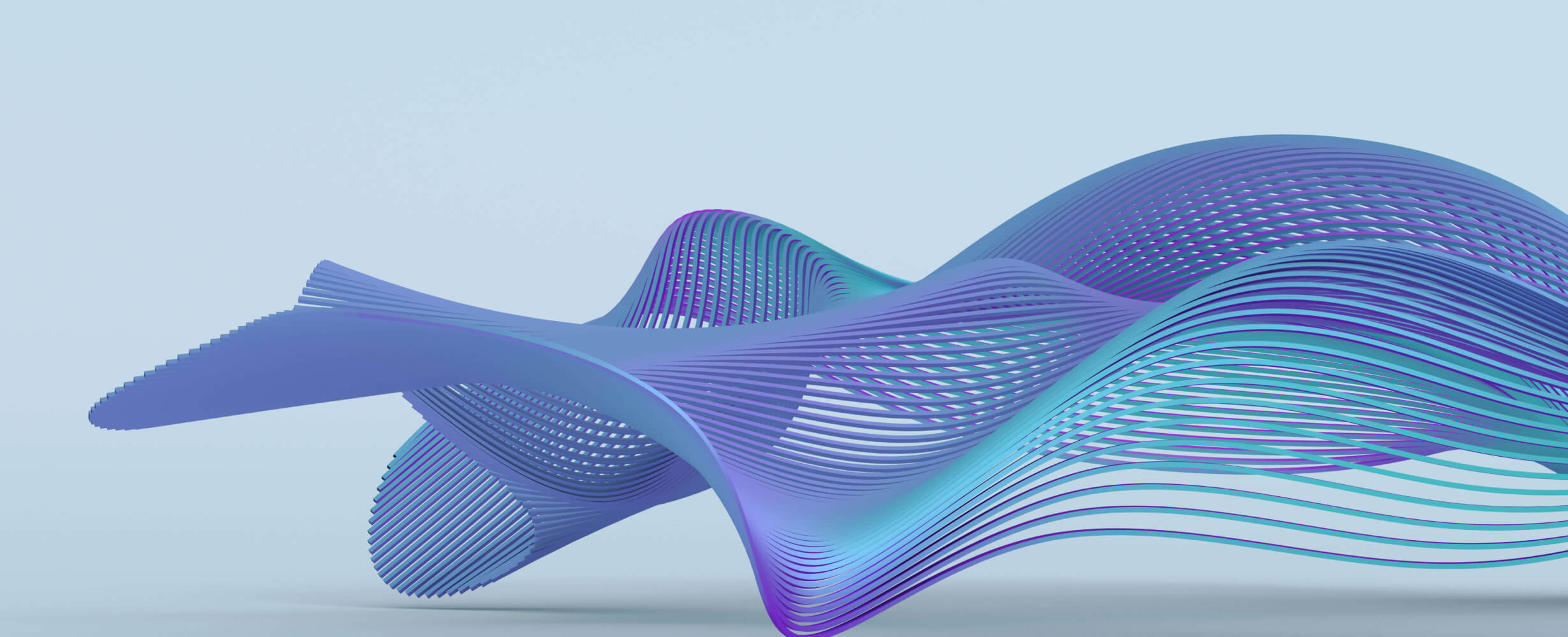
mcp-utils
Python utilities to work with MCP servers
3 years
Works with Finder
2
Github Watches
1
Github Forks
0
Github Stars
mcp-utils
A Python utility package for building Model Context Protocol (MCP) servers.
Table of Contents
- mcp-utils
Overview
mcp-utils
provides utilities and helpers for building MCP-compliant servers in Python, with a focus on synchronous implementations using Flask. This package is designed for developers who want to implement MCP servers in their existing Python applications without the complexity of asynchronous code.
