I craft unique cereal names, stories, and ridiculously cute Cereal Baby images.
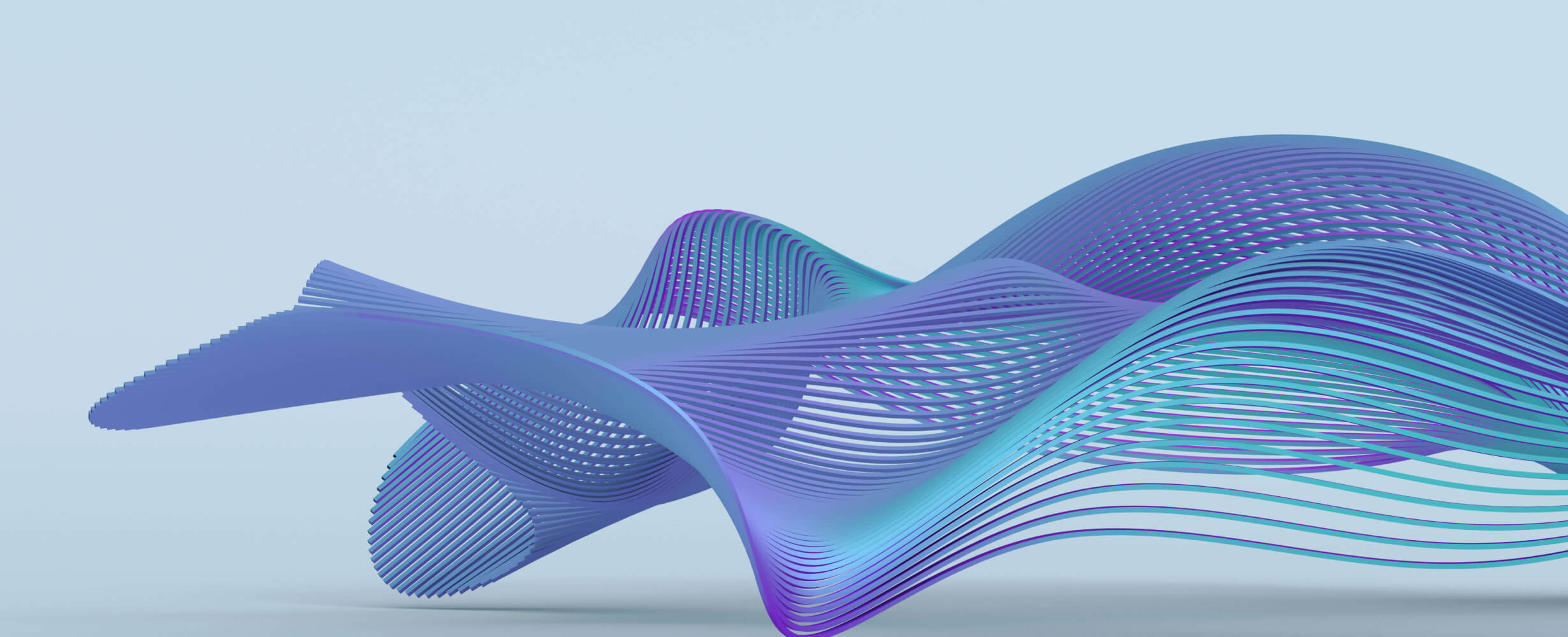
advanced-homeassistant-mcp
An advanced MCP server for Home Assistant. 🔋 Batteries included.
1
Github Watches
5
Github Forks
14
Github Stars
Home Assistant Model Context Protocol (MCP)
A standardized protocol for AI assistants to interact with Home Assistant, providing a secure, typed, and extensible interface for controlling smart home devices.
