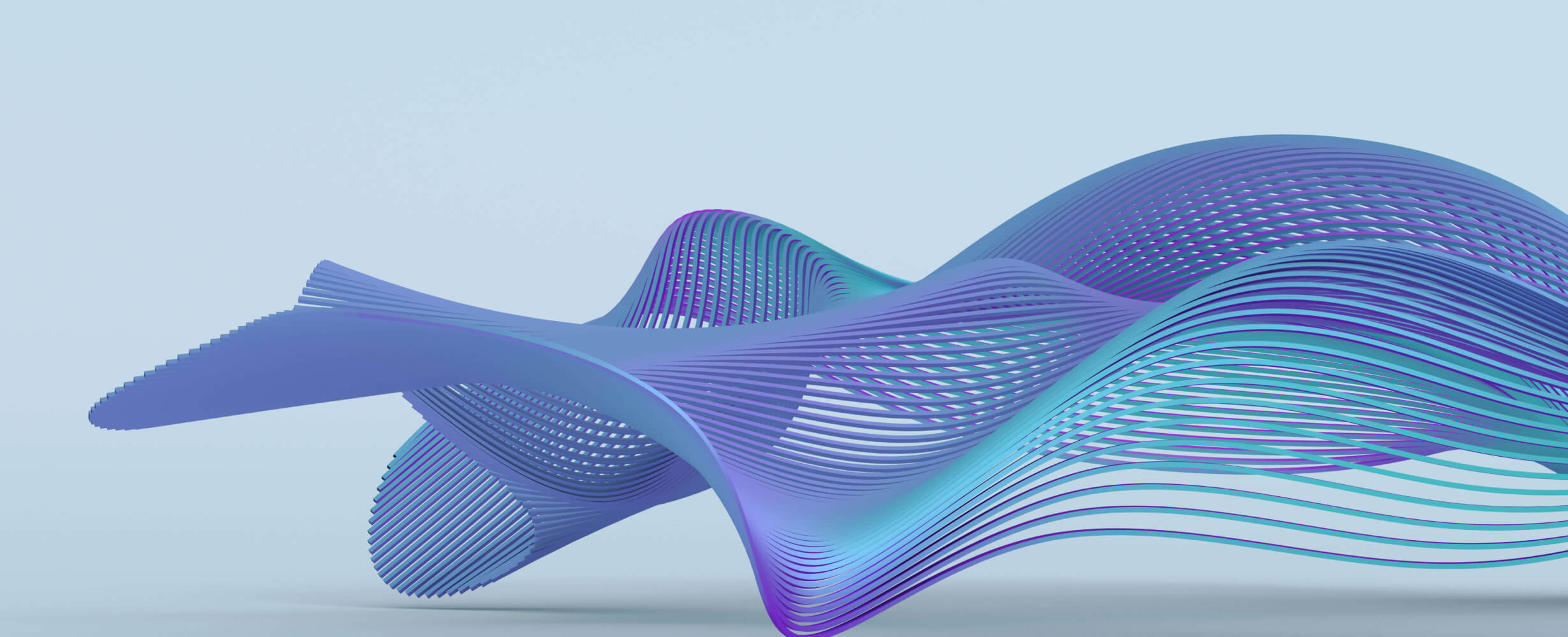
node-mcp23017
Node.js library for the I2C I/O Expander MCP23017 on a Raspberry Pi
3
Github Watches
28
Github Forks
19
Github Stars
node-mcp23017
Node.js library for the I2C I/O Expander MCP23017 on a Raspberry Pi
It currently supports reading, writing and changing the pull-up resistor of the GPIOs.
The module tries to mimic the Arduino-Syntax
##Installation
install via npm. just type the following in the terminal/console
npm install node-mcp23017 --save
Raspberry Pi Setup
In order to use this module with the Raspberry Pi running Raspbian you have to enable to stuff
$ sudo vi /etc/modules
Add these two lines
i2c-bcm2708
i2c-dev
$ sudo vi /etc/modprobe.d/raspi-blacklist.conf
Comment out blacklist i2c-bcm2708
#blacklist i2c-bcm2708
Load kernel module
$ sudo modprobe i2c-bcm2708
Make device writable
sudo chmod o+rw /dev/i2c*
Usage
NOTE
Pins are numbered from 0-15 where 0-7 is register A and 8-15 is register B
var MCP23017 = require('node-mcp23017');
var mcp = new MCP23017({
address: 0x20,//default: 0x20
device: 1, // 1 for '/dev/i2c-1' on model B | 0 for '/dev/i2c-0' on model A
// 'ls /dev/i2c*' to find which device file / number you should use
debug: true //default: false
});
/*
By default all GPIOs are defined as INPUTS.
