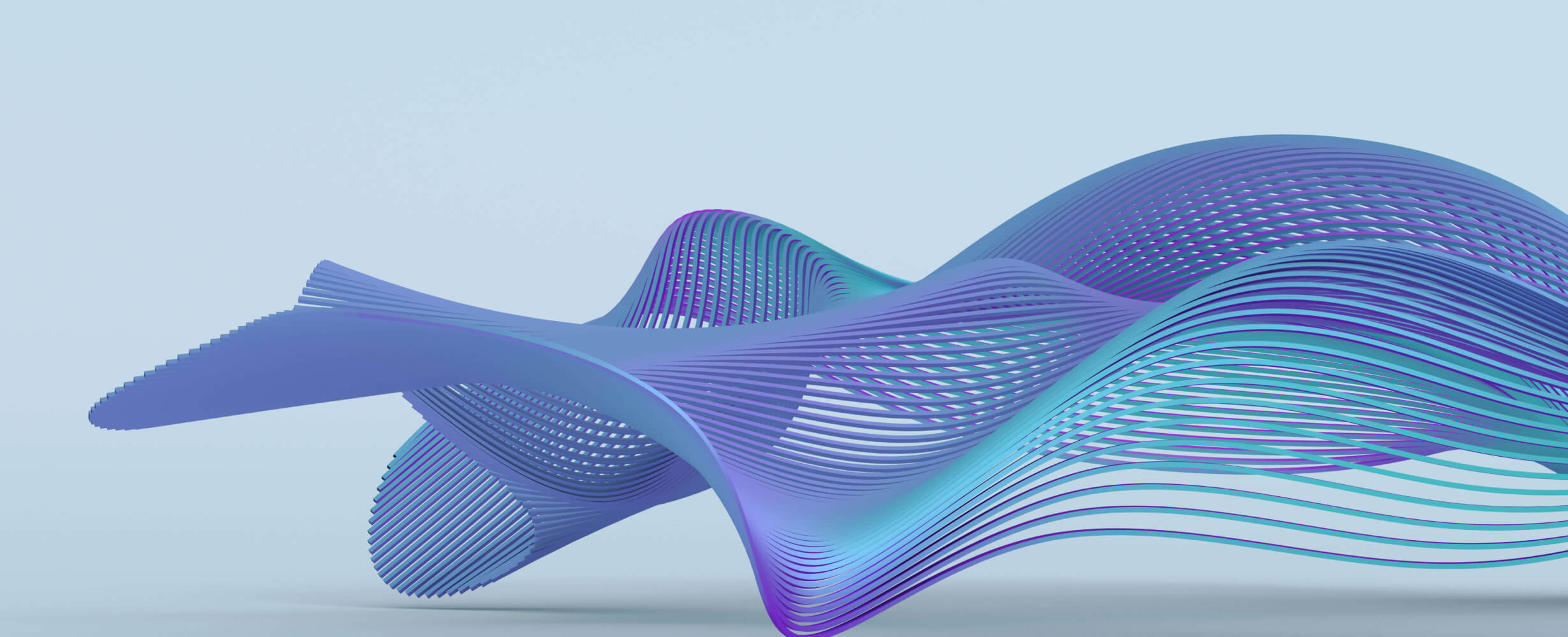
mcp-server-newrelic
The Unofficial MCP Server for New Relic (Alpha)
1
Github Watches
0
Github Forks
0
Github Stars
New Relic NerdGraph MCP Server
This repository provides a Model Context Protocol (MCP) server for interacting with the New Relic NerdGraph API. It allows MCP clients (like Claude Desktop) to use natural language or specific commands to query and interact with your New Relic account data and features.
Built using the fastmcp framework.
Features
This MCP server exposes various New Relic capabilities as tools and resources, including:
- Account Details: Fetch basic information about the configured account.
- Generic NerdGraph Queries: Execute arbitrary NerdGraph queries.
- NRQL Queries: Run specific NRQL queries against your account data.
- Entity Management: Search for entities (Applications, Hosts, Monitors, etc.) and retrieve detailed information by GUID.
- APM: List Application Performance Monitoring (APM) applications.
- Synthetics: List Synthetic monitors and create simple browser monitors.
- Alerts: List alert policies, view open incidents, and acknowledge incidents.
Prerequisites
-
Python: Python 3.10+ recommended (as required by
fastmcp
). - pip: Python package installer.
- New Relic Account: Access to a New Relic account.
- New Relic User API Key: A User API key is required for authentication. You can generate one here. Keep this key secure!
- New Relic Account ID: Your New Relic Account ID is needed for most operations. You can find it in the New Relic UI (often in the URL or account settings).
