I craft unique cereal names, stories, and ridiculously cute Cereal Baby images.
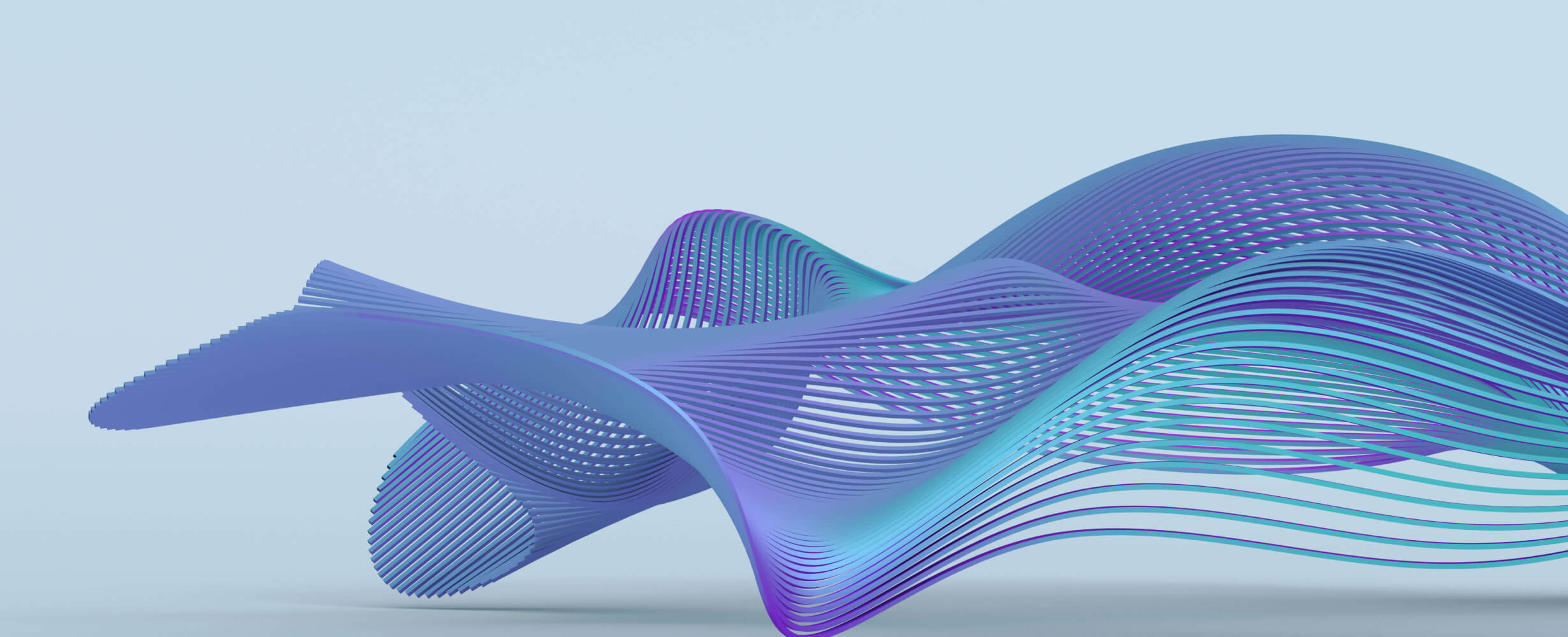
MCP Servers for Cursor IDE
This project hosts multiple Model-Context-Protocol (MCP) servers designed to work with the Cursor IDE. MCP servers allow Cursor to leverage external tools and functionalities through a standardized communication protocol.
Table of Contents
- Prerequisites
- How To Use
- What is MCP?
- Project Structure
- Available Servers
- Running the Servers
- Testing Your MCP Server
- Adding a New MCP Server
- Understanding MCP Server Development
- Building the Project
Prerequisites
- Node.js (v16 or newer)
- npm or yarn
How To Use
-
Clone this repository:
git clone https://github.com/yourusername/mcp-servers.git cd mcp-servers
-
Install dependencies and set up everything:
npm run setup
This command will:
- Install all dependencies
- Build the TypeScript project
- Generate the necessary scripts for Cursor IDE integration
- Provide instructions for setting up each server in Cursor
-
Configure Cursor IDE:
- Open Cursor IDE
- Go to Cursor Settings > Features > Mcp Servers
- Click "Add New Mcp Server"
- Enter a name for the server (e.g., "jira")
- For "Connection Type", select "command"
- For "command", paste the path provided by the prepare script
- Click "Save"
-
Environment Variables:
- Copy the
.env.example
file to.env
- Update the variables with your own credentials for each service
- Copy the
-
Use Mcp In Cursor IDE:
- Open the composer
- make sure you are using agent mode (claude 3.7 sonnet thinking is recommended)
- submit the message you want to cursor
What is MCP?