Key Features
- Basic utilities for MCP server implementation
- Server-Sent Events (SSE) support
- Simple decorators for MCP endpoints
- Synchronous implementation
- HTTP protocol support
- Redis response queue
- Comprehensive Pydantic models for MCP schema
- Built-in validation and documentation
Installation
pip install mcp-utils
Requirements
- Python 3.10+
- Pydantic 2
Optional Dependencies
- Flask (for web server)
- Gunicorn (for production deployment)
- Redis (for response queue)
Usage
Basic MCP Server
Here's a simple example of creating an MCP server:
from mcp_utils.core import MCPServer
from mcp_utils.schema import GetPromptResult, Message, TextContent, CallToolResult
# Create a basic MCP server
mcp = MCPServer("example", "1.0")
@mcp.prompt()
def get_weather_prompt(city: str) -> GetPromptResult:
return GetPromptResult(
description="Weather prompt",
messages=[
Message(
role="user",
content=TextContent(
text=f"What is the weather like in {city}?",
),
)
],
)
@mcp.tool()
def get_weather(city: str) -> str:
return "sunny"
Flask with Redis Example
For production use, you can integrate the MCP server with Flask and Redis for better message handling:
from flask import Flask, Response, url_for, request
import redis
from mcp_utils.queue import RedisResponseQueue
# Setup Redis client
redis_client = redis.Redis(host="localhost", port=6379, db=0)
# Create Flask app and MCP server with Redis queue
app = Flask(__name__)
mcp = MCPServer(
"example",
"1.0",
response_queue=RedisResponseQueue(redis_client)
)
@app.route("/sse")
def sse():
session_id = mcp.generate_session_id()
messages_endpoint = url_for("message", session_id=session_id)
return Response(
mcp.sse_stream(session_id, messages_endpoint),
mimetype="text/event-stream"
)
@app.route("/message/<session_id>", methods=["POST"])
def message(session_id):
mcp.handle_message(session_id, request.get_json())
return "", 202
if __name__ == "__main__":
app.run(debug=True)
SQLAlchemy Transaction Handling Example
For production use, you can integrate the MCP server with Flask, Redis, and SQLAlchemy for better message handling and database transaction management:
from flask import Flask, request
from sqlalchemy.orm import Session
from sqlalchemy import create_engine
import redis
from mcp_utils.queue import RedisResponseQueue
# Setup Redis client
redis_client = redis.Redis(host="localhost", port=6379, db=0)
# Create engine for PostgreSQL database
engine = create_engine("postgresql://user:pass@localhost/dbname")
# Create Flask app and MCP server with Redis queue
app = Flask(__name__)
mcp = MCPServer(
"example",
"1.0",
response_queue=RedisResponseQueue(redis_client)
)
@app.route("/message/<session_id>", methods=["POST"])
def message(session_id):
with Session(engine) as session:
try:
mcp.handle_message(session_id, request.get_json())
session.commit()
return "", 202
except Exception as e:
session.rollback()
raise
if __name__ == "__main__":
app.run(debug=True)
For a more comprehensive example including logging setup and session management, check out the example Flask application in the repository.
Connecting with MCP Clients
Claude Desktop
Currently, only Claude Desktop (not claude.ai) can connect to MCP servers. As of this writing, Claude Desktop does not support MCP through SSE and only supports stdio. To connect Claude Desktop with an MCP server, you'll need to use mcp-proxy.
Configuration example for Claude Desktop:
{
"mcpServers": {
"weather": {
"command": "/Users/yourname/.local/bin/mcp-proxy",
"args": ["http://127.0.0.1:9000/sse"]
}
}
}
Installing via Smithery
To install MCP Proxy for Claude Desktop automatically via Smithery:
npx -y @smithery/cli install mcp-proxy --client claude
Installing via PyPI
The stable version of the package is available on the PyPI repository. You can install it using the following command:
# Option 1: With uv (recommended)
uv tool install mcp-proxy
# Option 2: With pipx (alternative)
pipx install mcp-proxy
Once installed, you can run the server using the mcp-proxy
command.
Contributing
Contributions are welcome! Please feel free to submit a Pull Request.
Related Projects
- MCP Python SDK - The official async Python SDK for MCP
- mcp-proxy - A proxy tool to connect Claude Desktop with MCP servers
License
MIT License
Testing with MCP Inspector
The MCP Inspector is a useful tool for testing and debugging MCP servers. It provides a web interface to inspect and test MCP server endpoints.
Installation
Install MCP Inspector using npm:
npm install -g @modelcontextprotocol/inspector
Usage
- Start your MCP server (e.g., the Flask example above)
- Run MCP Inspector:
git clone git@github.com:modelcontextprotocol/inspector.git
cd inspector
npm run build
npm start
- Open your browser and navigate to
http://127.0.0.1:6274/
- Enter your MCP server URL (e.g.,
http://localhost:9000/sse
) - Use the inspector to:
- Change transport type to SSE
- Test server connections
- Monitor SSE events
- Send test messages
- Debug responses
This tool is particularly useful during development to ensure your MCP server implementation is working correctly and complies with the protocol specification.
相关推荐
I find academic articles and books for research and literature reviews.
Confidential guide on numerology and astrology, based of GG33 Public information
Converts Figma frames into front-end code for various mobile frameworks.
Embark on a thrilling diplomatic quest across a galaxy on the brink of war. Navigate complex politics and alien cultures to forge peace and avert catastrophe in this immersive interstellar adventure.
Advanced software engineer GPT that excels through nailing the basics.
Delivers concise Python code and interprets non-English comments
💬 MaxKB is a ready-to-use AI chatbot that integrates Retrieval-Augmented Generation (RAG) pipelines, supports robust workflows, and provides advanced MCP tool-use capabilities.
Micropython I2C-based manipulation of the MCP series GPIO expander, derived from Adafruit_MCP230xx
MCP server to provide Figma layout information to AI coding agents like Cursor
The all-in-one Desktop & Docker AI application with built-in RAG, AI agents, No-code agent builder, MCP compatibility, and more.
Reviews
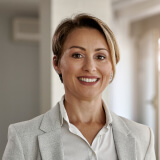
user_N9K2dEw1
I've been using mcp-utils for several projects, and it's been a game changer! The toolset provided by fulfilio is comprehensive and saves me so much time with its efficient utilities. The GitHub repository is well-documented, making it easy to integrate and use. I highly recommend this to anyone looking for reliable and versatile utilities in their workflow.