Setup & Installation
-
Clone the Repository:
git clone <repository_url> # Replace <repository_url> with the actual URL cd mcp-server-newrelic
-
Install Dependencies:
pip install -r requirements.txt
This will install
fastmcp
,requests
, and their dependencies.
Configuration
The server requires your New Relic API Key and Account ID to function. Configure these using environment variables before running the server:
# Replace YOUR_API_KEY and YOUR_ACCOUNT_ID with your actual credentials
export NEW_RELIC_API_KEY="YOUR_API_KEY"
export NEW_RELIC_ACCOUNT_ID="YOUR_ACCOUNT_ID"
Security Note: Do not hardcode your API key in the source code. Using environment variables is the recommended approach. You can also use tools like direnv
or place these export
commands in your shell profile (.zshrc
, .bashrc
, etc.) for persistence, but be mindful of the security implications.
Running the Server
Once configured, start the server using the fastmcp
command-line tool:
fastmcp run server.py:mcp
-
server.py
: The main entry point script. -
mcp
: The name of theFastMCP
instance created withinserver.py
.
The server will start, print registration messages, and listen for incoming MCP connections (typically on port 8000 by default, managed by fastmcp
). You should see output similar to:
Using New Relic Account ID: YOUR_ACCOUNT_ID
Registering common features...
Registering entity features...
Registering APM features...
Registering Synthetics features...
Registering Alerts features...
Feature registration complete.
INFO: Started server process [XXXXX]
INFO: Waiting for application startup.
INFO: Application startup complete.
INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit)
Leave this terminal window running.
Usage with MCP Clients
-
Start the MCP Server (as described above).
-
Restart your MCP Client (e.g., Claude Desktop).
-
The client should automatically detect the running server and connect to it. You might see an indicator (like a 🔨 icon in Claude Desktop) showing that external tools are available.
-
You can now interact with your New Relic account using natural language or by directly invoking the tools listed below.
- Natural Language Example: "Show me my APM applications" or "List open critical incidents in account 1234567"
-
Direct Invocation (if supported):
list_apm_applications()
orlist_open_incidents(priority='CRITICAL', target_account_id=1234567)
Available Tools & Resources
The server provides the following functions accessible via the Model Context Protocol:
Common (features/common.py
)
-
Tool:
query_nerdgraph
- Description: Executes an arbitrary NerdGraph query. Use for operations not covered by specific tools.
-
Arguments:
-
nerdgraph_query
(str): The GraphQL query string. -
variables
(Optional[Dict]): JSON dictionary of variables for the query.
-
- Returns: JSON string of the query result.
-
Tool:
run_nrql_query
- Description: Executes a NRQL query.
-
Arguments:
-
nrql
(str): The NRQL query string (e.g.,"SELECT count(*) FROM Transaction TIMESERIES"
). -
target_account_id
(Optional[int]): Account ID to query (uses default from env if omitted).
-
- Returns: JSON string of the NRQL result.
-
Resource:
get_account_details
- Description: Provides basic details (ID, name) for the configured New Relic account.
-
URI:
newrelic://account_details
-
Returns: JSON string containing account details (
{"data": {"id": ..., "name": ...}}
) or an error.
Entities (features/entities.py
)
-
Tool:
search_entities
- Description: Searches for New Relic entities based on criteria.
-
Arguments:
-
name
(Optional[str]): Filter by name (fuzzy match). -
entity_type
(Optional[str]): Filter by type (e.g.,'APPLICATION'
,'HOST'
). -
domain
(Optional[str]): Filter by domain (e.g.,'APM'
,'INFRA'
). -
tags
(Optional[List[Dict]]): Filter by tags (e.g.,[{"key": "env", "value": "prod"}]
). -
target_account_id
(Optional[int]): Explicit account ID to search within. -
limit
(int): Max results (default 50).
-
- Returns: JSON string of search results.
-
Resource:
get_entity_details
- Description: Retrieves detailed information for a specific entity. Includes common fields and type-specific details for APM, Browser, Infra, Synthetics, Dashboards, etc.
-
URI:
newrelic://entity/{guid}
(replace{guid}
with the entity GUID) - Returns: JSON string of entity details.
-
Prompt:
generate_entity_search_query
-
Description: Generates the
query
string condition part for theentitySearch
NerdGraph field, useful for constructing search queries. -
Arguments:
-
entity_name
(str): Name to search for (exact match used in prompt generation). -
entity_domain
(Optional[str]): Domain to include in the query condition. -
entity_type
(Optional[str]): Type to include in the query condition. -
target_account_id
(Optional[int]): Account ID to include in the query condition.
-
-
Returns: String representing the search condition (e.g.,
"accountId = 123 AND name = 'My App' AND domain = 'APM'"
).
-
Description: Generates the
APM (features/apm.py
)
-
Tool:
list_apm_applications
- Description: Lists APM applications.