Overview
The Model Context Protocol (MCP) server acts as a bridge between AI models (like Claude, GPT, etc.) and Home Assistant, enabling AI assistants to:
- Execute commands on Home Assistant devices
- Retrieve information about the smart home
- Stream responses for long-running operations
- Validate parameters and inputs
- Provide consistent error handling
Features
- Modular Architecture - Clean separation between transport, middleware, and tools
- Typed Interface - Fully TypeScript typed for better developer experience
-
Multiple Transports:
- Standard I/O (stdin/stdout) for CLI integration
- HTTP/REST API with Server-Sent Events support for streaming
- Middleware System - Validation, logging, timeout, and error handling
-
Built-in Tools:
- Light control (brightness, color, etc.)
- Climate control (thermostats, HVAC)
- More to come...
- Extensible Plugin System - Easily add new tools and capabilities
- Streaming Responses - Support for long-running operations
- Parameter Validation - Using Zod schemas
- Claude & Cursor Integration - Ready-made utilities for AI assistants
Getting Started
Prerequisites
- Node.js 16+
- Home Assistant instance (or you can use the mock implementations for testing)
Installation
# Clone the repository
git clone https://github.com/your-repo/homeassistant-mcp.git
# Install dependencies
cd homeassistant-mcp
npm install
# Build the project
npm run build
Running the Server
# Start with standard I/O transport (for AI assistant integration)
npm start -- --stdio
# Start with HTTP transport (for API access)
npm start -- --http
# Start with both transports
npm start -- --stdio --http
Configuration
Configure the server using environment variables or a .env
file:
# Server configuration
PORT=3000
NODE_ENV=development
# Execution settings
EXECUTION_TIMEOUT=30000
STREAMING_ENABLED=true
# Transport settings
USE_STDIO_TRANSPORT=true
USE_HTTP_TRANSPORT=true
# Debug and logging
DEBUG_MODE=false
DEBUG_STDIO=false
DEBUG_HTTP=false
SILENT_STARTUP=false
# CORS settings
CORS_ORIGIN=*
Architecture
The MCP server is built with a layered architecture:
- Transport Layer - Handles communication protocols (stdio, HTTP)
- Middleware Layer - Processes requests through a pipeline
- Tool Layer - Implements specific functionality
- Resource Layer - Manages stateful resources
Tools
Tools are the primary way to add functionality to the MCP server. Each tool:
- Has a unique name
- Accepts typed parameters
- Returns typed results
- Can stream partial results
- Validates inputs and outputs
Example tool registration:
import { LightsControlTool } from "./tools/homeassistant/lights.tool.js";
import { ClimateControlTool } from "./tools/homeassistant/climate.tool.js";
// Register tools
server.registerTool(new LightsControlTool());
server.registerTool(new ClimateControlTool());
API
When running with HTTP transport, the server provides a JSON-RPC 2.0 API:
-
POST /api/mcp/jsonrpc
- Execute a tool -
GET /api/mcp/stream
- Connect to SSE stream for real-time updates -
GET /api/mcp/info
- Get server information -
GET /health
- Health check endpoint
Integration with AI Models
Claude Integration
import { createClaudeToolDefinitions } from "./mcp/index.js";
// Generate Claude-compatible tool definitions
const claudeTools = createClaudeToolDefinitions([
new LightsControlTool(),
new ClimateControlTool()
]);
// Use with Claude API
const messages = [
{ role: "user", content: "Turn on the lights in the living room" }
];
const response = await claude.messages.create({
model: "claude-3-opus-20240229",
messages,
tools: claudeTools
});
Cursor Integration
To use the Home Assistant MCP server with Cursor, add the following to your .cursor/config/config.json
file:
{
"mcpServers": {
"homeassistant-mcp": {
"command": "bash",
"args": ["-c", "cd ${workspaceRoot} && bun run dist/index.js --stdio 2>/dev/null | grep -E '\\{\"jsonrpc\":\"2\\.0\"'"],
"env": {
"NODE_ENV": "development",
"USE_STDIO_TRANSPORT": "true",
"DEBUG_STDIO": "true"
}
}
}
}
This configuration:
- Runs the MCP server with stdio transport
- Redirects all stderr output to /dev/null
- Uses grep to filter stdout for lines containing
{"jsonrpc":"2.0"
, ensuring clean JSON-RPC output
Troubleshooting Cursor Integration
If you encounter a "failed to create client" error when using the MCP server with Cursor:
-
Make sure you're using the correct command and arguments in your Cursor configuration
- The bash script approach ensures only valid JSON-RPC messages reach Cursor
- Ensure the server is built by running
bun run build
before trying to connect
-
Ensure the server is properly outputting JSON-RPC messages to stdout:
bun run dist/index.js --stdio 2>/dev/null | grep -E '\{"jsonrpc":"2\.0"' > json_only.txt
Then examine json_only.txt to verify it contains only valid JSON-RPC messages.
