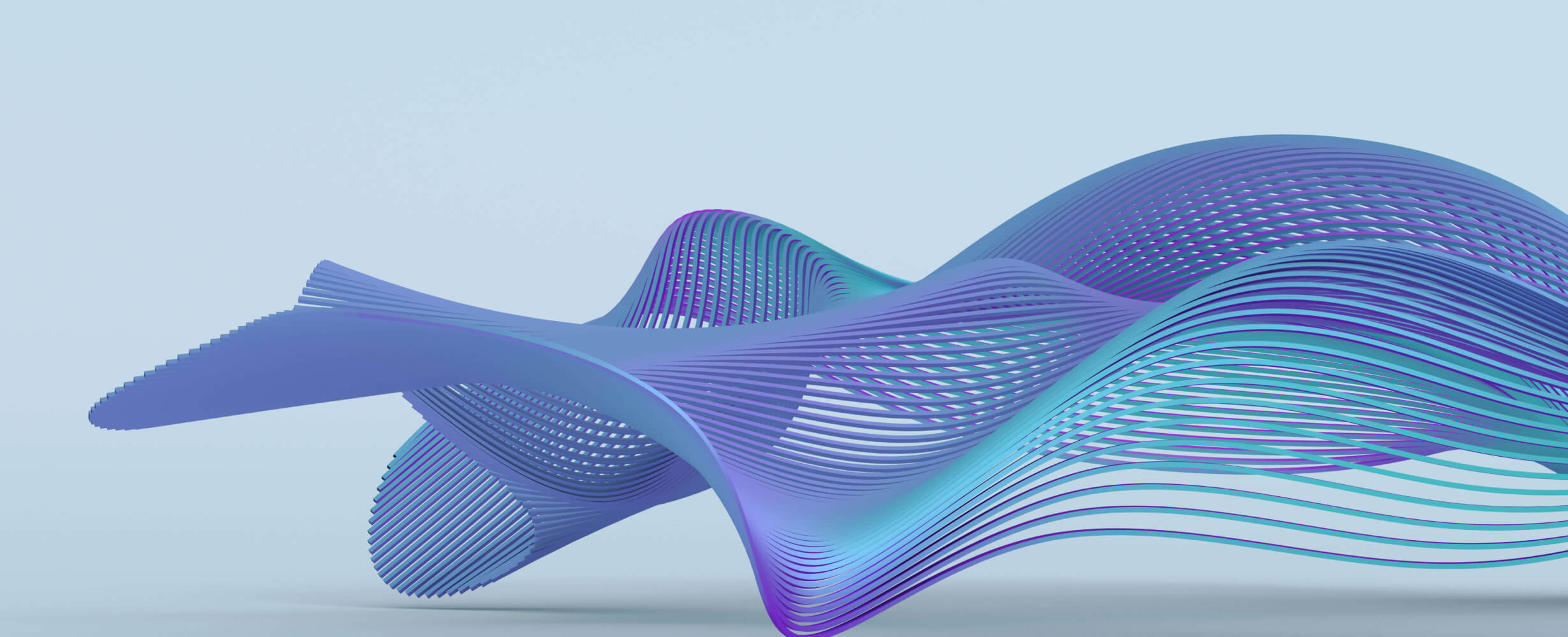
multiplatform-cursor-mcp
1
Github Watches
14
Github Forks
58
Github Stars
Cursor MCP (Model Context Protocol)
Cursor MCP is a bridge between Claude's desktop application and the Cursor editor, enabling seamless AI-powered automation and multi-instance management. It's part of the broader Model Context Protocol (MCP) ecosystem, allowing Cursor to interact with various AI models and services through standardized interfaces.
