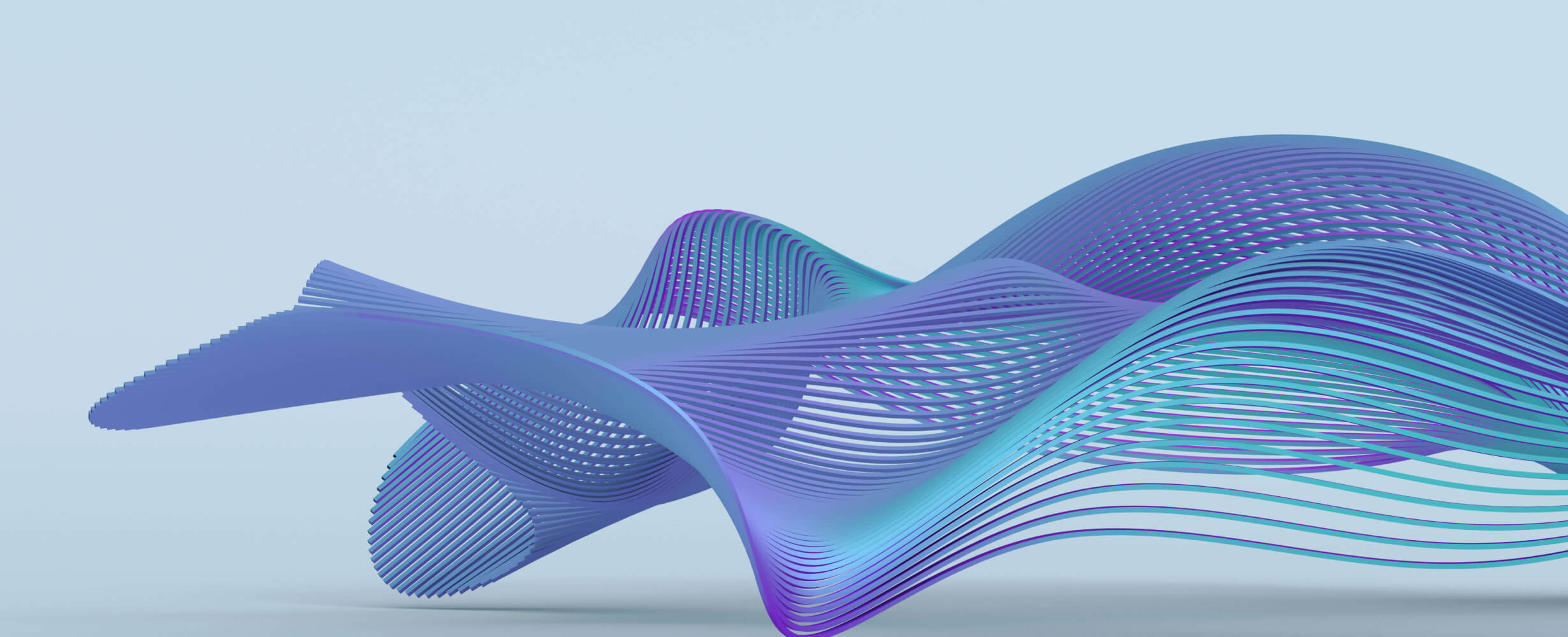
Google_chat_mcp_server
This is G_chat Mcp server written in Ts contain various tools fetch to post.
1
Github Watches
0
Github Forks
0
Github Stars
Google Workspace MCP Server
A Model Context Protocol (MCP) server that provides tools for interacting with Gmail and Calendar APIs. This server enables you to manage your emails and calendar events programmatically through the MCP interface.