Model Context Protocol (MCP) is an open protocol that standardizes how applications provide context to LLMs (Large Language Models). Think of MCP like a communication interface between Cursor IDE and external tools. MCP servers expose tools that can be used by Cursor IDE to enhance its capabilities.
Project Structure
mcp-servers/
├── src/
│ ├── servers/ # Individual MCP servers
│ │ ├── addition-server/ # Example addition server
│ │ │ └── addition-server.ts
│ │ └── ... (more servers)
│ ├── template/ # Reusable templates
│ │ └── mcp-server-template.ts
│ ├── index.ts # Server runner utility
│ └── run-all.ts # Script to run all servers
├── package.json
└── tsconfig.json
Available Servers
Currently, the following MCP servers are available:
- Addition Server - A simple server that adds two numbers together.
- Jira Server - A server that provides access to Jira's REST API for retrieving projects, issues, boards, and sprints.
- GitHub Server - A server that provides access to GitHub's REST API for retrieving repositories, issues, pull requests, branches, and commits.
- PostgreSQL Server - A server that provides access to a PostgreSQL database for executing queries and retrieving database schema information.
- Kubernetes Server - A server that provides access to a Kubernetes cluster for managing pods, executing commands, and retrieving logs.
Jira Server Setup
The Jira server requires the following environment variables:
-
Create a
.env
file in the project root (or copy from.env.example
):# Jira API Configuration JIRA_API_URL=https://your-domain.atlassian.net JIRA_EMAIL=your-email@example.com JIRA_API_TOKEN=your-jira-api-token
-
Generate an API token at: https://id.atlassian.com/manage-profile/security/api-tokens
Available Jira Tools
The Jira server exposes the following tools:
-
get_projects
- Retrieves all accessible Jira projects -
get_project
- Gets details about a specific project by key -
search_issues
- Searches for issues using JQL (Jira Query Language) -
get_issue
- Gets details about a specific issue by key -
get_boards
- Retrieves all accessible boards -
get_sprints
- Gets all sprints for a specific board
GitHub Server Setup
The GitHub server requires the following environment variables:
-
Create a
.env
file in the project root (or copy from.env.example
):# GitHub API Configuration GITHUB_TOKEN=your-github-personal-access-token
-
Generate a personal access token at: https://github.com/settings/tokens
Available GitHub Tools
The GitHub server exposes the following tools:
-
get_repositories
- Retrieves all repositories accessible to the authenticated user -
get_repository
- Gets details about a specific repository -
get_issues
- Retrieves issues for a repository -
get_issue
- Gets details about a specific issue -
get_pull_requests
- Retrieves pull requests for a repository -
get_pull_request
- Gets details about a specific pull request -
get_branches
- Retrieves branches for a repository -
get_commits
- Gets commits for a repository, optionally filtered by branch -
comment_on_pr
- Adds a comment to a specific pull request (always signed with "Created by Cursor") -
summarize_pr_diff
- Generates a summary of PR changes and optionally posts it as a comment
PostgreSQL Server Setup
The PostgreSQL server requires the following environment variables:
-
Create a
.env
file in the project root (or copy from.env.example
):# PostgreSQL Configuration POSTGRES_HOST=localhost POSTGRES_PORT=5432 POSTGRES_DB=your_database_name POSTGRES_USER=your_username POSTGRES_PASSWORD=your_password # POSTGRES_SSL_MODE=require # Uncomment if SSL is required # POSTGRES_MAX_CONNECTIONS=10 # Optional: limit connection pool size
-
Ensure you have a PostgreSQL database running and accessible with the provided credentials.
Available PostgreSQL Tools
The PostgreSQL server exposes the following tools:
-
mcp__get_database_info
- Retrieves information about the connected database -
mcp__list_tables
- Lists all tables in the current database schema -
mcp__get_table_structure
- Gets the column definitions for a specific table -
mcp__execute_query
- Executes a custom SQL query against the database
Kubernetes Server Setup
The Kubernetes server requires the following environment variables:
-
Create a
.env
file in the project root (or copy from.env.example
):# Kubernetes Configuration KUBECONFIG=/path/to/your/kubeconfig # or # KUBE_API_URL=https://your-kubernetes-api-server # KUBE_API_TOKEN=your-kubernetes-service-account-token
-
Ensure you have access to a Kubernetes cluster and the appropriate permissions.
Available Kubernetes Tools
The Kubernetes server exposes the following tools:
-
get_pods
- Retrieves pods from a specified namespace, with optional field and label selectors -
find_pods
- Finds pods matching a name pattern in a specified namespace -
kill_pod
- Deletes a pod in a specified namespace -
exec_in_pod
- Executes a command in a specified pod and container -
get_pod_logs
- Retrieves logs from a specified pod, with options for container, line count, and previous instance
Running the Servers
Quick Start
The setup command creates individual shell scripts for each server that can be used directly with Cursor IDE.
After running npm run setup
, you'll see instructions for each server configuration.
