I craft unique cereal names, stories, and ridiculously cute Cereal Baby images.
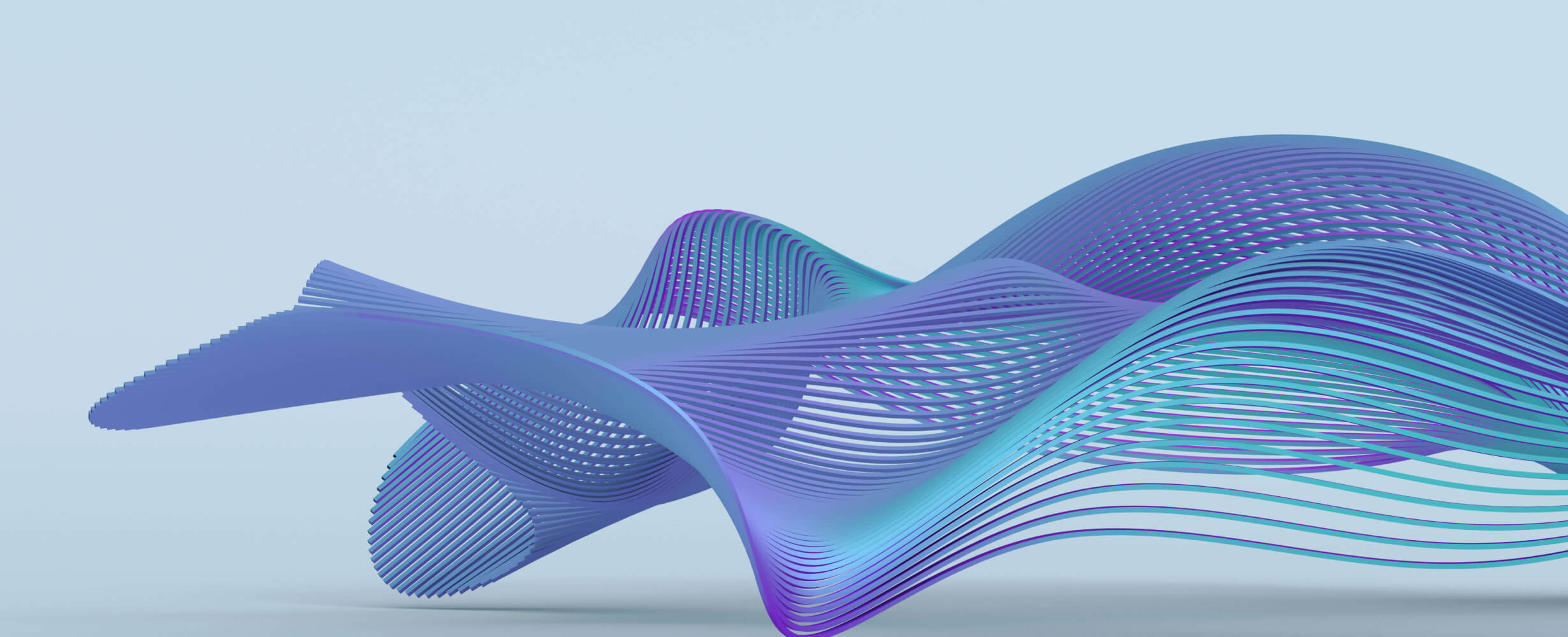
mcp-lambda-sam
An AWS Serverless Application Model that operates as an MCP server via serverless AWS resources
3
Github Watches
2
Github Forks
9
Github Stars
MCP Lambda SAM
Model Context Protocol (MCP) implementation using AWS Lambda and SAM.
Overview
This project provides a serverless implementation of the Model Context Protocol, with two distinct interfaces:
-
System Configuration (Administrative):
- Registration of MCP tools, resources, and prompts
- IAM permission management
- Infrastructure setup and configuration
-
System Usage (Client):
- Establishing SSE connections
- Sending commands
- Receiving streaming responses
Architecture
Mermaid Diagram
You can visualize the system using this Mermaid syntax:
graph TD
Client --> MCP[/"MCP Lambda\n(/sse & /message)"/]
MCP -->|read/write| SessionTable[(Session Table)]
MCP -->|query| RegistrationTable[(Registration Table)]
MCP -->|invoke| RegisteredLambda["Registered Lambda Tool"]
Admin[Administrator] --> RegistrationLambda[/"Registration Lambda\n(/register)"/]
RegistrationLambda -->|write| RegistrationTable
- The MCP Lambda reads registrations from the Registration Table on startup and when handling requests.