Overview
🤖 AI Integration
- Direct integration with Claude's desktop application
- Ability to leverage other MCP-compatible AI services
- Real-time context sharing between AI and editor
- AI-powered automation and code generation
🔌 MCP Protocol Support
- Standardized communication with AI models
- Extensible plugin system for additional MCPs
- Context-aware command execution
- Secure token-based authentication
🖥️ Cross-Platform Window Management
- Seamlessly manage Cursor editor windows across operating systems
- Focus, minimize, restore, and arrange windows programmatically
- Track window state changes and positions
- Handle multiple Cursor instances simultaneously
⌨️ Input Automation
- AI-driven keyboard input with support for:
- Code generation and insertion
- Refactoring operations
- Context-aware completions
- Multi-cursor editing
- Intelligent mouse automation including:
- Smart selection
- Context-menu operations
- AI-guided navigation
🔄 Process Management
- AI-orchestrated instance management
- Smart workspace organization
- Automatic context preservation
- Intelligent session recovery
MCP Integration
Claude Desktop Integration
import { ClaudeMCP } from 'cursor-mcp/claude'
// Connect to Claude's desktop app
const claude = await ClaudeMCP.connect()
// Execute AI-powered operations
await claude.generateCode({
prompt: 'Create a React component',
context: currentFileContent,
language: 'typescript'
})
// Get AI suggestions
const suggestions = await claude.getSuggestions({
code: selectedText,
type: 'refactor'
})
Using Multiple MCPs
import { MCPRegistry } from 'cursor-mcp/registry'
// Register available MCPs
MCPRegistry.register('claude', ClaudeMCP)
MCPRegistry.register('github-copilot', CopilotMCP)
// Use different AI services
const claude = await MCPRegistry.get('claude')
const copilot = await MCPRegistry.get('github-copilot')
// Compare suggestions
const claudeSuggestions = await claude.getSuggestions(context)
const copilotSuggestions = await copilot.getSuggestions(context)
Custom MCP Integration
import { BaseMCP, MCPProvider } from 'cursor-mcp/core'
class CustomMCP extends BaseMCP implements MCPProvider {
async connect() {
// Custom connection logic
}
async generateSuggestions(context: CodeContext) {
// Custom AI integration
}
}
// Register custom MCP
MCPRegistry.register('custom-ai', CustomMCP)
Configuration
The tool can be configured through environment variables or a config file at:
- Windows:
%LOCALAPPDATA%\cursor-mcp\config\config.json
- macOS:
~/Library/Application Support/cursor-mcp/config/config.json
- Linux:
~/.config/cursor-mcp/config.json
Example configuration:
{
"mcp": {
"claude": {
"enabled": true,
"apiKey": "${CLAUDE_API_KEY}",
"contextWindow": 100000
},
"providers": {
"github-copilot": {
"enabled": true,
"auth": "${GITHUB_TOKEN}"
}
}
},
"autoStart": true,
"maxInstances": 4,
"windowArrangement": "grid",
"logging": {
"level": "info",
"file": "cursor-mcp.log"
}
}
Installation
Windows
# Run as Administrator
Invoke-WebRequest -Uri "https://github.com/your-org/cursor-mcp/releases/latest/download/cursor-mcp-windows.zip" -OutFile "cursor-mcp.zip"
Expand-Archive -Path "cursor-mcp.zip" -DestinationPath "."
.\windows.ps1
macOS
# Run with sudo
curl -L "https://github.com/your-org/cursor-mcp/releases/latest/download/cursor-mcp-macos.zip" -o "cursor-mcp.zip"
unzip cursor-mcp.zip
sudo ./macos.sh
Linux
# Run with sudo
curl -L "https://github.com/your-org/cursor-mcp/releases/latest/download/cursor-mcp-linux.zip" -o "cursor-mcp.zip"
unzip cursor-mcp.zip
sudo ./linux.sh
Usage
Basic Usage
import { CursorInstanceManager } from 'cursor-mcp'
// Get the instance manager
const manager = CursorInstanceManager.getInstance()
// Start a new Cursor instance
await manager.startNewInstance()
// Get all running instances
const instances = await manager.getRunningInstances()
// Focus a specific instance
await manager.focusInstance(instances[0])
// Close all instances
await manager.closeAllInstances()
Window Management
import { WindowManager } from 'cursor-mcp'
const windowManager = WindowManager.getInstance()
// Find all Cursor windows
const windows = await windowManager.findCursorWindows()
// Focus a window
await windowManager.focusWindow(windows[0])
// Arrange windows side by side
await windowManager.arrangeWindows(windows, 'sideBySide')
// Minimize all windows
for (const window of windows) {
await windowManager.minimizeWindow(window)
}
Input Automation
import { InputAutomationService } from 'cursor-mcp'
const inputService = InputAutomationService.getInstance()
// Type text
await inputService.typeText('Hello, World!')
