I craft unique cereal names, stories, and ridiculously cute Cereal Baby images.
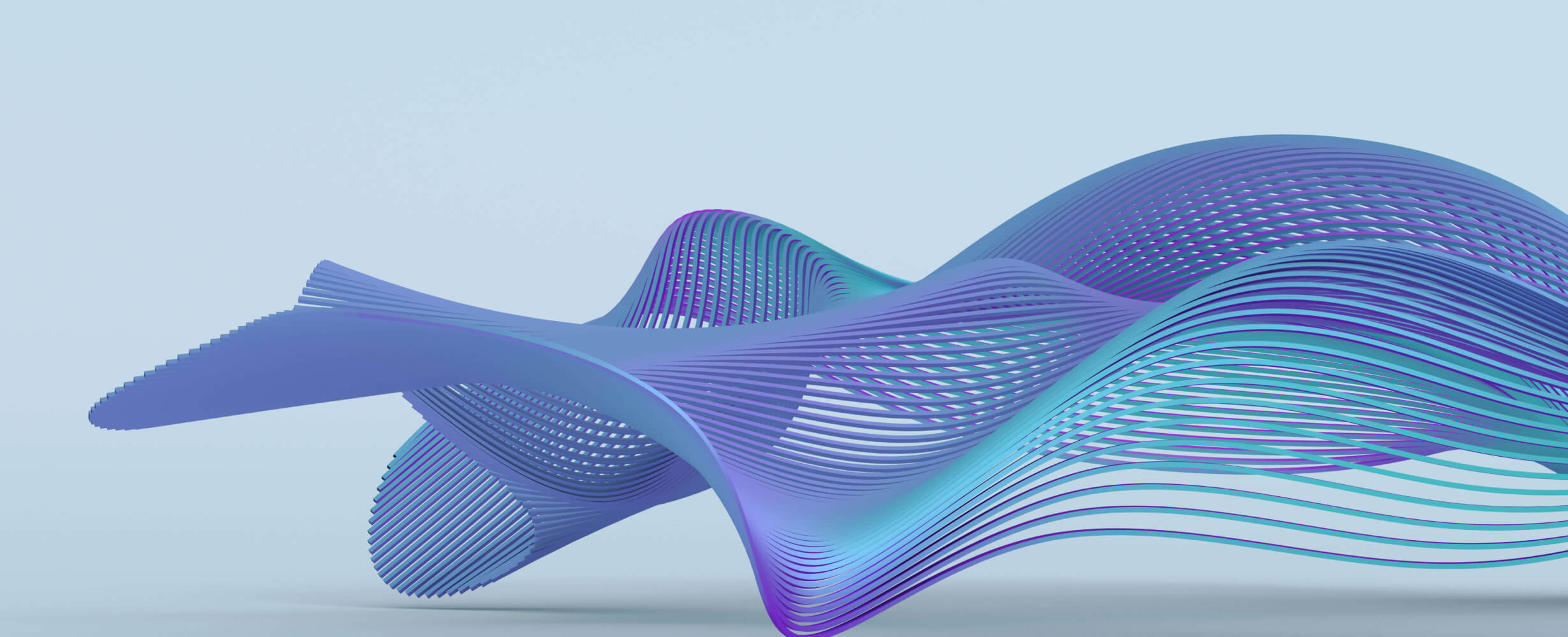
stablemcp
Simple MCP Server to generate iamges using Stable Diffusion
3 years
Works with Finder
1
Github Watches
0
Github Forks
0
Github Stars
StableMCP
A Model Context Protocol (MCP) server for generating images using Stable Diffusion.
Features
- JSON-RPC 2.0 based Model Context Protocol (MCP) implementation
- MCP-compliant API endpoint for image generation
- Integration with Stable Diffusion image generation models
- Support for various image parameters (size, style, prompt)
- API key authentication (optional)
- Configurable image size and quality settings
- Rate limiting and request validation
- Extensible capabilities system for adding new tools
Project Structure
.