-
Make sure grep is installed on your system (it should be available by default on most systems)
-
Try rebuilding the server with:
bun run build
-
Enable debug mode by setting
DEBUG_STDIO=true
in the environment variables
If the issue persists, you can try:
- Restarting Cursor
- Clearing Cursor's cache (Help > Developer > Clear Cache and Reload)
- Using a similar approach with Node.js:
{ "command": "bash", "args": ["-c", "cd ${workspaceRoot} && node dist/index.js --stdio 2>/dev/null | grep -E '\\{\"jsonrpc\":\"2\\.0\"'"] }
License
MIT
Contributing
Contributions are welcome! Please feel free to submit a Pull Request.
MCP Server for Home Assistant 🏠🤖
Overview 🌐
MCP (Model Context Protocol) Server is my lightweight integration tool for Home Assistant, providing a flexible interface for device management and automation. It's designed to be fast, secure, and easy to use. Built with Bun for maximum performance.
Core Features ✨
- 🔌 Basic device control via REST API
- 📡 WebSocket/Server-Sent Events (SSE) for state updates
- 🤖 Simple automation rule management
- 🔐 JWT-based authentication
- 🔄 Standard I/O (stdio) transport for integration with Claude and other AI assistants
Why Bun? 🚀
I chose Bun as the runtime for several key benefits:
-
⚡ Blazing Fast Performance
- Up to 4x faster than Node.js
- Built-in TypeScript support
- Optimized file system operations
-
🎯 All-in-One Solution
- Package manager (faster than npm/yarn)
- Bundler (no webpack needed)
- Test runner (built-in testing)
- TypeScript transpiler
-
🔋 Built-in Features
- SQLite3 driver
- .env file loading
- WebSocket client/server
- File watcher
- Test runner
-
💾 Resource Efficient
- Lower memory usage
- Faster cold starts
- Better CPU utilization
-
🔄 Node.js Compatibility
- Runs most npm packages
- Compatible with Express/Fastify
- Native Node.js APIs
Prerequisites 📋
- 🚀 Bun runtime (v1.0.26+)
- 🏡 Home Assistant instance
- 🐳 Docker (optional, recommended for deployment)
- 🖥️ Node.js 18+ (optional, for speech features)
- 🎮 NVIDIA GPU with CUDA support (optional, for faster speech processing)
Quick Start 🚀
- Clone my repository:
git clone https://github.com/jango-blockchained/homeassistant-mcp.git
cd homeassistant-mcp
- Set up the environment:
# Make my setup script executable
chmod +x scripts/setup-env.sh
# Run setup (defaults to development)
./scripts/setup-env.sh
# Or specify an environment:
NODE_ENV=production ./scripts/setup-env.sh
# Force override existing files:
./scripts/setup-env.sh --force
- Configure your settings:
- Edit
.env
file with your Home Assistant details - Required: Add your
HASS_TOKEN
(long-lived access token)
- Build and launch with Docker:
# Standard build
./docker-build.sh
# Launch:
docker compose up -d
Docker Build Options 🐳
My Docker build script (docker-build.sh
) supports different configurations:
1. Standard Build
./docker-build.sh
- Basic MCP server functionality
- REST API and WebSocket support
- No speech features
2. Speech-Enabled Build
