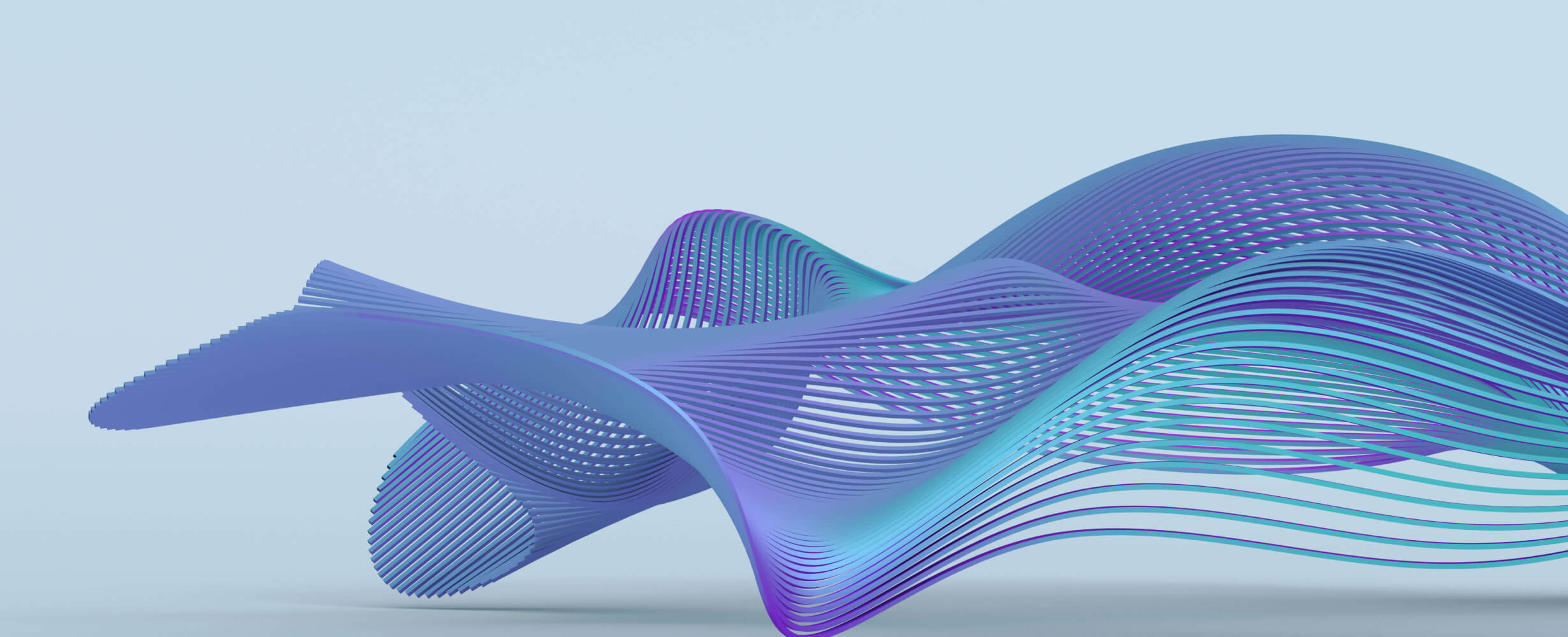
buildkite-mcp
A Model Context Protocol (MCP) server for Buildkite integration
3 years
Works with Finder
1
Github Watches
0
Github Forks
0
Github Stars
Buildkite MCP Server
A microservice for retrieving information from Buildkite via Model Context Protocol (MCP).
Setup
- Clone this repository
- Run
npm install
- Set the
BUILDKITE_ACCESS_TOKEN
environment variable - Start the server with
node index.js
Using with Cursor (MCP Integration)
To add this server to Cursor, you need to configure it in your ~/.cursor/config/mcp.json
file:
{
"mcpServers": {
"buildkite": {
"command": "npx",
"args": [
"-y",
"@drew-goddyn/buildkite-mcp"
],
"env": {
"BUILDKITE_ACCESS_TOKEN": "your-buildkite-access-token"
}
}
}
}
With this configuration:
- You don't need to install or run the server manually
- Cursor will automatically start and stop the server as needed
- Replace
your-buildkite-access-token
with your actual Buildkite API token
After updating the configuration, restart Cursor to apply the changes.