// Send keyboard shortcuts
if (process.platform === 'darwin') {
await inputService.sendKeys(['command', 'c'])
} else {
await inputService.sendKeys(['control', 'c'])
}
// Mouse operations
await inputService.moveMouse(100, 100)
await inputService.mouseClick('left')
await inputService.mouseDrag(100, 100, 200, 200)
How It Works
Bridge Architecture
This tool acts as a middleware layer between Cursor and MCP servers:
-
Cursor Integration:
- Monitors Cursor's file system events
- Captures editor state and context
- Injects responses back into the editor
- Manages window and process automation
-
MCP Protocol Translation:
- Translates Cursor's internal events into MCP protocol messages
- Converts MCP responses into Cursor-compatible actions
- Maintains session state and context
- Handles authentication and security
-
Server Communication:
- Connects to Claude's desktop app MCP server
- Routes requests to appropriate AI providers
- Manages concurrent connections to multiple MCPs
- Handles fallbacks and error recovery
graph LR
A[Cursor Editor] <--> B[Cursor MCP Bridge]
B <--> C[Claude Desktop MCP]
B <--> D[GitHub Copilot MCP]
B <--> E[Custom AI MCPs]
Example Workflow
-
Code Completion Request:
// 1. Cursor Event (File Change) // When user types in Cursor: function calculateTotal(items) { // Calculate the total price of items| <-- cursor position // 2. Bridge Translation const event = { type: 'completion_request', context: { file: 'shopping-cart.ts', line: 2, prefix: '// Calculate the total price of items', language: 'typescript', cursor_position: 43 } } // 3. MCP Protocol Message await mcpServer.call('generate_completion', { prompt: event.context, max_tokens: 150, temperature: 0.7 }) // 4. Response Translation // Bridge converts MCP response: const response = `return items.reduce((total, item) => { return total + (item.price * item.quantity); }, 0);` // 5. Cursor Integration // Bridge injects the code at cursor position
-
Code Refactoring:
// 1. Cursor Event (Command) // User selects code and triggers refactor command const oldCode = ` if (user.age >= 18) { if (user.hasLicense) { if (car.isAvailable) { rentCar(user, car); } } } ` // 2. Bridge Translation const event = { type: 'refactor_request', context: { selection: oldCode, command: 'simplify_nesting' } } // 3. MCP Protocol Message await mcpServer.call('refactor_code', { code: event.context.selection, style: 'simplified', maintain_logic: true }) // 4. Response Translation const response = ` const canRentCar = user.age >= 18 && user.hasLicense && car.isAvailable; if (canRentCar) { rentCar(user, car); } ` // 5. Cursor Integration // Bridge replaces selected code
-
Multi-File Context:
// 1. Cursor Event (File Dependencies) // When user requests help with a component // 2. Bridge Translation const event = { type: 'context_request', files: { 'UserProfile.tsx': '...', 'types.ts': '...', 'api.ts': '...' }, focus_file: 'UserProfile.tsx' } // 3. MCP Protocol Message await mcpServer.call('analyze_context', { files: event.files, primary_file: event.focus_file, analysis_type: 'component_dependencies' }) // 4. Response Processing // Bridge maintains context across requests
Integration Methods
-
File System Monitoring:
import { FileSystemWatcher } from 'cursor-mcp/watcher' const watcher = new FileSystemWatcher({ paths: ['/path/to/cursor/workspace'], events: ['change', 'create', 'delete'] }) watcher.on('change', async (event) => { const mcpMessage = await bridge.translateEvent(event) await mcpServer.send(mcpMessage) })
-
Window Integration:
import { CursorWindow } from 'cursor-mcp/window' const window = new CursorWindow() // Inject AI responses await window.injectCode({ position: cursorPosition, code: mcpResponse.code, animate: true // Smooth typing animation }) // Handle user interactions window.onCommand('refactor', async (selection) => { const mcpMessage = await bridge.createRefactorRequest(selection) const response = await mcpServer.send(mcpMessage) await window.applyRefactoring(response) })