├── api # API definitions, routes, and documentation
├── bin # Build artifacts
├── cmd # Application entrypoints
├── configs # Configuration files
├── examples # Example usages
├── internal # Private application code
│ ├── config # Configuration handling
│ ├── models # Data models
│ └── helpers # Helper functions
├── pkg # Public packages
│ ├── auth # Authentication/authorization
│ ├── handlers # Request handlers
│ ├── mcp # MCP protocol implementation
│ │ ├── server.go # Server implementation
│ │ └── types.go # Protocol type definitions
│ └── stablediffusion # Stable Diffusion client
└── scripts # Utility scripts
Prerequisites
- Go 1.22 or higher
- A running Stable Diffusion API (local or remote)
Getting Started
# Clone the repository
git clone https://github.com/yourusername/stablemcp.git
cd stablemcp
# Create a default configuration file
make config
# Build the server
make build
# Run with default config
make run
# Run with a custom config
make run-config
Alternatively, you can use Go commands directly:
# Build the server
go build -o bin/stablemcp ./main.go
# Run with default config
./bin/stablemcp server
# Run with a custom config
./bin/stablemcp server --config configs/.stablemcp.yaml
Configuration
StableMCP provides a flexible configuration system powered by Viper. You can configure the application through multiple sources, which are applied in the following order of precedence (highest to lowest):
- Command line flags (highest priority)
-
Environment variables with
STABLEMCP_
prefix -
Configuration file specified with
--config
flag -
Standard configuration files named
.stablemcp.yaml
or.stablemcp.json
:-
./configs/.stablemcp.yaml
(project directory) -
$HOME/.config/.stablemcp.yaml
(user home directory) -
/etc/.stablemcp.yaml
(system-wide)
-
- Default values (lowest priority)
Command Line Flags
StableMCP supports these global command line flags:
Global Flags:
-c, --config string Path to the configuration file
-d, --debug Enable debug mode (default: false)
-h, --help Help for stablemcp
-l, --log-level string Set the logging level: debug, info, warn, error (default: "info")
-o, --output string Output format: json, text (default: "json")
--timeout string Request timeout duration (default: "30s")
Server-specific flags:
Server Flags:
--host string Server host address (default: "localhost")
--port int Server port (default: 8080)
Environment Variables
All configuration options can be set using environment variables with the STABLEMCP_
prefix:
# Examples
export STABLEMCP_DEBUG=true
export STABLEMCP_LOG_LEVEL=debug
export STABLEMCP_SERVER_PORT=9000
export STABLEMCP_SERVER_HOST=0.0.0.0
Configuration File Options
You can customize the application by setting the following options in your YAML configuration file:
server:
host: "localhost" # Server host address (default: "localhost")
port: 8080 # Server port (default: 8080)
tls:
enabled: false # Enable/disable TLS (default: false)
# There are no default values for the following options, so these must be set if TLS is enabled
cert: "/path/to/cert.pem" # TLS certificate path (default: "")
key: "/path/to/key.pem" # TLS key path (default: "")
logging:
level: "info" # Log level: debug, info, warn, error (default: "info")
format: "json" # Log format: json, text (default: "json")
debug: false # Enable debug mode (default: false)
timeout: "30s" # Request timeout (default: "30s")
telemetry:
metrics:
enabled: false # Enable metrics collection (default: false)
port: 9090 # Metrics server port (default: 9090)
path: "/metrics" # Metrics endpoint path (default: "/metrics")
tracing:
enabled: false # Enable distributed tracing (default: false)
port: 9091 # Tracing server port (default: 9091)
path: "/traces" # Tracing endpoint path (default: "/traces")
# OpenAI configuration
openai:
apiKey: "your-openai-api-key" # OpenAI API key for API calls (default: "")
model: "gpt-3.5-turbo" # Model to use (default: "gpt-3.5-turbo")
baseUrl: "https://api.openai.com/v1" # Base URL for API calls
# download path for generated images
downloadPath: "~/Downloads" # Path where generated images will be saved (default: "~/Downloads")
Using a Custom Configuration File
You can specify a custom configuration file when running the server:
./bin/stablemcp server --config path/to/your/config.yaml
Or create one of these standard configuration files:
# In your project directory
touch configs/.stablemcp.yaml
# In your home directory
touch ~/.config/.stablemcp.yaml
# System-wide (requires sudo)
sudo touch /etc/.stablemcp.yaml
Configuration Precedence Examples
StableMCP applies configuration values in order of precedence. For example:
- If you set
--log-level=debug
on the command line, it will override the log level in any config file - If you set
STABLEMCP_SERVER_PORT=9000
in the environment, it will be used unless overridden by a command line flag - If you have
server.port: 8000
in your config file, it will be used unless overridden by an environment variable or command line flag
MCP Implementation
StableMCP implements the Model Context Protocol (MCP), a standard JSON-RPC 2.0 based protocol for LLM-based tools and services. The implementation consists of:
