I find academic articles and books for research and literature reviews.
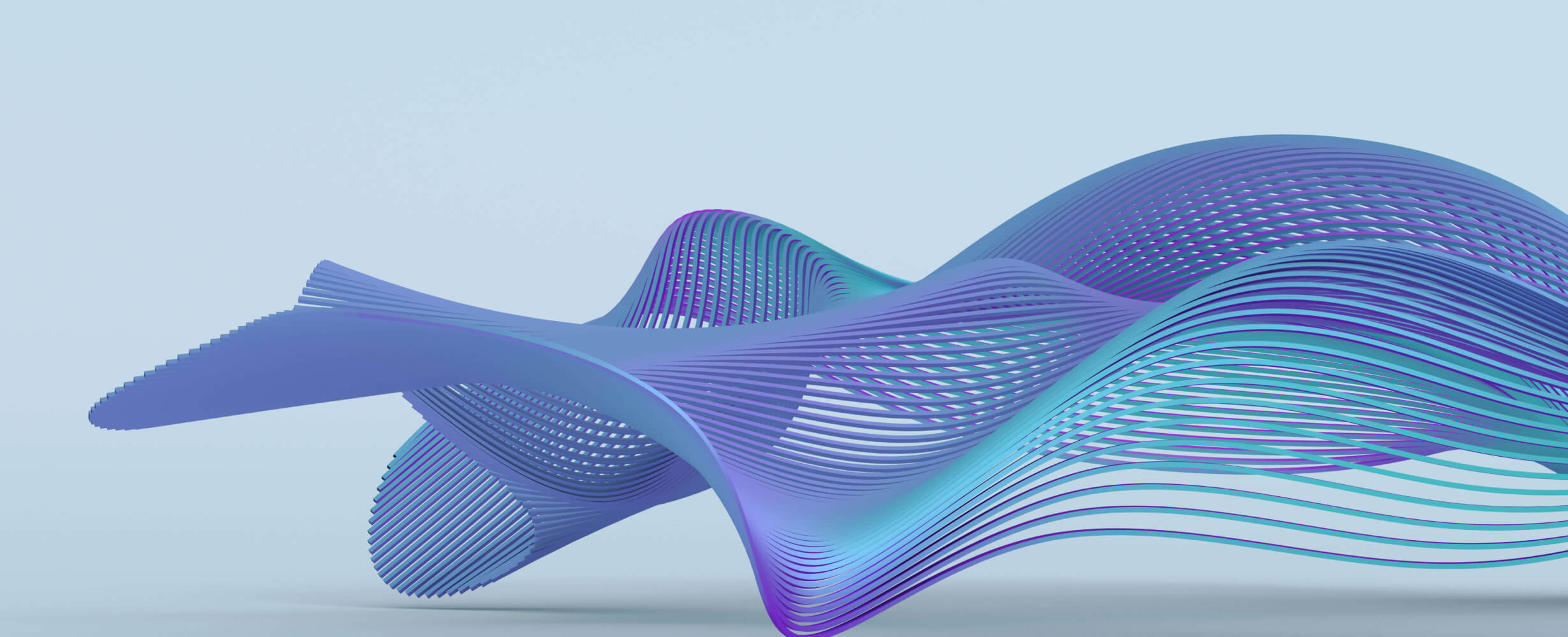
micropython-mcp2515
Micropython MCP2515 driver, porting from Arduino MCP2515 CAN interface library
4
Github Watches
14
Github Forks
28
Github Stars
micropython-mcp2515
Micropython MCP2515 driver, porting from Arduino MCP2515 CAN interface library
Getting Started
This MCP2515 driver could be used for any MCU or platform supported by Micropython, such as Pyboard, ESP32, ESP8286, etc.