MCP Endpoints
List Organizations
POST /mcp_buildkite_list_organizations
Request Body:
{
"access_token": "optional-if-set-in-env"
}
Response:
[
{
"id": "org-id",
"name": "Organization Name",
"slug": "organization-slug"
}
]
List Pipelines
POST /mcp_buildkite_list_pipelines
Request Body:
{
"organization": "my-org",
"access_token": "optional-if-set-in-env"
}
Response:
[
{
"id": "pipeline-id",
"name": "Pipeline Name",
"slug": "pipeline-slug"
}
]
List Builds
POST /mcp_buildkite_list_builds
Request Body:
{
"organization": "my-org",
"pipeline": "my-pipeline",
"access_token": "optional-if-set-in-env",
"branch": "main",
"state": "failed",
"per_page": 10,
"page": 1
}
Response:
[
{
"id": "build-id",
"number": 123,
"state": "failed"
}
]
Get Build Details
POST /mcp_buildkite_get_build
Request Body:
{
"organization": "my-org",
"pipeline": "my-pipeline",
"build_number": 123,
"access_token": "optional-if-set-in-env"
}
Response:
{
"id": "build-id",
"number": 123,
"jobs": [
{
"id": "job-id",
"name": "Job Name",
"state": "failed"
}
]
}
List All Jobs
POST /mcp_buildkite_list_jobs
Request Body:
{
"organization": "my-org",
"pipeline": "my-pipeline",
"build_number": 123,
"access_token": "optional-if-set-in-env"
}
Response:
[
{
"id": "job-id",
"name": "Job Name",
"state": "failed"
}
]
List Failed Jobs
POST /mcp_buildkite_list_failed_jobs
Request Body:
{
"organization": "my-org",
"pipeline": "my-pipeline",
"build_number": 123,
"access_token": "optional-if-set-in-env"
}
Response:
[
{
"id": "01234567-89ab-cdef-0123-456789abcdef",
"name": "Test Job",
"state": "failed",
"web_url": "https://buildkite.com/my-org/my-pipeline/builds/123#01234567-89ab-cdef-0123-456789abcdef"
}
]
List Job Spec Failures
POST /mcp_buildkite_list_job_spec_failures
Request Body:
{
"organization": "my-org",
"pipeline": "my-pipeline",
"build_number": 123,
"job_id": "01234567-89ab-cdef-0123-456789abcdef",
"access_token": "optional-if-set-in-env"
}
Response:
[
{
"type": "RSpec",
"spec": "./spec/path/to/file_spec.rb:123",
"message": "Expected result to be X but got Y"
}
]
Get Job Log
POST /mcp_buildkite_get_job_log
Request Body:
{
"organization": "my-org",
"pipeline": "my-pipeline",
"build_number": 123,
"job_id": "01234567-89ab-cdef-0123-456789abcdef",
"access_token": "optional-if-set-in-env",
"limit": 100 // optional, defaults to all content
}
Response:
{
"content": "...log content...",
"size": 12345,
"format": "raw"
}
List Failed Specs from Build URL
POST /mcp_buildkite_list_failed_specs
Request Body:
{
"build_url": "https://buildkite.com/my-org/my-pipeline/builds/123",
"access_token": "optional-if-set-in-env"
}
Response:
{
"build_url": "https://buildkite.com/my-org/my-pipeline/builds/123",
"failed_job_count": 2,
"jobs": [
{
"id": "01234567-89ab-cdef-0123-456789abcdef",
"name": "Test Job 1",
"web_url": "https://buildkite.com/my-org/my-pipeline/builds/123#01234567-89ab-cdef-0123-456789abcdef",
"failures": [
{
"spec": "./spec/path/to/file_spec.rb:123",
"message": "Expected result to be X but got Y"
}
]
},
{
"id": "fedcba98-7654-3210-fedc-ba9876543210",
"name": "Test Job 2",
"web_url": "https://buildkite.com/my-org/my-pipeline/builds/123#fedcba98-7654-3210-fedc-ba9876543210",
"failures": []
}
],
"failures": [
{
"job_id": "01234567-89ab-cdef-0123-456789abcdef",
"job_name": "Test Job 1",
"job_url": "https://buildkite.com/my-org/my-pipeline/builds/123#01234567-89ab-cdef-0123-456789abcdef",
"spec": "./spec/path/to/file_spec.rb:123",
"message": "Expected result to be X but got Y"
}
]
}
Retry Job
POST /mcp_buildkite_retry_job
Request Body:
{
"organization": "my-org",
"pipeline": "my-pipeline",
"build_number": 123,
"job_id": "01234567-89ab-cdef-0123-456789abcdef",
"access_token": "optional-if-set-in-env"
}
Response:
{
"id": "job-id",
"state": "scheduled"
}
List Pipeline Build Failures
POST /mcp_buildkite_list_pipeline_build_failures
Request Body:
{
"organization": "my-org",
"pipeline": "my-pipeline",
"state": "finished",
"per_page": 10,
"page": 1,
"access_token": "optional-if-set-in-env"
}
Response:
[
{
"build_number": 123,
"build_url": "https://buildkite.com/my-org/my-pipeline/builds/123",
"failed_jobs": [
{
"name": "Test Job",
"url": "https://buildkite.com/my-org/my-pipeline/builds/123#01234567-89ab-cdef-0123-456789abcdef"
}
]
}
]
Environment Variables
-
BUILDKITE_ACCESS_TOKEN
: Your Buildkite API token with read access -
PORT
: (Optional) Port to run the server on (default: 63330)
Error Handling
The server returns appropriate HTTP status codes and error messages when something goes wrong:
-
400
: Bad Request - Missing or invalid parameters -
401
: Unauthorized - Invalid or missing access token -
404
: Not Found - Resource not found -
500
: Internal Server Error - Server-side error
相关推荐
I find academic articles and books for research and literature reviews.
Converts Figma frames into front-end code for various mobile frameworks.
Confidential guide on numerology and astrology, based of GG33 Public information
Embark on a thrilling diplomatic quest across a galaxy on the brink of war. Navigate complex politics and alien cultures to forge peace and avert catastrophe in this immersive interstellar adventure.
Advanced software engineer GPT that excels through nailing the basics.
Delivers concise Python code and interprets non-English comments
💬 MaxKB is a ready-to-use AI chatbot that integrates Retrieval-Augmented Generation (RAG) pipelines, supports robust workflows, and provides advanced MCP tool-use capabilities.
Micropython I2C-based manipulation of the MCP series GPIO expander, derived from Adafruit_MCP230xx
MCP server to provide Figma layout information to AI coding agents like Cursor
The all-in-one Desktop & Docker AI application with built-in RAG, AI agents, No-code agent builder, MCP compatibility, and more.
Reviews
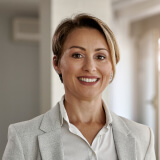
user_UqfMQ9Xm
buildkite-mcp by Drew-Goddyn is a fantastic tool for continuous integration and delivery pipelines. It offers seamless integration with Buildkite, making it super easy to manage and monitor CI/CD workflows. The setup process is straightforward, and the documentation is very helpful. Highly recommended for DevOps teams looking to streamline their CI/CD processes.