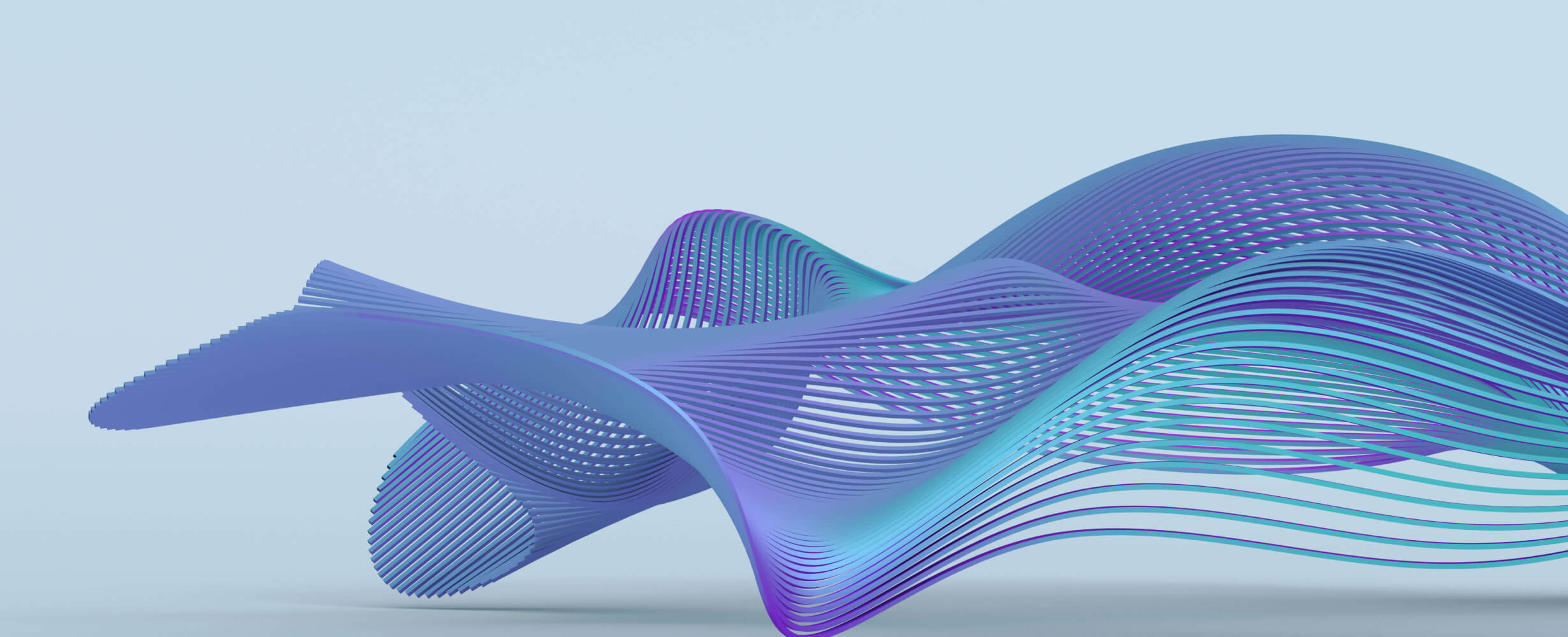
mcp-congress_gov_server
This is a Model Context Protocol (MCP) server designed to provide access to the official Congress.gov API (v3) using a hybrid approach.
3 years
Works with Finder
1
Github Watches
0
Github Forks
0
Github Stars
Congress.gov API MCP Server
This is a Model Context Protocol (MCP) server designed to provide access to the official Congress.gov API (v3) using a hybrid approach:
- MCP Resources: For direct lookups of core legislative entities (Bills, Members, Congresses, Committees, general Info) using standardized URIs.
-
MCP Tools: For more complex operations like searching across collections (
congress_search
) and retrieving related data lists (congress_getSubResource
).
This server acts as a bridge, allowing MCP clients (like AI assistants or development tools) to easily query and utilize U.S. legislative data.