"""
Following SPI drivers are supported, please adjust by your hardware
from .src import SPIESP8286 as SPI
from .src import SPIESP32 as SPI
The CAN driver can be initialized with default baudrate 10MHz
CAN(SPI(cs=YOUR_SPI_CS_PIN)) or
CAN(SPI(cs=YOUR_SPI_CS_PIN, baudrate=YOUR_DESIRED_BAUDRATE))
And here is an example to set filter for extended frame with ID 0x12345678
can.setFilter(RXF.RXF0, True, 0x12345678 | CAN_EFF_FLAG)
can.setFilterMask(MASK.MASK0, True, 0x1FFFFFFF | CAN_EFF_FLAG)
can.setFilterMask(MASK.MASK1, True, 0x1FFFFFFF | CAN_EFF_FLAG)
"""
import time
from src import (
CAN,
CAN_CLOCK,
CAN_EFF_FLAG,
CAN_ERR_FLAG,
CAN_RTR_FLAG,
CAN_SPEED,
ERROR,
)
from src import SPIESP32 as SPI
from src import CANFrame
def main():
# Initialization
can = CAN(SPI(cs=23))
# Configuration
if can.reset() != ERROR.ERROR_OK:
print("Can not reset for MCP2515")
return
if can.setBitrate(CAN_SPEED.CAN_500KBPS, CAN_CLOCK.MCP_8MHZ) != ERROR.ERROR_OK:
print("Can not set bitrate for MCP2515")
return
if can.setNormalMode() != ERROR.ERROR_OK:
print("Can not set normal mode for MCP2515")
return
# Prepare frames
data = b"\x12\x34\x56\x78\x9A\xBC\xDE\xF0"
sff_frame = CANFrame(can_id=0x7FF, data=data)
sff_none_data_frame = CANFrame(can_id=0x7FF)
err_frame = CANFrame(can_id=0x7FF | CAN_ERR_FLAG, data=data)
eff_frame = CANFrame(can_id=0x12345678 | CAN_EFF_FLAG, data=data)
eff_none_data_frame = CANFrame(can_id=0x12345678 | CAN_EFF_FLAG)
rtr_frame = CANFrame(can_id=0x7FF | CAN_RTR_FLAG)
rtr_with_eid_frame = CANFrame(can_id=0x12345678 | CAN_RTR_FLAG | CAN_EFF_FLAG)
rtr_with_data_frame = CANFrame(can_id=0x7FF | CAN_RTR_FLAG, data=data)
frames = [
sff_frame,
sff_none_data_frame,
err_frame,
eff_frame,
eff_none_data_frame,
rtr_frame,
rtr_with_eid_frame,
rtr_with_data_frame,
]
# Read all the time and send message in each second
end_time, n = time.ticks_add(time.ticks_ms(), 1000), -1
while True:
error, iframe = can.readMessage()
if error == ERROR.ERROR_OK:
print("RX {}".format(iframe))
if time.ticks_diff(time.ticks_ms(), end_time) >= 0:
end_time = time.ticks_add(time.ticks_ms(), 1000)
n += 1
n %= len(frames)
error = can.sendMessage(frames[n])
if error == ERROR.ERROR_OK:
print("TX {}".format(frames[n]))
else:
print("TX failed with error code {}".format(error))
if __name__ == "__main__":
try:
main()
except KeyboardInterrupt:
pass
Limitation
- Since Micropython is not fast enough, MCP2515 might send duplicated messages when CAN speed is too fast or SPI speed is too low. This is because previous message will still be transmitted repeatedly when MCP2515's SPI CS is not disabled timely.
相关推荐
Confidential guide on numerology and astrology, based of GG33 Public information
Converts Figma frames into front-end code for various mobile frameworks.
Advanced software engineer GPT that excels through nailing the basics.
Take an adjectivised noun, and create images making it progressively more adjective!
Embark on a thrilling diplomatic quest across a galaxy on the brink of war. Navigate complex politics and alien cultures to forge peace and avert catastrophe in this immersive interstellar adventure.
Discover the most comprehensive and up-to-date collection of MCP servers in the market. This repository serves as a centralized hub, offering an extensive catalog of open-source and proprietary MCP servers, complete with features, documentation links, and contributors.
Micropython I2C-based manipulation of the MCP series GPIO expander, derived from Adafruit_MCP230xx
The all-in-one Desktop & Docker AI application with built-in RAG, AI agents, No-code agent builder, MCP compatibility, and more.
Bridge between Ollama and MCP servers, enabling local LLMs to use Model Context Protocol tools
Fair-code workflow automation platform with native AI capabilities. Combine visual building with custom code, self-host or cloud, 400+ integrations.
🧑🚀 全世界最好的LLM资料总结(Agent框架、辅助编程、数据处理、模型训练、模型推理、o1 模型、MCP、小语言模型、视觉语言模型) | Summary of the world's best LLM resources.
Reviews
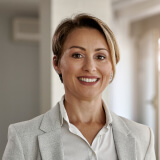
user_hiEL9zqL
The micropython-mcp2515 by jxltom is an excellent tool for integrating MCP2515 CAN controllers using MicroPython. It's well-documented and easy to use, making it perfect for hobbyists and professionals alike. The repository at https://github.com/jxltom/micropython-mcp2515 offers comprehensive information and examples, which are greatly helpful. Highly recommended for anyone working with CAN protocol and MicroPython!