- It uses the Session Table to persist session state.
- It invokes registered Lambda tools dynamically using the ARNs stored in the Registration Table.
System Configuration Guide (Administrators)
This section is for system administrators who need to configure and manage the MCP server.
Deployment
npx @markvp/mcp-lambda-sam deploy
The command will interactively prompt for administrative configuration:
- Stack name (for multiple instances)
- AWS Region
- VPC configuration (optional)
Permissions Overview
To access MCP endpoints, users and clients must have IAM permission to invoke the relevant Function URLs.
-
Administrators: must be allowed to invoke the
mcp-registration
function URL -
Clients: must be allowed to invoke the
mcp
function URL
You can grant access using either an IAM policy or aws lambda add-permission
(see below).
Assigning Permissions via AWS CLI
To grant permission to invoke the registration function URL:
aws lambda add-permission \
--function-name <registration-function-name> \
--statement-id allow-registration \
--action lambda:InvokeFunctionUrl \
--principal "*" \
--function-url-auth-type IAM
To grant permission to invoke the MCP function URL (SSE and message):
aws lambda add-permission \
--function-name <mcp-function-name> \
--statement-id allow-mcp \
--action lambda:InvokeFunctionUrl \
--principal "*" \
--function-url-auth-type IAM
Replace <registration-function-name>
and <mcp-function-name>
with the actual Lambda function names.
Registration API
Use these endpoints to manage MCP tools, resources, and prompts:
Register a New Tool
awscurl -X POST ${REGISTRATION_URL}/register \
--region ap-southeast-2 \
--service lambda \
-H "Content-Type: application/json" \
-d '{
"type": "tool",
"name": "example",
"description": "Example tool",
"lambdaArn": "arn:aws:lambda:region:account:function:name",
"parameters": {
"input": "string"
}
}'
Update Registration
awscurl -X PUT ${REGISTRATION_URL}/register/{id} \
--region ap-southeast-2 \
--service lambda \
-d '...'
Delete Registration
awscurl -X DELETE ${REGISTRATION_URL}/register/{id} \
--region ap-southeast-2 \
--service lambda
List Registrations
awscurl ${REGISTRATION_URL}/register \
--region ap-southeast-2 \
--service lambda
Required IAM Permissions
For Administrators
Administrators need these permissions to manage registrations:
{
"Version": "2012-10-17",
"Statement": [{
"Effect": "Allow",
"Action": "lambda:InvokeFunctionUrl",
"Resource": "arn:aws:lambda:${region}:${account}:function:${stack-id}-mcp-registration",
"Condition": {
"StringEquals": {
"lambda:FunctionUrlAuthType": "AWS_IAM"
}
}
}]
}
System Usage Guide (Clients)
This section is for clients who want to use the MCP server.
Required IAM Permissions
Clients need these permissions to use the MCP server:
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": "lambda:InvokeFunctionUrl",
"Resource": [
"arn:aws:lambda:${region}:${account}:function:${stack-id}-mcp",
],
"Condition": {
"StringEquals": {
"lambda:FunctionUrlAuthType": "AWS_IAM"
}
}
}
]
}
Connecting to the Server
- Establish SSE Connection:
const sse = new EventSource(SSE_URL, {
headers: {
Authorization: 'AWS4-HMAC-SHA256 ...', // Must be AWS SigV4 signed
}
});
sse.onmessage = (event) => {
console.log(JSON.parse(event.data));
};
Example cURL for SSE
awscurl -X GET "${MCP_URL}/sse" \
--region ap-southeast-2 \
--service lambda
The first event will include a sessionId
. Use this when sending messages.
- Send Commands:
awscurl -X POST "${MCP_URL}/message?sessionId=session-123" \
--region ap-southeast-2 \
--service lambda \
-H "Content-Type: application/json" \
-d '{
"jsonrpc": "2.0",
"id": "1",
"method": "example",
"params": {
"input": "hello"
}
}'
Error Handling
Common Client Errors
-
401
: Invalid/missing AWS credentials -
403
: Insufficient permissions -
404
: Invalid session ID -
429
: Rate limit exceeded
Troubleshooting
-
Connection Issues:
- Verify AWS credentials
- Check IAM permissions
- Ensure network connectivity
-
Command Execution Errors:
- Verify session ID is active
- Check command format matches tool registration
- Ensure parameters match schema
Requirements
- AWS CLI installed and configured
- AWS SAM CLI installed
- Node.js 20.x or later
- An AWS account with permissions to create:
- Lambda functions
- DynamoDB tables
- IAM roles
- SQS queues
AWS SAM CLI Setup
To deploy this application locally or to AWS using the AWS SAM CLI:
- Install the AWS SAM CLI: https://docs.aws.amazon.com/serverless-application-model/latest/developerguide/install-sam-cli.html
- Ensure it's available in your PATH:
sam --version
- Build and deploy the application:
sam build sam deploy --guided
- Follow the prompts to configure the stack name, region, capabilities, and parameter overrides.
