PDF scientific paper translation with preserved formats - 基于 AI 完整保留排版的 PDF 文档全文双语翻译,支持 Google/DeepL/Ollama/OpenAI 等服务,提供 CLI/GUI/MCP/Docker/Zotero
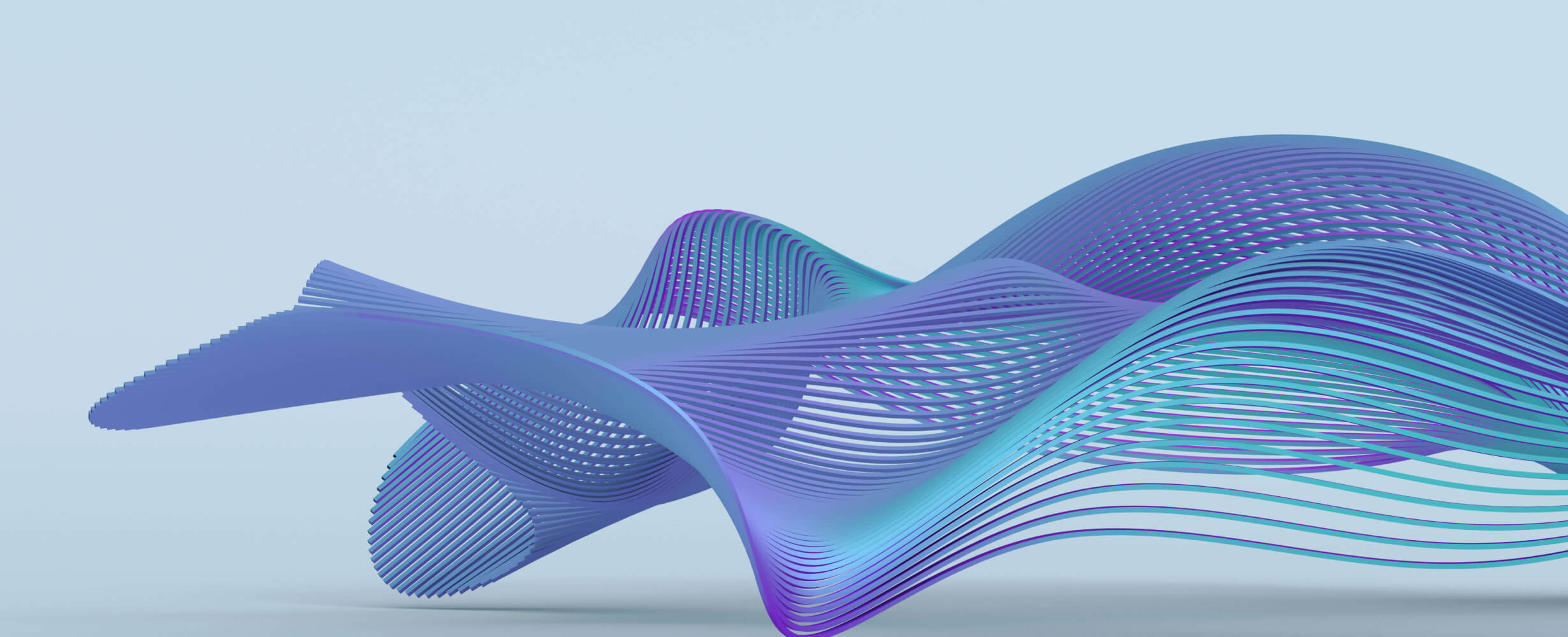
Cognisphere
Cognisphere ADK is an AI agent development framework built on Google's Agent Development Kit (ADK)
3 years
Works with Finder
5
Github Watches
0
Github Forks
5
Github Stars
Cognisphere ADK: A Cognitive Architecture Framework
Current Version: https://github.com/IhateCreatingUserNames2/Cognisphere/tree/main/cognisphere_adk_1.1
How to Edit, Workflows, More technical Report: https://github.com/IhateCreatingUserNames2/Cognisphere/blob/main/cognisphere_how_to_edit.md
Cognisphere is an advanced cognitive architecture built with Google's Agent Development Kit (ADK). It features sophisticated memory, narrative, and identity capabilities, allowing for context-aware conversations with persistent state across sessions.
🧠 Overview
Cognisphere implements a multi-agent architecture with specialized components:
- Orchestrator Agent: Coordinates all sub-agents and handles user interaction
- Memory Agent: Stores and retrieves memories of different types
- Narrative Agent: Creates and manages narrative threads for experiences
- Identity Agent: Manages different identities/personas the system can adopt
- MCP Agent: Integrates with Model Context Protocol servers for extended capabilities
- AIRA Network: Enables agent-to-agent communication
🔄 Chat History and Session Management
Cognisphere maintains conversation history across sessions through a sophisticated system of session management, state persistence, and memory retrieval.
