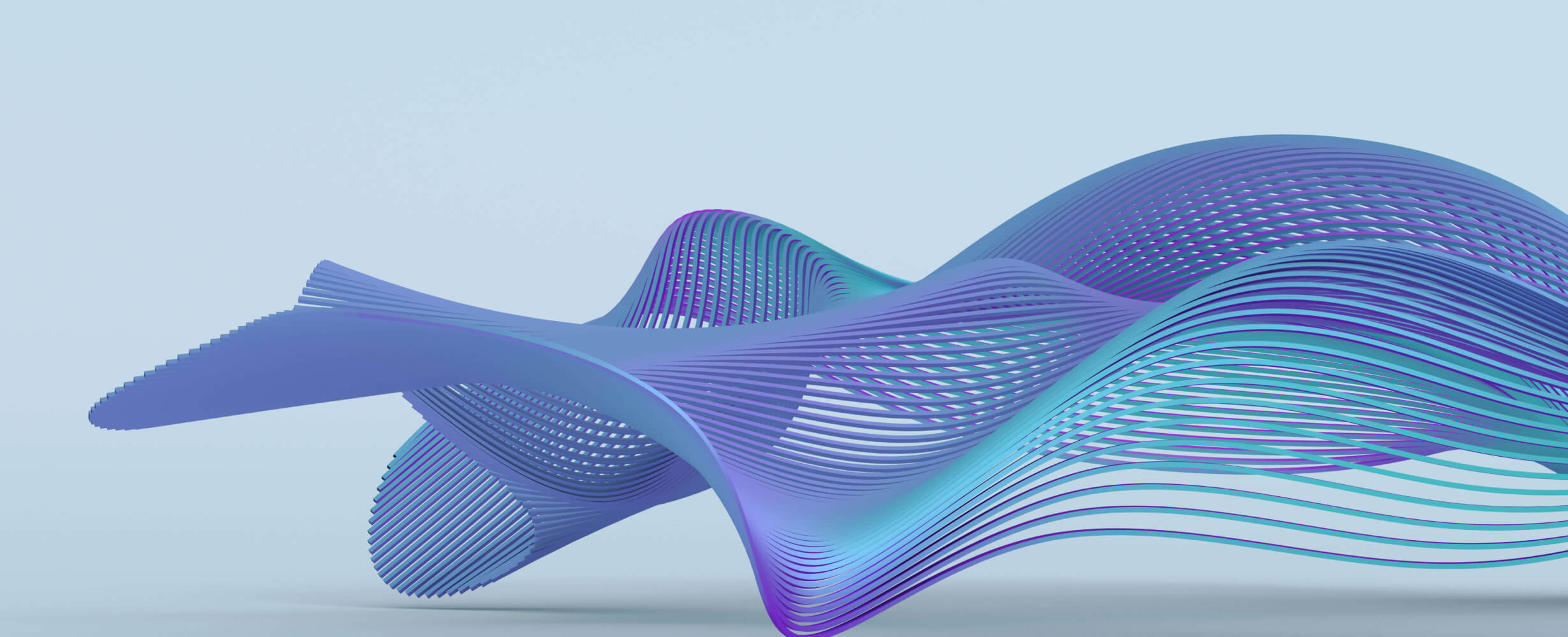
go-mcp
Go-MCP is a powerful Go(Golang) version of the MCP SDK that implements the Model Context Protocol (MCP) to facilitate seamless communication between external systems and AI applications.
3 years
Works with Finder
8
Github Watches
45
Github Forks
306
Github Stars
Go-MCP

🚀 Overview
Go-MCP is a powerful Go version of the MCP SDK that implements the Model Context Protocol (MCP) to facilitate seamless communication between external systems and AI applications. Based on the strong typing and performance advantages of the Go language, it provides a concise and idiomatic API to facilitate your integration of external systems into AI applications.
✨ Key Features
- 🔄 Complete Protocol Implementation: Full implementation of the MCP specification, ensuring seamless integration with all compatible services
- 🏗️ Elegant Architecture Design: Adopts a clear three-layer architecture, supports bidirectional communication, ensuring code modularity and extensibility
- 🔌 Seamless Integration with Web Frameworks: Provides MCP protocol-compliant http.Handler, allowing developers to integrate MCP into their service frameworks
- 🛡️ Type Safety: Leverages Go's strong type system for clear, highly maintainable code
- 📦 Simple Deployment: Benefits from Go's static compilation, eliminating complex dependency management
- ⚡ High-Performance Design: Fully utilizes Go's concurrency capabilities, maintaining excellent performance and low resource overhead across various scenarios
🛠️ Installation
go get github.com/ThinkInAIXYZ/go-mcp
Requires Go 1.18 or higher.
