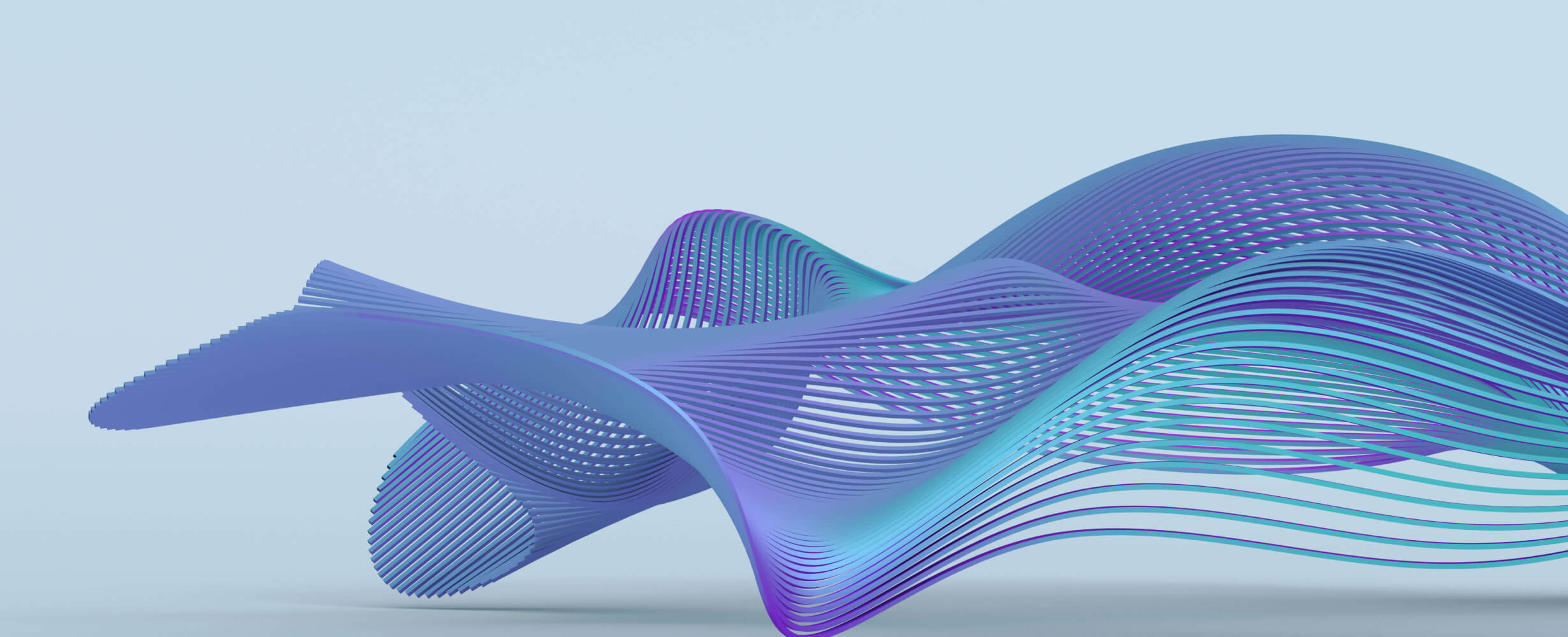
mcp-rust-sdk
Rust SDK for the Model Context Protocol (MCP)
3 years
Works with Finder
4
Github Watches
14
Github Forks
124
Github Stars
Model Context Protocol (MCP) Rust SDK
⚠️ Warning: This SDK is currently a work in progress and is not ready for production use.
A Rust implementation of the Model Context Protocol (MCP), designed for seamless communication between AI models and their runtime environments.
Features
- 🚀 Full implementation of MCP protocol specification
- 🔄 Multiple transport layers (WebSocket, stdio)
- ⚡ Async/await support using Tokio
- 🛡️ Type-safe message handling
- 🔍 Comprehensive error handling
- 📦 Zero-copy serialization/deserialization
Installation
Add this to your Cargo.toml
:
[dependencies]
mcp_rust_sdk = "0.1.0"
Quick Start
Client Example
use mcp_rust_sdk::{Client, transport::WebSocketTransport};
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
// Create a WebSocket transport
let transport = WebSocketTransport::new("ws://localhost:8080").await?;
// Create and connect the client
let client = Client::new(transport);
client.connect().await?;
// Make requests
let response = client.request("method_name", Some(params)).await?;
Ok(())
}
Server Example
use mcp_rust_sdk::{Server, transport::StdioTransport};
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error>> {
// Create a stdio transport
let (transport, _) = StdioTransport::new();
// Create and start the server
let server = Server::new(transport);
server.start().await?;
Ok(())
}
Transport Layers
The SDK supports multiple transport layers:
WebSocket Transport
- Ideal for network-based communication
- Supports both secure (WSS) and standard (WS) connections
- Built-in reconnection handling
stdio Transport
- Perfect for local process communication
- Lightweight and efficient
- Great for command-line tools and local development
Error Handling
The SDK provides comprehensive error handling through the Error
type:
use mcp_rust_sdk::Error;
match result {
Ok(value) => println!("Success: {:?}", value),
Err(Error::Protocol(code, msg)) => println!("Protocol error {}: {}", code, msg),
Err(Error::Transport(e)) => println!("Transport error: {}", e),
Err(e) => println!("Other error: {}", e),
}
License
This project is licensed under the MIT License - see the LICENSE file for details.
相关推荐
I find academic articles and books for research and literature reviews.
Confidential guide on numerology and astrology, based of GG33 Public information
Embark on a thrilling diplomatic quest across a galaxy on the brink of war. Navigate complex politics and alien cultures to forge peace and avert catastrophe in this immersive interstellar adventure.
Converts Figma frames into front-end code for various mobile frameworks.
Delivers concise Python code and interprets non-English comments
Advanced software engineer GPT that excels through nailing the basics.
💬 MaxKB is a ready-to-use AI chatbot that integrates Retrieval-Augmented Generation (RAG) pipelines, supports robust workflows, and provides advanced MCP tool-use capabilities.
Micropython I2C-based manipulation of the MCP series GPIO expander, derived from Adafruit_MCP230xx
MCP server to provide Figma layout information to AI coding agents like Cursor
The all-in-one Desktop & Docker AI application with built-in RAG, AI agents, No-code agent builder, MCP compatibility, and more.
Python code to use the MCP3008 analog to digital converter with a Raspberry Pi or BeagleBone black.
Put an end to hallucinations! GitMCP is a free, open-source, remote MCP server for any GitHub project
Reviews
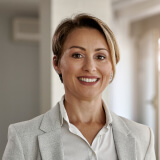
user_IAkHrdkO
As a dedicated user of the mcp-rust-sdk, I can confidently say it's a must-have for developers working with Rust. Derek-X-Wang has done a fantastic job with this SDK, providing a seamless and efficient way to integrate MCP functionalities. The clear documentation and ease of use make it a top choice for any project. Check it out at https://github.com/Derek-X-Wang/mcp-rust-sdk - you won't be disappointed!