./docker-build.sh --speech
- Includes wake word detection
- Speech-to-text capabilities
- Pulls required images:
-
onerahmet/openai-whisper-asr-webservice
-
rhasspy/wyoming-openwakeword
-
3. GPU-Accelerated Build
./docker-build.sh --speech --gpu
- All speech features
- CUDA GPU acceleration
- Optimized for faster processing
- Float16 compute type for better performance
Build Features
- 🔄 Automatic resource allocation
- 💾 Memory-aware building
- 📊 CPU quota management
- 🧹 Automatic cleanup
- 📝 Detailed build logs
- 📊 Build summary and status
Environment Configuration 🔧
I've implemented a hierarchical configuration system:
File Structure 📁
-
.env.example
- My template with all options -
.env
- Your configuration (copy from .env.example) - Environment overrides:
-
.env.dev
- Development settings -
.env.prod
- Production settings -
.env.test
- Test settings
-
Loading Priority ⚡
Files load in this order:
-
.env
(base config) - Environment-specific file:
-
NODE_ENV=development
→.env.dev
-
NODE_ENV=production
→.env.prod
-
NODE_ENV=test
→.env.test
-
Later files override earlier ones.
Development 💻
# Install dependencies
bun install
# Run in development mode
bun run dev
# Run tests
bun test
# Run with hot reload
bun --hot run dev
# Build for production
bun build ./src/index.ts --target=bun
# Run production build
bun run start
Performance Comparison 📊
Operation | Bun | Node.js |
---|---|---|
Install Dependencies | ~2s | ~15s |
Cold Start | 300ms | 1000ms |
Build Time | 150ms | 4000ms |
Memory Usage | ~150MB | ~400MB |
Documentation 📚
Core Documentation
Advanced Features
- Natural Language Processing - AI-powered automation analysis and control
- Custom Prompts Guide - Create and customize AI behavior
- Extras & Tools - Additional utilities and advanced features
Client Integration 🔗
Cursor Integration 🖱️
Add to .cursor/config/config.json
:
{
"mcpServers": {
"homeassistant-mcp": {
"command": "bash",
"args": ["-c", "cd ${workspaceRoot} && bun run dist/index.js --stdio 2>/dev/null | grep -E '\\{\"jsonrpc\":\"2\\.0\"'"],
"env": {
"NODE_ENV": "development",
"USE_STDIO_TRANSPORT": "true",
"DEBUG_STDIO": "true"
}
}
}
}
Claude Desktop 💬
Add to your Claude config:
{
"mcpServers": {
"homeassistant-mcp": {
"command": "bun",
"args": ["run", "start", "--port", "8080"],
"env": {
"NODE_ENV": "production"
}
}
}
}
Command Line 💻
Windows users can use the provided script:
- Go to
scripts
directory - Run
start_mcp.cmd
Additional Features
Speech Features 🎤
MCP Server optionally supports speech processing capabilities:
- 🗣️ Wake word detection ("hey jarvis", "ok google", "alexa")
- 🎯 Speech-to-text using fast-whisper
- 🌍 Multiple language support
- 🚀 GPU acceleration support
Speech Features Setup
Prerequisites
- 🐳 Docker installed and running
- 🎮 NVIDIA GPU with CUDA (optional)
- 💾 4GB+ RAM (8GB+ recommended)
Configuration
- Enable speech in
.env
:
ENABLE_SPEECH_FEATURES=true
ENABLE_WAKE_WORD=true
ENABLE_SPEECH_TO_TEXT=true
WHISPER_MODEL_PATH=/models