-
Arguments:
-
target_account_id
(Optional[int]): Account ID to query (uses default if omitted).
-
- Returns: JSON string containing a list of APM applications.
Synthetics (features/synthetics.py
)
-
Tool:
list_synthetics_monitors
- Description: Lists Synthetic monitors.
-
Arguments:
-
target_account_id
(Optional[int]): Account ID to query (uses default if omitted).
-
- Returns: JSON string containing a list of Synthetic monitors.
-
Tool:
create_simple_browser_monitor
- Description: Creates a basic Synthetics simple browser monitor.
-
Arguments:
-
monitor_name
(str): Name for the new monitor. -
url
(str): URL to monitor. -
locations
(List[str]): List of public location labels (e.g.,["AWS_US_EAST_1"]
). -
period
(str): Check frequency (e.g.,"EVERY_15_MINUTES"
). Default:"EVERY_15_MINUTES"
. -
status
(str): Initial status ("ENABLED"
or"DISABLED"
). Default:"ENABLED"
. -
target_account_id
(Optional[int]): Account ID for creation (uses default if omitted). -
tags
(Optional[List[Dict]]): Optional tags (e.g.,[{"key": "team", "value": "ops"}]
).
-
- Returns: JSON string with the result, including the new monitor's GUID.
-
Resource: Synthetics monitor details can be retrieved using the
get_entity_details
resource with the monitor's GUID (newrelic://entity/{monitor_guid}
).
Alerts (features/alerts.py
)
-
Tool:
list_alert_policies
- Description: Lists alert policies, optionally filtering by name.
-
Arguments:
-
target_account_id
(Optional[int]): Account ID to query (uses default if omitted). -
policy_name_filter
(Optional[str]): Filter policies where name contains this string.
-
- Returns: JSON string containing a list of alert policies.
-
Tool:
list_open_incidents
- Description: Lists currently open alert incidents.
-
Arguments:
-
target_account_id
(Optional[int]): Account ID to query (uses default if omitted). -
priority
(Optional[str]): Filter by priority ('CRITICAL'
,'WARNING'
).
-
- Returns: JSON string containing a list of open incidents.
-
Tool:
acknowledge_alert_incident
- Description: Acknowledges an open alert incident.
-
Arguments:
-
incident_id
(int): The ID of the incident to acknowledge. -
target_account_id
(Optional[int]): Account ID where the incident occurred (uses default if omitted). -
message
(Optional[str]): Optional message for the acknowledgement.
-
- Returns: JSON string with the result of the acknowledgement.
Contributing
Contributions are welcome! Please feel free to submit issues or pull requests.
- Fork the repository.
- Create a new branch (
git checkout -b feature/your-feature
). - Make your changes.
- Commit your changes (
git commit -am 'Add some feature'
). - Push to the branch (
git push origin feature/your-feature
). - Open a Pull Request.
License
This project is licensed under the MIT License - see the LICENSE file for details (if a LICENSE file exists).
相关推荐
I find academic articles and books for research and literature reviews.
Confidential guide on numerology and astrology, based of GG33 Public information
Converts Figma frames into front-end code for various mobile frameworks.
Advanced software engineer GPT that excels through nailing the basics.
Take an adjectivised noun, and create images making it progressively more adjective!
Embark on a thrilling diplomatic quest across a galaxy on the brink of war. Navigate complex politics and alien cultures to forge peace and avert catastrophe in this immersive interstellar adventure.
Discover the most comprehensive and up-to-date collection of MCP servers in the market. This repository serves as a centralized hub, offering an extensive catalog of open-source and proprietary MCP servers, complete with features, documentation links, and contributors.
Micropython I2C-based manipulation of the MCP series GPIO expander, derived from Adafruit_MCP230xx
The all-in-one Desktop & Docker AI application with built-in RAG, AI agents, No-code agent builder, MCP compatibility, and more.
Bridge between Ollama and MCP servers, enabling local LLMs to use Model Context Protocol tools
🧑🚀 全世界最好的LLM资料总结(Agent框架、辅助编程、数据处理、模型训练、模型推理、o1 模型、MCP、小语言模型、视觉语言模型) | Summary of the world's best LLM resources.
Reviews
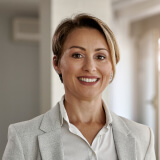
user_Y6xeDULZ
As a dedicated user of mcp applications, I must say mcp-server-newrelic by ulucaydin is a game-changer for server monitoring. The integration with New Relic makes tracking performance metrics seamless and efficient. The thorough documentation available at https://github.com/ulucaydin/mcp-server-newrelic ensures a smooth setup process. Highly recommend it for anyone looking to streamline their server monitoring capabilities!