How Session Management Works
-
Session Service:
- The application uses
DatabaseSessionService
for persistent storage of sessions - Each session has a unique ID, and is associated with a user ID
- Sessions store both the conversation history (events) and state variables
- The application uses
-
State Management:
- Session state is used to track context across conversations
- Different state prefixes have different scopes:
- No prefix: Session-specific state (current conversation only)
-
user:
prefix: User-specific state (shared across all sessions for that user) -
app:
prefix: Application-wide state (shared across all users) -
temp:
prefix: Temporary state (discarded after processing)
-
Memory Processing:
- After each conversation, the
process_session_memory
function extracts key moments - These are analyzed for emotional content and significance
- Important memories are stored in the database with embeddings for later retrieval
- After each conversation, the
-
Session Continuity:
- When a new conversation starts, the system can recall relevant memories from previous sessions
- This is done by the
_process_message_async
function which searches previous sessions for context - Memories are retrieved based on relevance to the current query
User Interface for Sessions
The UI provides features for managing sessions:
- View all conversation sessions
- Switch between existing sessions
- Create new conversations
- Each session maintains its own state and history
🧩 Core Components
Services
-
Database Service (
DatabaseService
): Persistent storage for memories and narratives -
Embedding Service (
EmbeddingService
): Generates vector embeddings for semantic search -
Session Service (
DatabaseSessionService
): Manages conversation sessions and state -
Identity Store (
IdentityStore
): Handles identity creation and persistence
Data Models
- Memory: Stores information with emotion and relevance scoring
- Narrative Thread: Organizes experiences into coherent storylines
- Identity: Represents different personas the system can embody
Integration Capabilities
-
MCP Integration: Connects with Model Context Protocol servers for extended tools
- how to add MCP Servers, take for example the Claude.Json configuration : NPX { "mcpServers": { "memory": { "command": "npx", "args": [ "-y", "@modelcontextprotocol/server-memory" ] } } } In the UI, add mcpServers, NPC, and -y,@modelcontextprotoco/server-memory ....
Refresh the Page to reload MCPServers. Sometimes it Fails. ***** It Only INSTALLS NPX SERVERS FOR NOW.
-
You can add more commands thru .../mcpIntegration/server_installer.py , mcp_shared_environment.py ....
-
read https://github.com/IhateCreatingUserNames2/Cognisphere/blob/main/mcp.md to know more
-
-
AIRA Network: Enables discovery and communication with other AI agents
🛠️ API Endpoints
Session Management
-
/api/sessions/all
: Get all sessions for a user -
/api/sessions/create
: Create a new session -
/api/sessions
: Get recent sessions for a user -
/api/session/messages
: Get messages for a specific session
Chat
-
/api/chat
: Process a user message through the orchestrator
Memory & Narrative
-
/api/memories
: Get recalled memories for the current context -
/api/narratives
: Get active narrative threads
Identity
-
/api/identities
: Get available identities -
/api/identities/switch
: Switch to a different identity
System
-
/api/status
: Get system status information
Integrations
-
/api/mcp/*
: MCP server management endpoints -
/api/aira/*
: AIRA network endpoints
📂 Code Structure
Cognisphere follows a modular architecture:
-
agents/
: Agent implementations (orchestrator, memory, narrative, identity) -
data_models/
: Data structures for memory, narrative, and identity -
services/
: Core services (database, embedding, etc.) -
web/
: Web routes for API endpoints -
tools/
: Tool implementations for agents -
callbacks/
: Callback functions for agent behavior -
mcpIntegration/
: Model Context Protocol integration -
a2a/
: Agent-to-agent communication
🔧 Session Management Implementation Details
The session management system has these key components:
-
Session Initialization:
def ensure_session(user_id: str, session_id: str) -> Session: """ Ensure a session exists and is initialized with service objects. """ session = session_service.get_session( app_name=app_name, user_id=user_id, session_id=session_id ) if not session: # Create a new session with initialized state initial_state = { "user_preference_temperature_unit": "Celsius" } session = session_service.create_session( app_name=app_name, user_id=user_id, session_id=session_id, state=initial_state ) # Ensure identity catalog is loaded into session state initialize_identity_state(session) return session
-
Message Processing with Context:
