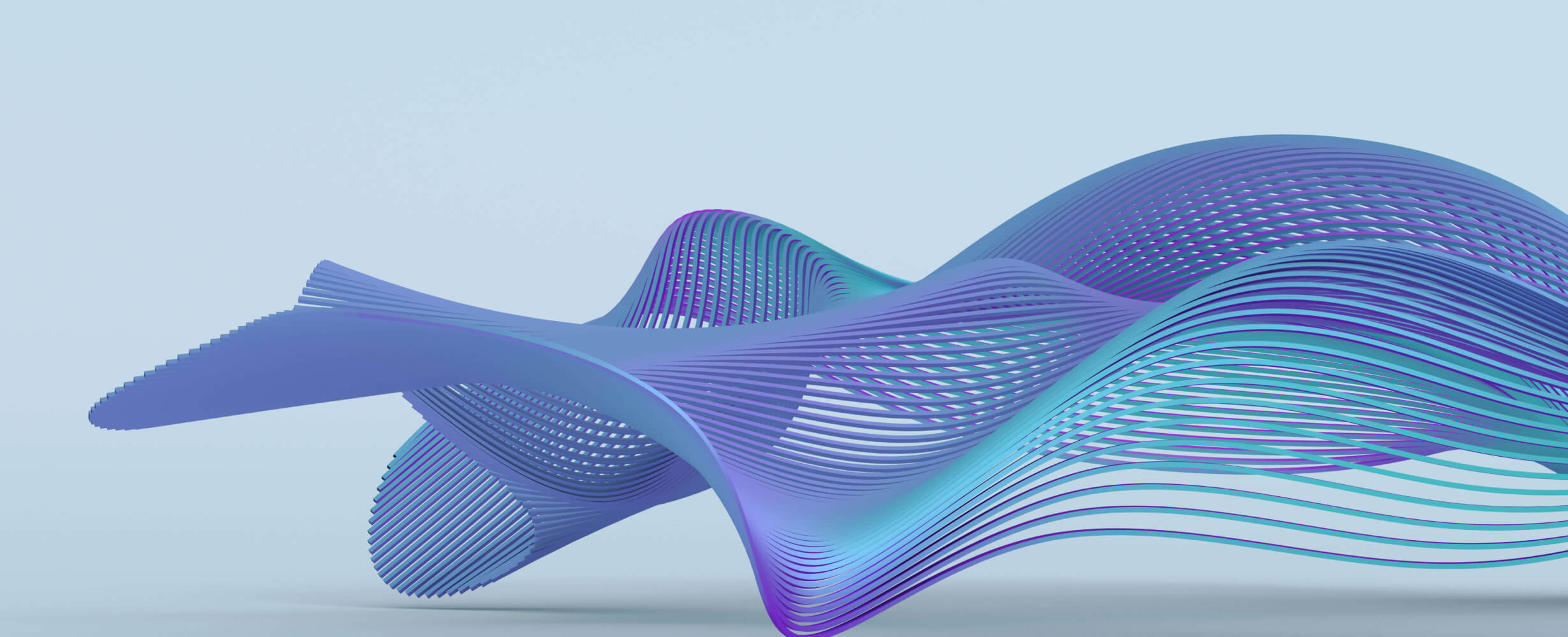
zaptomcp
A zap to build MCP Servers
3 years
Works with Finder
0
Github Watches
0
Github Forks
0
Github Stars
ZapToMCP
⚠️ Work in Progress
Warning: This repository is currently under active development. The API and implementation details are subject to change. Use at your own risk.
ZapToMCP is a monorepo containing tools and libraries for building Model Context Protocol (MCP) servers and clients. It provides a streamlined way to create, deploy, and manage MCP-compatible services.
Overview
The Model Context Protocol (MCP) is an open standard for communication between AI assistants and tools. ZapToMCP simplifies the process of building MCP-compatible servers and clients, allowing developers to focus on creating powerful AI experiences rather than implementing protocol details.
Repository Structure
This monorepo contains the following packages:
-
@zaptomcp/sdk
: A TypeScript implementation of the Model Context Protocol -
@zaptomcp/server
: A higher-level abstraction for creating MCP servers - More packages coming soon...