Features
Gmail Tools
-
list_emails
: List recent emails from your inbox with optional filtering -
search_emails
: Advanced email search with Gmail query syntax -
send_email
: Send new emails with support for CC and BCC -
modify_email
: Modify email labels (archive, trash, mark read/unread)
Calendar Tools
-
list_events
: List upcoming calendar events with date range filtering -
create_event
: Create new calendar events with attendees -
update_event
: Update existing calendar events -
delete_event
: Delete calendar events
Prerequisites
- Node.js: Install Node.js version 14 or higher
-
Google Cloud Console Setup:
- Go to Google Cloud Console
- Create a new project or select an existing one
- Enable the Gmail API and Google Calendar API:
- Go to "APIs & Services" > "Library"
- Search for and enable "Gmail API"
- Search for and enable "Google Calendar API"
- Set up OAuth 2.0 credentials:
- Go to "APIs & Services" > "Credentials"
- Click "Create Credentials" > "OAuth client ID"
- Choose "Web application"
- Set "Authorized redirect URIs" to include:
http://localhost:3000/auth/callback
- Note down the Client ID and Client Secret
Installing via Smithery
Install the server using Smithery's CLI:
npx spinai-mcp install @KaranThink41/gsuite-mcp-server --provider smithery --config '{"googleClientId":"your_google_client_id","googleClientSecret":"your_google_client_secret","googleRefreshToken":"your_google_refresh_token"}'
Alternatively, you can use the following configuration:
// smithery.config.js
export default {
KaranThink41_google_chat_mcp_server: {
command: 'npx',
args: [
'-y',
'@smithery/cli@latest',
'run',
'@KaranThink41/google_chat_mcp_server'
]
},
};
Local Development (Optional)
If you prefer to run the server locally:
- Clone the repository:
git clone https://github.com/KaranThink41/Google_workspace_mcp_server.git
cd Google_workspace_mcp_server
- Install dependencies:
npm install
- Build the project:
npm run build
- Run the server:
node build/index.js
Usage Examples
Gmail Operations
-
List Recent Emails:
{ "maxResults": 5, "query": "is:unread" }
-
Search Emails:
{ "query": "from:example@gmail.com has:attachment", "maxResults": 10 }
-
Send Email:
{ "to": "recipient@example.com", "subject": "Hello", "body": "Message content", "cc": "cc@example.com", "bcc": "bcc@example.com" }
-
Modify Email:
{ "id": "message_id", "addLabels": ["UNREAD"], "removeLabels": ["INBOX"] }
Calendar Operations
-
List Events:
{ "maxResults": 10, "timeMin": "2024-01-01T00:00:00Z", "timeMax": "2024-12-31T23:59:59Z" }
-
Create Event:
{ "summary": "Team Meeting", "location": "Conference Room", "description": "Weekly sync-up", "start": "2024-01-24T10:00:00Z", "end": "2024-01-24T11:00:00Z", "attendees": ["colleague@example.com"] }
-
Update Event:
{ "eventId": "event_id", "summary": "Updated Meeting Title", "location": "Virtual", "start": "2024-01-24T11:00:00Z", "end": "2024-01-24T12:00:00Z" }
-
Delete Event:
{ "eventId": "event_id" }
Troubleshooting
-
Authentication Issues:
- Ensure all required OAuth scopes are granted
- Verify client ID and secret are correct
- Check if refresh token is valid
-
API Errors:
- Check Google Cloud Console for API quotas and limits
- Ensure APIs are enabled for your project
- Verify request parameters match the required format
License
This project is licensed under the MIT License.
Google Chat MCP Server
A Model Context Protocol (MCP) server implementation for interacting with Google Chat API. This server provides tools for managing messages, spaces, and memberships in Google Chat.
Features
- Post text messages to Google Chat spaces
- Get space details and list spaces
- Manage memberships (list, get member details)
- List and retrieve messages with filtering capabilities
- Natural language filtering support
Setup Instructions
1. Google Cloud Project Setup
- Go to Google Cloud Console
- Create a new project or select an existing one
- Enable the Google Chat API
- Create OAuth 2.0 credentials:
- Go to API & Services > Credentials
- Click "Create Credentials" > OAuth client ID
- Choose "Web application"
- Set "Authorized redirect URIs" to include:
http://localhost:3000/auth/callback
- Note down the Client ID and Client Secret
2. Get Refresh Token
- Create a temporary credentials file:
cp temp-credentials.json.example temp-credentials.json
-
Update the
temp-credentials.json
with your Client ID and Client Secret -
Run the refresh token script:
node get-refresh-token.js
- Follow the browser authentication flow to get the refresh token
3. Install via Smithery
Install the server using Smithery's CLI:
npx spinai-mcp install @KaranThink41/google_chat_mcp_server --provider smithery --config '{"spaceId":"your_space_id","googleClientId":"your_google_client_id","googleClientSecret":"your_google_client_secret","googleRefreshToken":"your_google_refresh_token"}'