You can set them all the be OUTPUTs by using the pinMode-Methode (see below),
You can also disable the debug option by simply not passing it to the constructor
or by setting it to false
*/
//set all GPIOS to be OUTPUTS
for (var i = 0; i < 16; i++) {
mcp.pinMode(i, mcp.OUTPUT);
//mcp.pinMode(i, mcp.INPUT); //if you want them to be inputs
//mcp.pinMode(i, mcp.INPUT_PULLUP); //if you want them to be pullup inputs
}
mcp.digitalWrite(0, mcp.HIGH); //set GPIO A Pin 0 to state HIGH
mcp.digitalWrite(0, mcp.LOW); //set GPIO A Pin 0 to state LOW
/*
to read an input use the following code-block.
This reads pin Nr. 0 (GPIO A Pin 0)
value is either false or true
*/
mcp.digitalRead(0, function (pin, err, value) {
console.log('Pin 0', value);
});
Example (Blink 16 LEDs)
see examples folder
var MCP23017 = require('node-mcp23017');
var mcp = new MCP23017({
address: 0x20, //all address pins pulled low
device: 1, // Model B
debug: false
});
/*
This function blinks 16 LED, each hooked up to an port of the MCP23017
*/
var pin = 0;
var max = 16;
var state = false;
var blink = function() {
if (pin >= max) {
pin = 0; //reset the pin counter if we reach the end
}
if (state) {
mcp.digitalWrite(pin, mcp.LOW); //turn off the current LED
pin++; //increase counter
} else {
mcp.digitalWrite(pin, mcp.HIGH); //turn on the current LED
console.log('blinking pin', pin);
}
state = !state; //invert the state of this LED
};
//define all gpios as outputs
for (var i = 0; i < 16; i++) {
mcp.pinMode(i, mcp.OUTPUT);
}
setInterval(blink, 100); //blink all LED's with a delay of 100ms
Acknowledgement
some parts are derived from the module https://github.com/x3itsolutions/mcp23017 by x3itsolutions (Fabian Behnke)
相关推荐
Confidential guide on numerology and astrology, based of GG33 Public information
Converts Figma frames into front-end code for various mobile frameworks.
Advanced software engineer GPT that excels through nailing the basics.
Take an adjectivised noun, and create images making it progressively more adjective!
Embark on a thrilling diplomatic quest across a galaxy on the brink of war. Navigate complex politics and alien cultures to forge peace and avert catastrophe in this immersive interstellar adventure.
Delivers concise Python code and interprets non-English comments
The all-in-one Desktop & Docker AI application with built-in RAG, AI agents, No-code agent builder, MCP compatibility, and more.
Bridge between Ollama and MCP servers, enabling local LLMs to use Model Context Protocol tools
Fair-code workflow automation platform with native AI capabilities. Combine visual building with custom code, self-host or cloud, 400+ integrations.
🧑🚀 全世界最好的LLM资料总结(Agent框架、辅助编程、数据处理、模型训练、模型推理、o1 模型、MCP、小语言模型、视觉语言模型) | Summary of the world's best LLM resources.
Dify is an open-source LLM app development platform. Dify's intuitive interface combines AI workflow, RAG pipeline, agent capabilities, model management, observability features and more, letting you quickly go from prototype to production.
Reviews
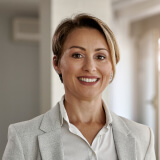
user_Q5AVhwM3
I've been using node-mcp23017 for a while now and it has exceeded my expectations. It offers seamless integration with the MCP23017 I/O expander and the documentation provided by kaihenzler is thorough and easy to follow. This library has significantly simplified my project development, and I highly recommend it to anyone working with MCP23017.