I craft unique cereal names, stories, and ridiculously cute Cereal Baby images.
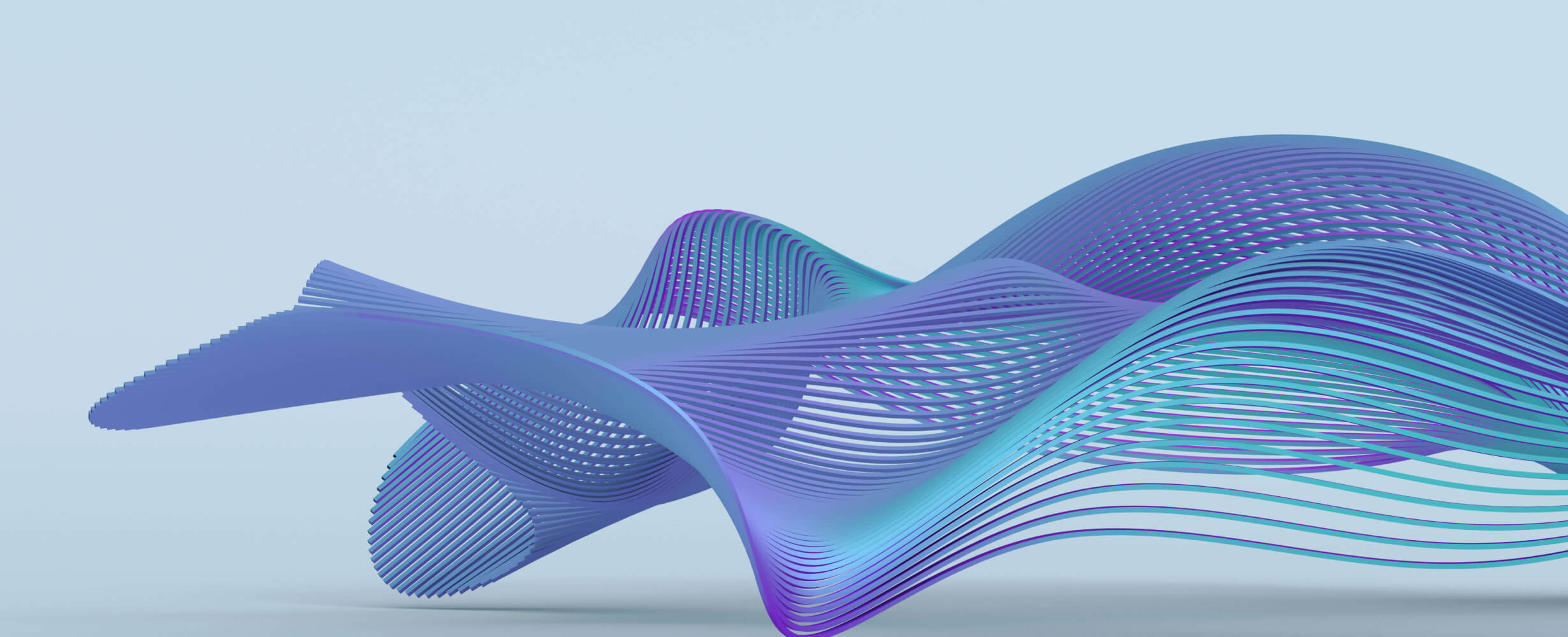
ezmcp
Easy-to-use MCP server framework specialized for SSE.
3 years
Works with Finder
1
Github Watches
6
Github Forks
17
Github Stars
ezmcp
Easy-to-use MCP server framework specialized for SSE.
Overview
ezmcp is a lightweight framework that makes it easy to create MCP-compatible servers using a FastAPI-like syntax. It provides a simple decorator-based API for defining tools that can be called by MCP clients.
Features
- FastAPI-style decorator API for defining MCP tools
- Automatic parameter validation and type conversion
- Automatic generation of tool schemas from function signatures
- Built-in support for SSE transport
- FastAPI-style middleware support
- Easy integration with existing Starlette applications
- Interactive documentation page for exploring and testing tools
Installation
pip install ezmcp
Quick Start
from ezmcp import ezmcp, TextContent
# Create an ezmcp application
app = ezmcp("my-app")
# Define a tool
@app.tool(description="Echo a message back to the user")
async def echo(message: str):
"""Echo a message back to the user."""
return [TextContent(type="text", text=f"Echo: {message}")]
# Run the application
if __name__ == "__main__":
app.run(host="0.0.0.0", port=8000)
Once the server is running, you can:
- Access the interactive documentation at
http://localhost:8000/docs
- Connect to the SSE endpoint at
http://localhost:8000/sse
Middleware
ezmcp supports middleware similar to FastAPI, allowing you to add behavior that is applied across your entire application.
from starlette.requests import Request
from ezmcp import TextContent, ezmcp
app = ezmcp("my-app")
@app.middleware
async def process_time_middleware(request: Request, call_next):
"""Add a header with the processing time."""
import time
start_time = time.perf_counter()
response = await call_next(request)
process_time = time.perf_counter() - start_time
response.headers["X-Process-Time"] = str(process_time)
return response
@app.tool(description="Echo a message back to the user")
async def echo(message: str):
"""Echo a message back to the user."""
return [TextContent(type="text", text=f"Echo: {message}")]
For more information on middleware, see the middleware documentation.
Documentation
For more detailed documentation, see the ezmcp/README.md file.
License
MIT
commands
- install for test
pdm install -G test
- install for dev
pdm install -G dev
相关推荐
Converts Figma frames into front-end code for various mobile frameworks.
Oede knorrepot die vasthoudt an de goeie ouwe tied van 't boerenleven
I find academic articles and books for research and literature reviews.
A unified API gateway for integrating multiple etherscan-like blockchain explorer APIs with Model Context Protocol (MCP) support for AI assistants.
Mirror ofhttps://github.com/suhail-ak-s/mcp-typesense-server
本项目是一个钉钉MCP(Message Connector Protocol)服务,提供了与钉钉企业应用交互的API接口。项目基于Go语言开发,支持员工信息查询和消息发送等功能。
Short and sweet example MCP server / client implementation for Tools, Resources and Prompts.
Reviews
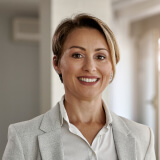
user_DaoQO8tR
As a dedicated MCP application user, I find the Mifos X - AI - Model Context Protocol (MCP) for Apache Fineract® by openMF to be incredibly efficient and user-friendly. It seamlessly integrates advanced AI capabilities, enhancing the operational workflows and decision-making processes. Highly recommended for organizations looking to leverage AI in their financial services. Check it out here: https://mcp.so/server/mcp-mifosx/openMF