WHISPER_MODEL_TYPE=base
- Choose your STT engine:
# For standard Whisper
STT_ENGINE=whisper
# For Fast Whisper (GPU recommended)
STT_ENGINE=fast-whisper
CUDA_VISIBLE_DEVICES=0 # Set GPU device
Available Models 🤖
Choose based on your needs:
-
tiny.en
: Fastest, basic accuracy -
base.en
: Good balance (recommended) -
small.en
: Better accuracy, slower -
medium.en
: High accuracy, resource intensive -
large-v2
: Best accuracy, very resource intensive
Launch with Speech Features
# Build with speech support
./docker-build.sh --speech
# Launch with speech features:
docker compose -f docker-compose.yml -f docker-compose.speech.yml up -d
Extra Tools 🛠️
I've included several powerful tools in the extra/
directory to enhance your Home Assistant experience:
-
Home Assistant Analyzer CLI (
ha-analyzer-cli.ts
)- Deep automation analysis using AI models
- Security vulnerability scanning
- Performance optimization suggestions
- System health metrics
-
Speech-to-Text Example (
speech-to-text-example.ts
)- Wake word detection
- Speech-to-text transcription
- Multiple language support
- GPU acceleration support
-
Claude Desktop Setup (
claude-desktop-macos-setup.sh
)- Automated Claude Desktop installation for macOS
- Environment configuration
- MCP integration setup
See Extras Documentation for detailed usage instructions and examples.
License 📄
MIT License. See LICENSE for details.
Author 👨💻
Created by jango-blockchained
Running with Standard I/O Transport 📝
MCP Server supports a JSON-RPC 2.0 stdio transport mode for direct integration with AI assistants like Claude:
MCP Stdio Features
✅ JSON-RPC 2.0 Compatibility: Full support for the MCP protocol standard
✅ NPX Support: Run directly without installation using npx homeassistant-mcp
✅ Auto Configuration: Creates necessary directories and default configuration
✅ Cross-Platform: Works on macOS, Linux, and Windows
✅ Claude Desktop Integration: Ready to use with Claude Desktop
✅ Parameter Validation: Automatic validation of tool parameters
✅ Error Handling: Standardized error codes and handling
✅ Detailed Logging: Logs to files without polluting stdio
Option 1: Using NPX (Easiest)
Run the MCP server directly without installation using npx:
# Basic usage
npx homeassistant-mcp
# Or with environment variables
HASS_URL=http://your-ha-instance:8123 HASS_TOKEN=your_token npx homeassistant-mcp
This will:
- Install the package temporarily
- Automatically run in stdio mode with JSON-RPC 2.0 transport
- Create a logs directory for logging
- Create a default .env file if not present
Perfect for integration with Claude Desktop or other MCP clients.
Integrating with Claude Desktop
To use MCP with Claude Desktop:
- Open Claude Desktop settings
- Go to the "Advanced" tab
- Under "MCP Server", select "Custom"
- Enter the command:
npx homeassistant-mcp
- Click "Save"
Claude will now use the MCP server for Home Assistant integration, allowing you to control your smart home directly through Claude.