Running a Server Using the Helper Script
To run a specific server using the included helper script:
npm run server -- [server-name]
For example, to run the addition server:
npm run server -- addition
This will automatically build the TypeScript code and start the server.
Running a Single Server Manually
To run a specific server manually:
npm run dev -- [server-name]
For example, to run the addition server:
npm run dev -- addition
Running All Servers
To run all servers simultaneously:
npm run dev:all
List Available Servers
To see a list of all available servers:
npm run dev -- --list
Testing Your MCP Server
Before connecting to Cursor IDE, you can test your MCP server's functionality:
-
Build your TypeScript project:
npm run build
-
Run the server:
npm run start:addition
-
In a separate terminal, you can test the server by sending MCP protocol messages manually:
{ "type": "request", "id": "test1", "name": "add", "params": { "num1": 5, "num2": 7 } }
The server should respond with:
{ "type": "response", "id": "test1", "content": [ { "type": "text", "text": "The sum of 5 and 7 is 12." }, { "type": "text", "text": "{\n \"num1\": 5,\n \"num2\": 7,\n \"sum\": 12\n}" } ] }
-
For convenience, this project includes a ready-to-use script for Cursor:
/path/to/mcp-servers/cursor-mcp-server.sh
You can use this script path directly in your Cursor IDE configuration.
Adding a New MCP Server
To add a new MCP server to this project:
-
Create a new directory for your server under
src/servers/
:mkdir -p src/servers/my-new-server
-
Create your server implementation:
// src/servers/my-new-server/my-new-server.ts import { McpServer } from "@modelcontextprotocol/sdk/server/mcp.js"; import { StdioServerTransport } from "@modelcontextprotocol/sdk/server/stdio.js"; import { z } from "zod"; // Create an MCP server const server = new McpServer({ name: "My New Server", version: "1.0.0", }); // Add your tools server.tool( "my-tool", { param1: z.string().describe("Parameter description"), param2: z.number().describe("Parameter description"), }, async ({ param1, param2 }) => { // Tool implementation return { content: [{ type: "text", text: `Result: ${param1} ${param2}` }], }; } ); // Start the server async function startServer() { const transport = new StdioServerTransport(); await server.connect(transport); console.log("My New Server started and ready to process requests"); } // Start the server if this file is run directly if (process.argv[1] === new URL(import.meta.url).pathname) { startServer(); } export default server;
-
Add your server to the server list in
src/index.ts
andsrc/run-all.ts
:const servers = [ // ... existing servers { name: "my-new-server", displayName: "My New Server", path: join(__dirname, "servers/my-new-server/my-new-server.ts"), }, ];
-
Update the package.json scripts (optional):
"scripts": { // ... existing scripts "dev:my-new-server": "ts-node --esm src/servers/my-new-server/my-new-server.ts" }
Understanding MCP Server Development
When developing an MCP server, keep in mind:
-
Tools are the primary way to expose functionality. Each tool should:
- Have a unique name
- Define parameters using Zod for validation
- Return results in a standardized format
-
Communication happens via stdio for Cursor integration.
-
Response Format is critical - MCP servers must follow the exact format:
- Tools should return content with
type: "text"
for text responses - Avoid using unsupported types like
type: "json"
directly - For structured data, convert to JSON string and use
type: "text"
- Example:
return { content: [ { type: "text", text: "Human-readable response" }, { type: "text", text: JSON.stringify(structuredData, null, 2) }, ], };
- Tools should return content with
Building the Project
To build the project, run:
npm run build
相关推荐
Evaluator for marketplace product descriptions, checks for relevancy and keyword stuffing.
I find academic articles and books for research and literature reviews.
Confidential guide on numerology and astrology, based of GG33 Public information
Converts Figma frames into front-end code for various mobile frameworks.
Advanced software engineer GPT that excels through nailing the basics.
Take an adjectivised noun, and create images making it progressively more adjective!
Discover the most comprehensive and up-to-date collection of MCP servers in the market. This repository serves as a centralized hub, offering an extensive catalog of open-source and proprietary MCP servers, complete with features, documentation links, and contributors.
Micropython I2C-based manipulation of the MCP series GPIO expander, derived from Adafruit_MCP230xx
Mirror ofhttps://github.com/agentience/practices_mcp_server
The all-in-one Desktop & Docker AI application with built-in RAG, AI agents, No-code agent builder, MCP compatibility, and more.
A unified API gateway for integrating multiple etherscan-like blockchain explorer APIs with Model Context Protocol (MCP) support for AI assistants.
Reviews
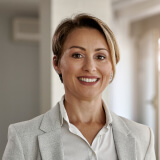
user_ijzyZ5cK
As a dedicated user of the PHP Universal MCP Server by Lucasdoreac, I must say this product is outstanding. It has significantly streamlined my server management tasks. The setup was straightforward, and the server's performance has been consistently reliable. If you're looking for a robust MCP server solution, this is definitely worth considering. Highly recommended!