🎯 Quick Start
Client Example
package main
import (
"context"
"log"
"github.com/ThinkInAIXYZ/go-mcp/client"
"github.com/ThinkInAIXYZ/go-mcp/transport"
)
func main() {
// Create SSE transport client
transportClient, err := transport.NewSSEClientTransport("http://127.0.0.1:8080/sse")
if err != nil {
log.Fatalf("Failed to create transport client: %v", err)
}
// Initialize MCP client
mcpClient, err := client.NewClient(transportClient)
if err != nil {
log.Fatalf("Failed to create MCP client: %v", err)
}
defer mcpClient.Close()
// Get available tools
tools, err := mcpClient.ListTools(context.Background())
if err != nil {
log.Fatalf("Failed to list tools: %v", err)
}
log.Printf("Available tools: %+v", tools)
}
Server Example
package main
import (
"fmt"
"log"
"time"
"github.com/ThinkInAIXYZ/go-mcp/protocol"
"github.com/ThinkInAIXYZ/go-mcp/server"
"github.com/ThinkInAIXYZ/go-mcp/transport"
)
type TimeRequest struct {
Timezone string `json:"timezone" description:"timezone" required:"true"` // Use field tag to describe input schema
}
func main() {
// Create SSE transport server
transportServer, err := transport.NewSSEServerTransport("127.0.0.1:8080")
if err != nil {
log.Fatalf("Failed to create transport server: %v", err)
}
// Initialize MCP server
mcpServer, err := server.NewServer(transportServer)
if err != nil {
log.Fatalf("Failed to create MCP server: %v", err)
}
// Register time query tool
tool, err := protocol.NewTool("current time", "Get current time for specified timezone", TimeRequest{})
if err != nil {
log.Fatalf("Failed to create tool: %v", err)
return
}
mcpServer.RegisterTool(tool, handleTimeRequest)
// Start server
if err = mcpServer.Run(); err != nil {
log.Fatalf("Server failed to start: %v", err)
}
}
func handleTimeRequest(req *protocol.CallToolRequest) (*protocol.CallToolResult, error) {
var timeReq TimeRequest
if err := protocol.VerifyAndUnmarshal(req.RawArguments, &timeReq); err != nil {
return nil, err
}
loc, err := time.LoadLocation(timeReq.Timezone)
if err != nil {
return nil, fmt.Errorf("invalid timezone: %v", err)
}
return &protocol.CallToolResult{
Content: []protocol.Content{
protocol.TextContent{
Type: "text",
Text: time.Now().In(loc).String(),
},
},
}, nil
}
🏗️ Architecture Design
Go-MCP adopts an elegant three-layer architecture:
- Transport Layer: Handles underlying communication implementation, supporting multiple transport protocols
- Protocol Layer: Handles MCP protocol encoding/decoding and data structure definitions
- User Layer: Provides friendly client and server APIs
Currently supported transport methods:
- HTTP SSE/POST: HTTP-based server push and client requests, suitable for web scenarios
- Stdio: Standard input/output stream-based, suitable for local inter-process communication
The transport layer uses a unified interface abstraction, making it simple to add new transport methods (like Streamable HTTP, WebSocket, gRPC) without affecting upper-layer code.
🤝 Contributing
We welcome all forms of contributions! Please see CONTRIBUTING.md for details.
📄 License
This project is licensed under the MIT License - see the LICENSE file for details.
📞 Contact Us
- GitHub Issues: Submit an issue
- Discord: Click here to join our user group
- WeChat Group:
✨ Contributors
Thanks to all developers who have contributed to this project!
📈 Project Trends
相关推荐
Embark on a thrilling diplomatic quest across a galaxy on the brink of war. Navigate complex politics and alien cultures to forge peace and avert catastrophe in this immersive interstellar adventure.
Confidential guide on numerology and astrology, based of GG33 Public information
Converts Figma frames into front-end code for various mobile frameworks.
Advanced software engineer GPT that excels through nailing the basics.
MCP server to provide Figma layout information to AI coding agents like Cursor
Python code to use the MCP3008 analog to digital converter with a Raspberry Pi or BeagleBone black.
Put an end to hallucinations! GitMCP is a free, open-source, remote MCP server for any GitHub project
The all-in-one Desktop & Docker AI application with built-in RAG, AI agents, No-code agent builder, MCP compatibility, and more.
Reviews
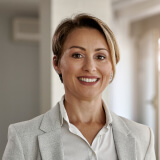
user_M7dyWlqL
I've been using go-mcp by ThinkInAIXYZ and it's fantastic! This application delivers a smooth and efficient performance consistent with MCP principles. It's easy to navigate and integrates seamlessly with my workflow. For anyone considering it, check out the official repository at https://github.com/ThinkInAIXYZ/go-mcp. Highly recommended!