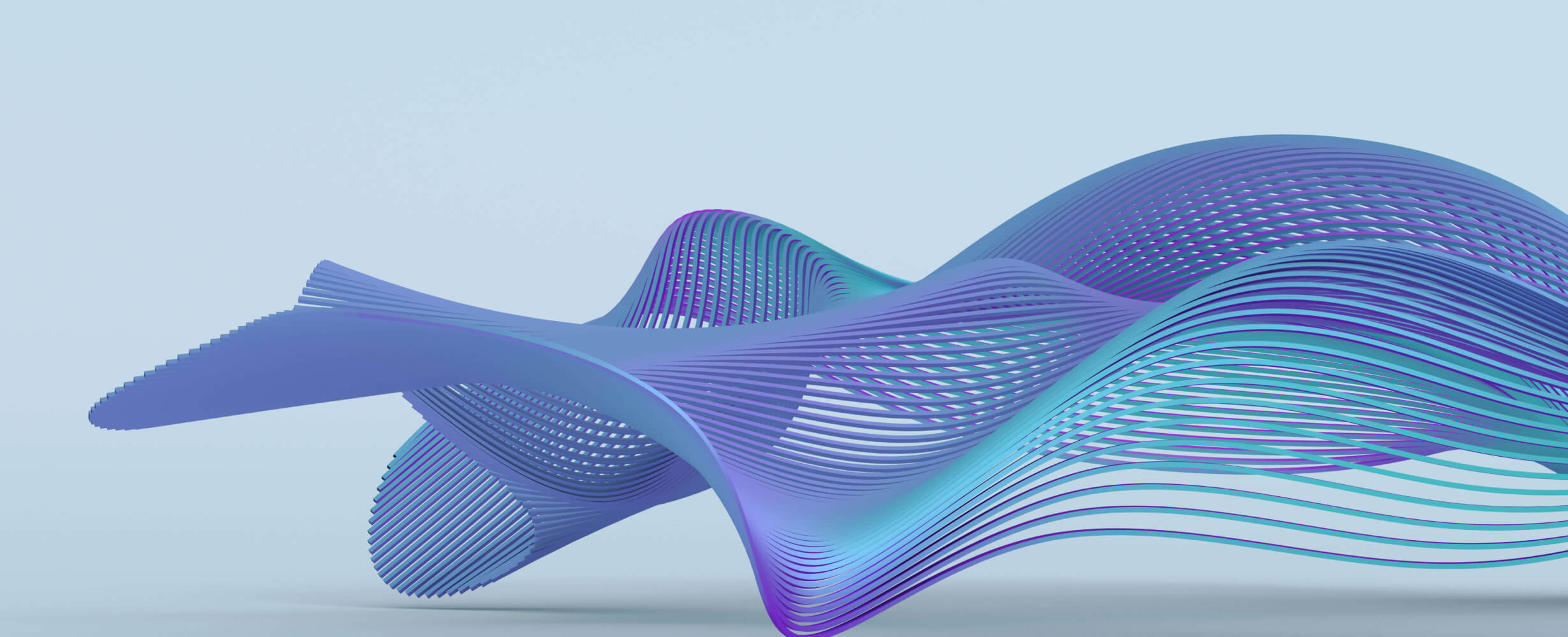
master_mcp_server
AI Master Control Program (MCP) Server - Enabling AI models to interact with your system
1
Github Watches
0
Github Forks
0
Github Stars
AI Master Control Program (MCP) Server
The AI MCP Server enables AI models, including locally hosted models with Ollama and Claude Desktop, to interact with your computer system. It acts as a bridge that allows AI models to:
- Execute system commands
- Create, read, update, and delete files
- Control other programs
- Communicate with each other
Architecture
The system consists of:
- MCP Server: Central server that processes requests from AI models
- Client Library: Enables easy integration with AI models
- Model Connectors: Interfaces with various AI model backends (Ollama, Claude Desktop, etc.)
- Task Execution Engine: Performs system operations and program control
Installation
Prerequisites
- Python 3.8+
- Ollama (optional, for local model hosting)
- Claude Desktop (recommended default model)
Automated Installation
For quick and easy installation, use the provided installation script:
# Clone the repository
git clone https://github.com/GrizzFuOnYou/master_mcp_server.git
cd master_mcp_server
# Run the installation script
python install.py
The installation script will:
- Verify Python version compatibility
- Install all dependencies
- Create a directory structure
- Configure environment variables
- Create platform-specific startup scripts
- Set up Claude Desktop as the default AI model
Manual Setup
If you prefer manual installation:
-
Clone the repository:
git clone https://github.com/GrizzFuOnYou/master_mcp_server.git cd master_mcp_server
-
Install dependencies:
pip install -r requirements.txt
-
Configure environment variables:
cp .env.example .env # Edit .env with your preferred settings
Usage
Starting the Server
Using Startup Script (Recommended)
After installation:
-
Windows: Run
start_mcp_server.bat
-
Linux/Mac: Run
./start_mcp_server.sh
Manual Start
Run the MCP server:
python startup.py
By default, the server will listen on 0.0.0.0:8000
.