Getting Started
Prerequisites
- Node.js >= 20
- pnpm >= 10.8.1
Installation
Clone the repository:
git clone https://github.com/hoghweed/zaptomcp.git
cd zaptomcp
Install dependencies:
pnpm install
Installation
# Install dependencies
pnpm install
# Bootstrap the monorepo
pnpm bootstrap
Development
Available Scripts
-
pnpm dev
: Start development mode for all packages -
pnpm build
: Build all packages -
pnpm clean
: Clean build artifacts -
pnpm lint
: Run linting across all packages -
pnpm format
: Format code across all packages -
pnpm typecheck
: Run type checking across all packages -
pnpm commit
: Create a commit using conventional commit format
Code Quality
This project uses:
- Biome for linting and formatting
- TypeScript for type checking
- Husky for git hooks
- Commitlint for commit message validation
@zaptomcp/sdk
The SDK package provides a TypeScript implementation for interacting with the Model Context Protocol (MCP).
Features
- Multiple transport implementations:
- In-memory
- SSE (Server-Sent Events)
- Stdio
- Streamable HTTP
- Type-safe API
- Built with Fastify for high performance
- Support for both ESM and CommonJS
Installation
pnpm add @zaptomcp/sdk
Usage
Usage Creating an MCP Server
import { McpServer } from '@zaptomcp/sdk';
// Create a server with basic information
const server = new McpServer(
{
name: "my-mcp-server",
version: "1.0.0"
},
{
capabilities: {
// Define your server capabilities
sampling: {},
prompts: {},
resources: {},
tools: {},
logging: {}
},
instructions: "This is an MCP-compatible server"
}
);
// Connect the server to a transport
import { FastifyTransport } from '@zaptomcp/sdk/transports';
const transport = new FastifyTransport();
await server.connect(transport);
// Start listening for requests
await transport.listen(3000);
console.log("MCP server running on port 3000");
Creating an MCP Client
import { Client } from '@zaptomcp/sdk';
// Create a client with basic information
const client = new Client(
{
name: "my-mcp-client",
version: "1.0.0"
},
{
capabilities: {
// Define your client capabilities
sampling: {}
}
}
);
// Connect to an MCP server
import { HTTPClientTransport } from '@zaptomcp/sdk/transports';
const transport = new HTTPClientTransport('http://localhost:3000');
await client.connect(transport);
// Read a resource
const resource = await client.readResource({
uri: "test://example/resource"
});
console.log(resource);
API Documentation
The SDK provides the following main exports:
-
Server
: The main server class -
transports
: Various transport implementations-
in-memory
: In-memory transport -
sse
: Server-Sent Events transport -
stdio
: Standard I/O transport -
streamable-http
: HTTP transport with streaming support
-
Development
To work on the SDK:
# Navigate to the SDK package
cd packages/sdk
# Install dependencies
pnpm install
# Start development mode
pnpm dev
# Run tests
pnpm test
# Build the package
pnpm build
Contributing
Contributions are welcome! Please feel free to submit a Pull Request.
- Fork the repository
- Create your feature branch ( git checkout -b feature/amazing-feature )
- Commit your changes using conventional commits ( pnpm commit )
- Push to the branch ( git push origin feature/amazing-feature )
- Open a Pull Request
License
This project is licensed under the MIT License - see the LICENSE file for details.
Acknowledgements
- ZapToMCP is an inspired porting of FastMCP
- Model Context Protocol Typescript SDK - The open standard this project implements
- FastMCP is inspired by the Python implementation by Jonathan Lowin.
- Parts of codebase were adopted from LiteMCP.
- Parts of codebase were adopted from Model Context protocolでSSEをやってみる.
相关推荐
🔥 1Panel provides an intuitive web interface and MCP Server to manage websites, files, containers, databases, and LLMs on a Linux server.
PDF scientific paper translation with preserved formats - 基于 AI 完整保留排版的 PDF 文档全文双语翻译,支持 Google/DeepL/Ollama/OpenAI 等服务,提供 CLI/GUI/MCP/Docker/Zotero
Easily create LLM tools and agents using plain Bash/JavaScript/Python functions.
Artifact2MCP Generator allows generation of MCP server automatically & dynamically given smart contract's compiled artifact (chain‑agnostic)
Reviews
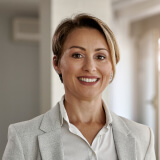
user_jc21lJ7k
I've been using zaptomcp for a while now, and it's been fantastic! Developed by hoghweed, this application truly stands out. The interface is intuitive, and the features are incredibly useful for managing my tasks efficiently. Highly recommended for anyone looking for a reliable MCP application.
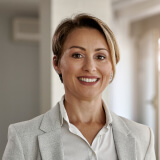
user_qxVkpwkB
As a dedicated user of zaptomcp, I must say this tool by hoghweed has significantly streamlined my processes. Its intuitive design and robust functionality have made complex tasks much simpler. I highly recommend it to anyone looking for an efficient and reliable solution.