You can rerun sam deploy
without --guided
to use saved configuration.
Installation
You can install and deploy this application in four ways:
1. Using AWS Serverless Application Repository (SAR)
The easiest way to deploy the MCP server is through the AWS Serverless Application Repository (SAR):
- Go to the SAR Console
- Search for mcp-lambda-sam by Mark Van Proctor
- Click Deploy
- Set your parameters:
-
StackIdentifier
: Unique ID for this MCP server instance -
VpcEnabled
: Set totrue
if deploying in a VPC -
VpcId
andSubnetIds
: Provide only ifVpcEnabled
istrue
-
- Follow the prompts to deploy
Alternatively, you can deploy from the AWS CLI:
aws serverlessrepo create-cloud-formation-change-set \
--application-id arn:aws:serverlessrepo:ap-southeast-2:522814717816:applications/mcp-lambda-sam \
--stack-name your-stack-name \
--capabilities CAPABILITY_IAM \
--parameter-overrides '[{"name":"StackIdentifier","value":"your-stack-id"}]'
2. Using npx (CLI)
npx @markvp/mcp-lambda-sam deploy
The command will interactively prompt for administrative configuration:
- Stack name (for multiple instances)
- AWS Region
- VPC configuration (optional)
3. Programmatic Usage with Install
Install the package:
npm install @markvp/mcp-lambda-sam
After installing the package, you can use it programmatically:
import { deploy } from '@markvp/mcp-lambda-sam';
// Usage example
deploy();
4. Local Development and Deployment
Install the package:
npm install @markvp/mcp-lambda-sam
After making development changes, you can deploy it manually:
npm run deploy
Development
# Install dependencies
npm install
# Lint
npm run lint
# Run tests
npm test
# Build
npm run build
# Deploy
npm run deploy
Publishing to SAR
If you're contributing to this project and need to publish updates to SAR:
- Package the application:
npm run package:sar
- Publish to SAR:
npm run publish:sar
- Make the application public (one-time setup):
- Go to AWS Console > Serverless Application Repository
- Select the application
- Click "Share" and choose "Public"
- Apply the following sharing policy:
{ "Version": "2012-10-17", "Statement": [ { "Effect": "Allow", "Principal": "*", "Action": "serverlessrepo:CreateCloudFormationTemplate", "Resource": "arn:aws:serverlessrepo:${region}:${account-id}:applications/mcp-lambda-sam" } ] }
License
MIT
Contributing
- Fork the repository
- Create your feature branch
- Commit your changes
- Push to the branch
- Create a new Pull Request
相关推荐
Evaluator for marketplace product descriptions, checks for relevancy and keyword stuffing.
I find academic articles and books for research and literature reviews.
Confidential guide on numerology and astrology, based of GG33 Public information
Converts Figma frames into front-end code for various mobile frameworks.
Advanced software engineer GPT that excels through nailing the basics.
Take an adjectivised noun, and create images making it progressively more adjective!
Discover the most comprehensive and up-to-date collection of MCP servers in the market. This repository serves as a centralized hub, offering an extensive catalog of open-source and proprietary MCP servers, complete with features, documentation links, and contributors.
Micropython I2C-based manipulation of the MCP series GPIO expander, derived from Adafruit_MCP230xx
Mirror ofhttps://github.com/agentience/practices_mcp_server
A unified API gateway for integrating multiple etherscan-like blockchain explorer APIs with Model Context Protocol (MCP) support for AI assistants.
The all-in-one Desktop & Docker AI application with built-in RAG, AI agents, No-code agent builder, MCP compatibility, and more.
Reviews
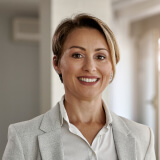
user_afFyLBL7
As a dedicated user of MCP applications, I found the mcp-lambda-sam incredibly efficient for managing AWS Lambda. Its functionality is well-structured and easy to deploy, streamlining development processes. Kudos to markvp for creating such a powerful tool, making it an essential resource for any developer working with AWS Lambda. Highly recommend checking it out at https://github.com/markvp/mcp-lambda-layer.