-
Context Management:
import { ContextManager } from 'cursor-mcp/context' const context = new ContextManager() // Track file dependencies await context.addFile('component.tsx') await context.trackDependencies() // Maintain conversation history context.addMessage({ role: 'user', content: 'Refactor this component' }) // Send to MCP server const response = await mcpServer.send({ type: 'refactor', context: context.getFullContext() })
Security
- Secure token-based authentication for AI services
- Encrypted communication channels
- Sandboxed execution environment
- Fine-grained permission controls
Requirements
Windows
- Windows 10 or later
- Node.js 18 or later
- Administrator privileges for installation
macOS
- macOS 10.15 (Catalina) or later
- Node.js 18 or later
- Xcode Command Line Tools
- Accessibility permissions for Terminal
Linux
- X11 display server
- Node.js 18 or later
- xdotool
- libxtst-dev
- libpng++-dev
- build-essential
Development
Setup
# Clone the repository
git clone https://github.com/your-org/cursor-mcp.git
cd cursor-mcp
# Install dependencies
npm install
# Build the project
npm run build
# Run tests
npm test
Running Tests
# Run all tests
npm test
# Run specific test suite
npm test -- window-management
# Run with coverage
npm run test:coverage
Contributing
We welcome contributions! Please see our Contributing Guide for details.
License
This project is licensed under the MIT License - see the LICENSE file for details.
Support
Acknowledgments
- Cursor Editor - The AI-first code editor
- Claude AI - Advanced AI assistant
- @nut-tree/nut-js - Cross-platform automation
- active-win - Window detection
相关推荐
I find academic articles and books for research and literature reviews.
Emulating Dr. Jordan B. Peterson's style in providing life advice and insights.
Confidential guide on numerology and astrology, based of GG33 Public information
Your go-to expert in the Rust ecosystem, specializing in precise code interpretation, up-to-date crate version checking, and in-depth source code analysis. I offer accurate, context-aware insights for all your Rust programming questions.
Converts Figma frames into front-end code for various mobile frameworks.
Advanced software engineer GPT that excels through nailing the basics.
Take an adjectivised noun, and create images making it progressively more adjective!
Discover the most comprehensive and up-to-date collection of MCP servers in the market. This repository serves as a centralized hub, offering an extensive catalog of open-source and proprietary MCP servers, complete with features, documentation links, and contributors.
Micropython I2C-based manipulation of the MCP series GPIO expander, derived from Adafruit_MCP230xx
The all-in-one Desktop & Docker AI application with built-in RAG, AI agents, No-code agent builder, MCP compatibility, and more.
Fair-code workflow automation platform with native AI capabilities. Combine visual building with custom code, self-host or cloud, 400+ integrations.
Bridge between Ollama and MCP servers, enabling local LLMs to use Model Context Protocol tools
🧑🚀 全世界最好的LLM资料总结(Agent框架、辅助编程、数据处理、模型训练、模型推理、o1 模型、MCP、小语言模型、视觉语言模型) | Summary of the world's best LLM resources.
Reviews
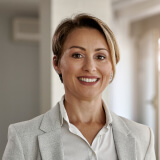
user_PZkDsCzt
The multiplatform-cursor-mcp by johnneerdael is an absolute game-changer for developers needing seamless cursor functionality across multiple platforms. The ease of integration and robust performance has significantly improved my productivity. I highly recommend checking out the GitHub link for more details and to see its full potential.