Connecting AI Models
Claude Desktop (Default)
Claude Desktop is configured as the default model. To use it:
- Make sure Claude Desktop is running on your system
- The server will automatically attempt to connect on startup
- Claude Desktop should be available at the default location:
http://localhost:5000/api
If you need to manually connect:
from mcp_client import MCPClient
# Initialize client
client = MCPClient("http://localhost:8000", "your-secret-api-key")
# Connect to Claude Desktop
result = client.connect_model("claude-desktop", "claude", {"api_url": "http://localhost:5000/api"})
print(f"Connection result: {result}")
Claude Desktop Connection JSON
If you need to manually configure Claude Desktop integration, use the following JSON configuration:
{
"model_id": "claude-desktop",
"model_type": "claude",
"config": {
"api_url": "http://localhost:5000/api",
"temperature": 0.7,
"max_tokens": 1000
}
}
Ollama Models
To connect to an Ollama model:
from mcp_client import MCPClient
# Initialize client
client = MCPClient("http://localhost:8000", "your-secret-api-key")
# Connect to an Ollama model
result = client.connect_model("llama2", "ollama", {"host": "http://localhost:11434"})
print(f"Connection result: {result}")
Executing System Operations
Once connected, AI models can perform various system operations:
# Execute a command
result = client.execute_system_command("claude-desktop", "echo", ["Hello, World!"])
# Write a file
result = client.write_file("claude-desktop", "test.txt", "This is a test file created by Claude!")
# Read a file
result = client.read_file("claude-desktop", "test.txt")
# Start a program
result = client.start_program("claude-desktop", "notepad.exe")
# Stop a program
result = client.stop_program("claude-desktop", pid)
# Query the AI model
result = client.query_model("claude-desktop", "claude-desktop", "What is the capital of France?")
API Reference
Server Endpoints
Endpoint | Method | Description |
---|---|---|
/connect_model |
POST | Connect to an AI model |
/disconnect_model/{model_id} |
POST | Disconnect from an AI model |
/list_models |
GET | List all connected models |
/execute_task |
POST | Execute a task requested by an AI model |
/task_status/{task_id} |
GET | Get the status of a task |
Client Methods
Method | Description |
---|---|
connect_model(model_id, model_type, config) |
Connect to an AI model |
disconnect_model(model_id) |
Disconnect from an AI model |
list_models() |
List all connected models |
execute_system_command(model_id, command, args, working_dir, timeout) |
Execute a system command |
execute_file_operation(model_id, operation, path, content) |
Execute a file operation |
control_program(model_id, action, program_path, args, pid) |
Control a program |
query_model(model_id, target_model, prompt) |
Query an AI model |
Model Configuration
Claude Desktop Configuration
To connect to Claude Desktop, use the following configuration:
{
"api_url": "http://localhost:5000/api",
"temperature": 0.7,
"max_tokens": 1000
}
Ollama Configuration
To connect to an Ollama model, use the following configuration:
{
"host": "http://localhost:11434"
}
Security Considerations
IMPORTANT: This server grants AI models significant access to your system. Use with caution.
Security measures implemented:
- API key authentication
- Logging of all operations
- Configurable permissions (coming soon)
- Rate limiting (coming soon)
Troubleshooting
Claude Desktop Connection Issues
If you encounter issues connecting to Claude Desktop:
- Ensure Claude Desktop is running
- Verify the API URL (default:
http://localhost:5000/api
) - Check the logs for specific error messages
- Restart Claude Desktop and try again
Ollama Connection Issues
If you encounter issues connecting to Ollama:
- Ensure Ollama is running (
ollama serve
) - Verify the model exists (
ollama list
) - Check the API URL (default:
http://localhost:11434
) - Try pulling the model again (
ollama pull modelname
)
Extension Points
The MCP server can be extended to support:
- Additional AI model backends
- More sophisticated program control
- GUI interaction capabilities
- Web browsing capabilities
- Network operation capabilities
License
MIT
Contributing
Contributions are welcome! Please feel free to submit a Pull Request.
相关推荐
I find academic articles and books for research and literature reviews.
Confidential guide on numerology and astrology, based of GG33 Public information
Converts Figma frames into front-end code for various mobile frameworks.
Advanced software engineer GPT that excels through nailing the basics.
Take an adjectivised noun, and create images making it progressively more adjective!
Embark on a thrilling diplomatic quest across a galaxy on the brink of war. Navigate complex politics and alien cultures to forge peace and avert catastrophe in this immersive interstellar adventure.
Emulating Dr. Jordan B. Peterson's style in providing life advice and insights.
Discover the most comprehensive and up-to-date collection of MCP servers in the market. This repository serves as a centralized hub, offering an extensive catalog of open-source and proprietary MCP servers, complete with features, documentation links, and contributors.
Micropython I2C-based manipulation of the MCP series GPIO expander, derived from Adafruit_MCP230xx
The all-in-one Desktop & Docker AI application with built-in RAG, AI agents, No-code agent builder, MCP compatibility, and more.
Bridge between Ollama and MCP servers, enabling local LLMs to use Model Context Protocol tools
Fair-code workflow automation platform with native AI capabilities. Combine visual building with custom code, self-host or cloud, 400+ integrations.
🧑🚀 全世界最好的LLM资料总结(Agent框架、辅助编程、数据处理、模型训练、模型推理、o1 模型、MCP、小语言模型、视觉语言模型) | Summary of the world's best LLM resources.
Reviews
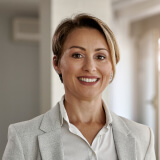
user_u0VyN3qN
I've been using master_mcp_server by GrizzFuOnYou, and it's simply outstanding. It provides seamless integration with my applications and the setup is remarkably intuitive. The detailed documentation on the GitHub link (https://github.com/GrizzFuOnYou/master_mcp_server) was very helpful, ensuring a smooth implementation. Truly a must-have for any MCP enthusiast!