async def _process_message_async(user_id: str, session_id: str, message: str): # Retrieve previous session's context previous_sessions = session_service.list_sessions( app_name=app_name, user_id=user_id ) # Get memories from previous sessions to inject context for prev_session in sorted_sessions[:3]: # Limit to last 3 sessions try: memories_result = recall_memories( tool_context=ToolContext( state={}, agent_name="memory_agent" ), query=message, limit=3, identity_filter=None, include_all_identities=False ) # Check if memories_result is a valid dictionary with memories if isinstance(memories_result, dict) and "memories" in memories_result: context_memories.extend(memories_result.get("memories", [])) except Exception as memory_error: print(f"Error recalling memories for session {prev_session.id}: {memory_error}") continue
-
Frontend Session Management:
// Switch to a different session async function switchToSession(sessionId) { console.log(`Switching to session: ${sessionId}`); if (sessionId === currentSessionId) { console.log('Already on this session'); return; } try { // Update the current session ID currentSessionId = sessionId; // Save to localStorage localStorage.setItem('currentSessionId', currentSessionId); // Load messages for this session await loadSessionMessages(sessionId); // Update UI to show which session is active document.querySelectorAll('.session-item').forEach(item => { if (item.dataset.id === sessionId) { item.classList.add('active'); } else { item.classList.remove('active'); } }); // Also refresh related data for this session updateMemories(); updateNarrativeThreads(); console.log(`Successfully switched to session: ${sessionId}`); } catch (error) { console.error('Error switching sessions:', error); addMessageToChat('assistant', `Error loading session: ${error.message}`); } }
🔄 Memory Processing
After each conversation, Cognisphere extracts important memories:
def process_session_memory(session, identity_id):
"""
Transform session into meaningful memories by analyzing emotional content,
extracting significant moments, and connecting them to identities.
"""
# Extract user messages
user_messages = []
for event in session.events:
if event.author == "user" and event.content and event.content.parts:
text = event.content.parts[0].text
if text:
user_messages.append(text)
# Analyze emotional content
combined_text = " ".join(user_messages)
emotion_data = analyze_emotion(combined_text)
# Extract key moments (most emotionally significant or newest)
key_moments = []
# Always include the most recent message
if user_messages:
key_moments.append({"content": user_messages[-1], "importance": 1.0})
# Include high-emotion messages
if len(user_messages) > 1:
for msg in user_messages[:-1]:
msg_emotion = analyze_emotion(msg)
if msg_emotion["score"] > 0.7:
key_moments.append({"content": msg, "importance": msg_emotion["score"]})
# Create memories for each key moment
for moment in key_moments:
# Determine memory type based on content and emotion
if emotion_data["emotion_type"] in ["joy", "excitement", "curiosity"]:
memory_type = "emotional"
elif emotion_data["score"] > 0.8:
memory_type = "flashbulb" # Highly significant memories
else:
memory_type = "explicit" # Regular factual memories
# Store memory in database with embedding
memory = Memory(
content=moment["content"],
memory_type=memory_type,
emotion_data=emotion_data,
source="session",
identity_id=identity_id,
source_identity=identity_id
)
embedding = embedding_service.encode(moment["content"])
if embedding:
db_service.add_memory(memory, embedding)
🚀 Getting Started
-
Install dependencies:
pip install google-adk litellm google-genai sentence-transformers flask openai
-
Run the application:
python app.py
-
Access the UI at
http://localhost:8095
🔧 Troubleshooting Session Management
If chat history is not persisting between sessions:
- Check that
DatabaseSessionService
is correctly initialized - Verify that session IDs are being properly passed between requests
- Ensure the localStorage mechanism in the frontend is working correctly
- Check backend logs for any errors during session retrieval or storage
- Verify that the
/api/sessions/all
endpoint is correctly identifying user sessions - Make sure session switching in the UI is properly updating session history
📝 Future Improvements
- Enhance memory processing for better context retrieval ( The Memory Agent needs to be Linked Correctly with the Narrative Agent )
- Implement more sophisticated narrative creation
- Enhance frontend session management with search capabilities
- Add metadata display for memories and narrative connections
- Better UI
- Attachments
- Artifacts
Added Stuff
- 04.22 - OpenRouter API Key On the UI
📝 Know Issues:
- Async issues
- Deprecated libs
- MCP sometimes fails
- AiraHub Needs to be Updated for auth or use the old AiraHub code.
- Invalid Date in Sessions
相关推荐
🔥 1Panel provides an intuitive web interface and MCP Server to manage websites, files, containers, databases, and LLMs on a Linux server.
Easily create LLM tools and agents using plain Bash/JavaScript/Python functions.