Core Components
- JSONRPCRequest/Response: Standard JSON-RPC 2.0 request and response structures
- MCPServer: Server implementation with name, version, and capabilities
- Capabilities: Extensible system for registering tools the server supports
Server Initialization
// Create a new MCP server with auto-version from the version package
server := mcp.NewMCPServer("StableMCP")
// Or create with a custom version
// server := mcp.NewMCPServerWithVersion("StableMCP", "0.1.1")
// Register capabilities/tools
server.Capabilities.Tools["stable-diffusion"] = map[string]interface{}{
"version": "1.0",
"models": []string{"sd-turbo", "sdxl"},
}
API Usage
Generate an Image
curl -X POST http://localhost:8080/v1/generate \
-H "Content-Type: application/json" \
-d '{
"prompt": "a photo of a cat in space",
"width": 512,
"height": 512,
"num_inference_steps": 50
}'
MCP Initialize Request
curl -X POST http://localhost:8080/mcp \
-H "Content-Type: application/json" \
-d '{
"jsonrpc": "2.0",
"id": "1",
"method": "initialize",
"params": {}
}'
Development
The project includes a Makefile with common development tasks:
# Run all tests
make test
# Run a specific test
make test-one TEST=TestConfigLoading
# Format code
make fmt
# Run linter
make lint
# Clean build artifacts
make clean
# Check version information
make version
# See all available commands
make help
Version Management
StableMCP follows semantic versioning and provides version information through the version
package:
import "github.com/mkm29/stablemcp/internal/version"
// Get the current version
fmt.Println("StableMCP version:", version.Version)
// Get all version info
versionInfo := version.Info()
fmt.Printf("Version: %s\nCommit: %s\nBuild Date: %s\n",
versionInfo["version"], versionInfo["gitCommit"], versionInfo["buildDate"])
Version information is embedded during build time using the following variables:
-
Version
: The current version from git tags or default (0.1.1) -
BuildDate
: The build date in ISO 8601 format -
GitCommit
: The git commit hash -
GitBranch
: The git branch name
You can check the version of a built binary with:
# JSON output (default)
./bin/stablemcp version
# Text output
./bin/stablemcp --output text version
# or
./bin/stablemcp -o text version
Alternatively, you can use Go commands directly:
# Run tests
go test ./...
# Format code
go fmt ./...
# Run linter
golangci-lint run
CI/CD with GitHub Actions
This project uses GitHub Actions for continuous integration and delivery:
Workflows
-
CI: Triggered on pushes to
main
anddevelop
branches and pull requests.- Runs linting
- Runs tests with coverage reporting
- Builds binaries for multiple platforms (Linux, macOS, Windows)
-
Release: Triggered when a new tag is pushed.
- Creates a GitHub release with binaries for all platforms
- Publishes Docker images to GitHub Container Registry
- Publishes to Homebrew tap (if configured)
-
Security Scan: Runs weekly and can be triggered manually.
- Runs
govulncheck
to check for vulnerabilities in dependencies - Runs
gosec
for Go security checks - Runs
nancy
for dependency vulnerability scanning - Runs
trivy
for comprehensive vulnerability scanning - Reports results to GitHub Security tab
- Runs
-
Issue & PR Labeler: Automatically adds labels to issues and PRs.
- Labels based on title and content
- Labels PRs based on modified files
Creating a Release
To create a new release:
# Tag a new version
git tag -a v0.1.2 -m "Release v0.1.2"
# Push the tag
git push origin v0.1.2
The release workflow will automatically build and publish the release.
License
相关推荐
Converts Figma frames into front-end code for various mobile frameworks.
I find academic articles and books for research and literature reviews.
Confidential guide on numerology and astrology, based of GG33 Public information
Embark on a thrilling diplomatic quest across a galaxy on the brink of war. Navigate complex politics and alien cultures to forge peace and avert catastrophe in this immersive interstellar adventure.
Delivers concise Python code and interprets non-English comments
Advanced software engineer GPT that excels through nailing the basics.
💬 MaxKB is an open-source AI assistant for enterprise. It seamlessly integrates RAG pipelines, supports robust workflows, and provides MCP tool-use capabilities.
Micropython I2C-based manipulation of the MCP series GPIO expander, derived from Adafruit_MCP230xx
The all-in-one Desktop & Docker AI application with built-in RAG, AI agents, No-code agent builder, MCP compatibility, and more.
MCP server to provide Figma layout information to AI coding agents like Cursor
Reviews
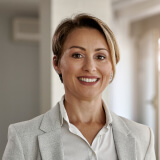
user_RA8nRHKp
StableMCP is an exceptional tool provided by mkm29 that has significantly streamlined my workflow. Its robust features and easy-to-use interface make it a standout in its category. The seamless integration and reliability of StableMCP have been incredibly beneficial. Highly recommend checking it out at https://github.com/mkm29/stablemcp for anyone looking to enhance their applications.