Option 2: Local Installation
-
Update your
.env
file to enable stdio transport:USE_STDIO_TRANSPORT=true
-
Run the server using the stdio-start script:
./stdio-start.sh
Available options:
./stdio-start.sh --debug # Enable debug mode ./stdio-start.sh --rebuild # Force rebuild ./stdio-start.sh --help # Show help
When running in stdio mode:
- The server communicates via stdin/stdout using JSON-RPC 2.0 format
- No HTTP server is started
- Console logging is disabled to avoid polluting the stdio stream
- All logs are written to the log files in the
logs/
directory
JSON-RPC 2.0 Message Format
Request Format
{
"jsonrpc": "2.0",
"id": "unique-request-id",
"method": "tool-name",
"params": {
"param1": "value1",
"param2": "value2"
}
}
Response Format
{
"jsonrpc": "2.0",
"id": "unique-request-id",
"result": {
// Tool-specific result data
}
}
Error Response Format
{
"jsonrpc": "2.0",
"id": "unique-request-id",
"error": {
"code": -32000,
"message": "Error message",
"data": {} // Optional error details
}
}
Notification Format (Server to Client)
{
"jsonrpc": "2.0",
"method": "notification-type",
"params": {
// Notification data
}
}
Supported Error Codes
Code | Description | Meaning |
---|---|---|
-32700 | Parse error | Invalid JSON was received |
-32600 | Invalid request | JSON is not a valid request object |
-32601 | Method not found | Method does not exist or is unavailable |
-32602 | Invalid params | Invalid method parameters |
-32603 | Internal error | Internal JSON-RPC error |
-32000 | Tool execution | Error executing the tool |
-32001 | Validation error | Parameter validation failed |
Integrating with Claude Desktop
To use this MCP server with Claude Desktop:
-
Create or edit your Claude Desktop configuration:
# On macOS nano ~/Library/Application\ Support/Claude/claude_desktop_config.json # On Linux nano ~/.config/Claude/claude_desktop_config.json # On Windows notepad %APPDATA%\Claude\claude_desktop_config.json
-
Add the MCP server configuration:
{ "mcpServers": { "homeassistant-mcp": { "command": "npx", "args": ["homeassistant-mcp"], "env": { "HASS_TOKEN": "your_home_assistant_token_here", "HASS_HOST": "http://your_home_assistant_host:8123" } } } }
-
Restart Claude Desktop.
-
In Claude, you can now use the Home Assistant MCP tools.
JSON-RPC 2.0 Message Format
Usage
Using NPX (Easiest)
The simplest way to use the Home Assistant MCP server is through NPX:
# Start the server in stdio mode
npx homeassistant-mcp
This will automatically:
- Start the server in stdio mode
- Output JSON-RPC messages to stdout
- Send log messages to stderr
- Create a logs directory if it doesn't exist
You can redirect stderr to hide logs and only see the JSON-RPC output:
npx homeassistant-mcp 2>/dev/null
Manual Installation
If you prefer to install the package globally or locally:
# Install globally
npm install -g homeassistant-mcp
# Then run
homeassistant-mcp
Or install locally:
# Install locally
npm install homeassistant-mcp
# Then run using npx
npx homeassistant-mcp
Advanced Usage
相关推荐
Evaluator for marketplace product descriptions, checks for relevancy and keyword stuffing.
This GPT assists in finding a top-rated business CPA - local or virtual. We account for their qualifications, experience, testimonials and reviews. Business operators provide a short description of your business, services wanted, and city or state.
I find academic articles and books for research and literature reviews.
Confidential guide on numerology and astrology, based of GG33 Public information
Advanced software engineer GPT that excels through nailing the basics.
Converts Figma frames into front-end code for various mobile frameworks.
Take an adjectivised noun, and create images making it progressively more adjective!
Discover the most comprehensive and up-to-date collection of MCP servers in the market. This repository serves as a centralized hub, offering an extensive catalog of open-source and proprietary MCP servers, complete with features, documentation links, and contributors.
Micropython I2C-based manipulation of the MCP series GPIO expander, derived from Adafruit_MCP230xx
Mirror ofhttps://github.com/agentience/practices_mcp_server
A unified API gateway for integrating multiple etherscan-like blockchain explorer APIs with Model Context Protocol (MCP) support for AI assistants.
The all-in-one Desktop & Docker AI application with built-in RAG, AI agents, No-code agent builder, MCP compatibility, and more.
Reviews
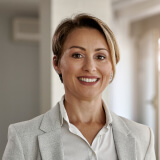
user_e73hPUr0
Mcp Unity Manager Server by bsmi021 is an outstanding product for any MCP application user. The server's seamless integration and robust management capabilities enhance productivity and streamline operations. With an intuitive interface and comprehensive support, it's a must-have tool for managing various MCP tasks efficiently. Highly recommended!