Alternatively, you can use the following configuration:
export default {
KaranThink41_google_chat_mcp_server: {
command: 'npx',
args: [
'-y',
'@smithery/cli@latest',
'run',
'@KaranThink41/google_chat_mcp_server'
]
},
};
4. Local Development (Optional)
If you prefer to run the server locally:
- Clone the repository:
git clone https://github.com/KaranThink41/Google_chat_mcp_server.git
cd Google_chat_mcp_server
- Install dependencies:
npm install
- Build the project:
npm run build
- Run the server:
node build/index.js
Available Tools
Message Management
-
post_text_message
: Post a text message to a Google Chat space{ "method": "tools/call", "params": { "name": "post_text_message", "arguments": { "spaceId": "AAAAfkdUqxE", "text": "Hello, this is a test message!" } } }
-
fetch_message_details
: Get detailed information about a specific message{ "method": "tools/call", "params": { "name": "fetch_message_details", "arguments": { "spaceId": "AAAAfkdUqxE", "messageId": "cpwLU-2f_z8.cpwLU-2f_z8" } } }
-
list_space_messages
: List messages in a space with optional filtering{ "method": "tools/call", "params": { "name": "list_space_messages", "arguments": { "spaceId": "AAAAfkdUqxE", "pageSize": 10, "orderBy": "createTime" } } }
Space Management
-
fetch_space_details
: Get comprehensive details about a space{ "method": "tools/call", "params": { "name": "fetch_space_details", "arguments": { "spaceId": "AAAAfkdUqxE" } } }
-
list_joined_spaces
: List all spaces the caller is a member of{ "method": "tools/call", "params": { "name": "list_joined_spaces", "arguments": { "pageSize": 10 } } }
Membership Management
-
list_space_memberships
: List all memberships in a space{ "method": "tools/call", "params": { "name": "list_space_memberships", "arguments": { "spaceId": "AAAAfkdUqxE", "pageSize": 10 } } }
-
fetch_member_details
: Get detailed information about a specific member{ "method": "tools/call", "params": { "name": "fetch_member_details", "arguments": { "spaceId": "AAAAfkdUqxE", "memberId": "user_123" } } }
Natural Language Filtering
-
apply_natural_language_filter
: Convert natural language queries into API filter strings{ "method": "tools/call", "params": { "name": "apply_natural_language_filter", "arguments": { "filterText": "messages from Monday" } } }
Error Handling
The server implements proper error handling with descriptive error messages. Common errors include:
- Authentication failures
- Invalid space IDs
- Rate limiting
- API quota exceeded
Security
- All sensitive credentials are stored in environment variables
- OAuth refresh tokens are securely managed
- API keys are never exposed in the code
Contributing
- Fork the repository
- Create your feature branch (
git checkout -b feature/amazing-feature
) - Commit your changes (
git commit -m 'Add some amazing feature'
) - Push to the branch (
git push origin feature/amazing-feature
) - Open a Pull Request
License
This project is licensed under the MIT License - see the LICENSE file for details.
相关推荐
I find academic articles and books for research and literature reviews.
Confidential guide on numerology and astrology, based of GG33 Public information
Converts Figma frames into front-end code for various mobile frameworks.
Advanced software engineer GPT that excels through nailing the basics.
Take an adjectivised noun, and create images making it progressively more adjective!
Emulating Dr. Jordan B. Peterson's style in providing life advice and insights.
Embark on a thrilling diplomatic quest across a galaxy on the brink of war. Navigate complex politics and alien cultures to forge peace and avert catastrophe in this immersive interstellar adventure.
Discover the most comprehensive and up-to-date collection of MCP servers in the market. This repository serves as a centralized hub, offering an extensive catalog of open-source and proprietary MCP servers, complete with features, documentation links, and contributors.
Micropython I2C-based manipulation of the MCP series GPIO expander, derived from Adafruit_MCP230xx
Mirror ofhttps://github.com/agentience/practices_mcp_server
The all-in-one Desktop & Docker AI application with built-in RAG, AI agents, No-code agent builder, MCP compatibility, and more.
Mirror ofhttps://github.com/bitrefill/bitrefill-mcp-server
Reviews
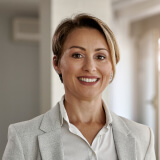
user_qSYeZmCp
I've been using the Google_chat_mcp_server by KaranThink41, and it's simply fantastic! The setup was straightforward, and the server's performance is top-notch, making it perfect for managing my chat applications. The support and documentation provided are also excellent. Highly recommend it to anyone looking for a reliable MCP server solution!