😎简单易用、🧩丰富生态 - 大模型原生即时通信机器人平台 | 适配 QQ / 微信(企业微信、个人微信)/ 飞书 / 钉钉 / Discord / Telegram / Slack 等平台 | 支持 ChatGPT、DeepSeek、Dify、Claude、Gemini、xAI、PPIO、Ollama、LM Studio、阿里云百炼、火山方舟、SiliconFlow、Qwen、Moonshot、ChatGLM、SillyTraven、MCP 等 LLM 的机器人 / Agent | LLM-based instant messaging bots platform, supports Discord, Telegram, WeChat, Lark, DingTalk, QQ, Slack
Artifact2MCP Generator allows generation of MCP server automatically & dynamically given smart contract's compiled artifact (chain‑agnostic)
Reviews
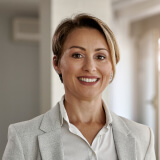
user_M7CwLVpK
Cognisphere by IhateCreatingUserNames2 is an incredibly versatile MCP app that has significantly improved my productivity. Its intuitive interface paired with powerful features makes managing my tasks a breeze. Whether you're a professional or a student, Cognisphere caters to all your organizational needs seamlessly. Highly recommend giving it a try!
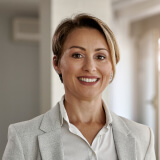
user_oel9XAJ2
Cognisphere by IhateCreatingUserNames2 is an incredibly versatile MCP application. It offers a seamless and user-friendly experience that's perfect for anyone looking to enhance their productivity. The interface is intuitive, and the features are robust, making it a must-have tool for any tech-savvy user. Highly recommend giving it a try!
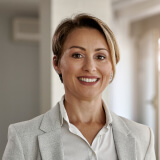
user_jYMkj7ZT
Cognisphere is an exceptional product by IhateCreatingUserNames2. Its user-friendly interface and intuitive design make it a pleasure to use. As a loyal MCP application user, I found Cognisphere to be a powerful tool that greatly enhances productivity. Highly recommended for anyone looking to optimize their workflow!
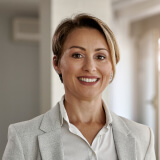
user_w84sMZgL
Cognisphere is an incredible application developed by IhateCreatingUserNames2. As a dedicated mcp user, I found the seamless integration and user-friendly interface to be particularly impressive. The developers have clearly put a lot of thought into creating a product that enhances productivity and user experience. Highly recommend trying Cognisphere!
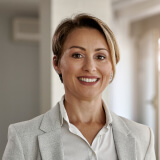
user_GOyMAwBX
Cognisphere is an exceptional tool that offers a streamlined and efficient user experience. Created by IhateCreatingUserNames2, this application is a game-changer for anyone looking to enhance their productivity. The intuitive design and smooth navigation make it a pleasure to use, and it truly simplifies complex tasks. Highly recommend!
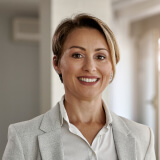
user_t0Wm0Tt8
Cognisphere is an incredible tool for managing and organizing information efficiently. As an MCP application user, I truly appreciate its intuitive design and seamless integration. It has significantly boosted my productivity and streamlined my workflow. Kudos to IhateCreatingUserNames2 for creating such a fantastic product! Highly recommended for anyone looking to enhance their organizational skills and efficiency.
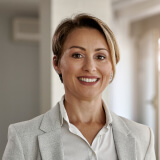
user_59JwHD8N
Cognisphere by IhateCreatingUserNames2 is an exceptional MCP application that seamlessly integrates innovative features and user-friendly design. The product's intuitive interface ensures a smooth user experience, making it accessible to all levels of expertise. Highly recommend checking it out!
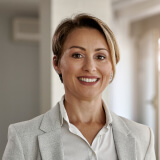
user_lzz4HGto
Cognisphere is an outstanding application by IhateCreatingUserNames2 that has significantly boosted my productivity. It offers a seamless user experience and provides a variety of powerful tools to streamline workflow. I highly recommend it to anyone looking to enhance their efficiency.
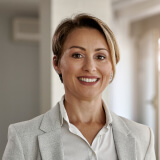
user_qEs34VVi
Cognisphere is an exceptional tool by IhateCreatingUserNames2 that excels in simplifying complex tasks. The user-friendly interface and seamless navigation make it a joy to use. It's a must-have for anyone looking to enhance productivity and efficiency. Highly recommend!
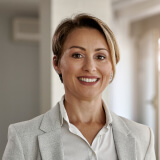
user_h8X2U78D
Cognisphere is an incredible application that has truly transformed my workflow. Developed by IhateCreatingUserNames2, it is both user-friendly and robust. The seamless integration and intuitive interface make managing tasks a breeze. I highly recommend Cognisphere to anyone looking to enhance their productivity and streamline their daily tasks. This app is a game-changer in every sense!