Project Structure
-
/src
: Contains all source code.-
/config
: Configuration management (ConfigurationManager.ts
). -
/services
: Core logic for interacting with Congress.gov API (CongressApiService.ts
,RateLimitService.ts
). -
/tools
: MCP tool definitions (search/
,subresource/
,index.ts
). -
/types
: TypeScript interfaces and Zod schemas. -
/utils
: Shared utility functions (logging, errors, etc.). -
resourceHandlers.ts
: Logic for handling core entity resource requests. -
createServer.ts
: Server instance creation, resource and tool registration. -
server.ts
: Main application entry point.
-
-
/dist
: Compiled JavaScript output (generated bynpm run build
). -
/docs
: Project documentation (PRD, Feature Spec, RFCs). -
package.json
: Project metadata and dependencies. -
tsconfig.json
: TypeScript compiler options. -
.eslintrc.json
,.prettierrc.json
: Linting and formatting rules. -
.env
: (Not committed) For storingCONGRESS_GOV_API_KEY
.
Getting Started
-
Install Dependencies:
npm install
-
Set API Key: Create a
.env
file in the project root and add your Congress.gov API key:CONGRESS_GOV_API_KEY=YOUR_API_KEY_HERE
(Get a key from https://api.data.gov/signup/)
-
Build the Server:
npm run build
-
Run the Server:
npm start
(This runs
node dist/server.js
)
Alternatively, run in development mode using npm run dev
(uses ts-node
and nodemon
).
Usage with MCP Client
Connect your MCP client to the running server (e.g., via stdio if running locally).
Accessing Resources
Use the access_mcp_resource
command/method with the appropriate URI.
Examples:
-
Get Bill H.R. 3076 (117th Congress):
<access_mcp_resource> <server_name>congress-server</server_name> <uri>congress-gov://bill/117/hr/3076</uri> </access_mcp_resource>
-
Get Member Pelosi:
<access_mcp_resource> <server_name>congress-server</server_name> <uri>congress-gov://member/P000197</uri> </access_mcp_resource>
-
Get Info about 118th Congress:
<access_mcp_resource> <server_name>congress-server</server_name> <uri>congress-gov://congress/118</uri> </access_mcp_resource>
-
Get API Overview:
<access_mcp_resource> <server_name>congress-server</server_name> <uri>congress-gov://info/overview</uri> </access_mcp_resource>
Using Tools
Use the use_mcp_tool
command/method.
!!! CRITICAL TOOL WORKFLOW: Finding Entities & Getting Related Data !!!
Many common tasks require a mandatory two-step process using both tools:
-
STEP 1: Find the Entity ID using
congress_search
-
Purpose: Locate the specific bill, member, committee, etc., you need and extract its unique identifier(s) (e.g.,
memberId
, or thecongress
,billType
,billNumber
for a bill URI). -
Tool:
congress_search
-
Example: Find member "John Kennedy" (might return multiple results requiring selection):
<use_mcp_tool> <server_name>congress-server</server_name> <tool_name>congress_search</tool_name> <arguments> { "collection": "member", "query": "John Kennedy" } </arguments> </use_mcp_tool>
-
Output: Look for the
memberId
(e.g.,K000393
) or other necessary identifiers in the results. -
!!! WARNING !!! Searching might return multiple results. You MUST identify the correct entity and use its specific ID for the next step.
-
!!! API LIMITATION !!! Filtering general searches by
congress
using thefilters
parameter is NOT SUPPORTED by the underlying API (e.g., for/v3/bill
or/v3/member
) and will be ignored. Congress-specific filtering usually requires using specific API paths (e.g.,/v3/bill/117
), which this tool does not construct.
-
-
STEP 2: Get Related Data using
congress_getSubResource
-
Purpose: Use the identifier(s) found in Step 1 to construct the
parentUri
and fetch related details (actions, sponsors, text, etc.). -
Tool:
congress_getSubResource
-
Prerequisite: You MUST have the correct
parentUri
(e.g.,congress-gov://member/K000393
) from Step 1. -
Example: Get legislation sponsored by member
K000393
:<use_mcp_tool> <server_name>congress-server</server_name> <tool_name>congress_getSubResource</tool_name> <arguments> { "parentUri": "congress-gov://member/K000393", "subResource": "sponsored-legislation", "limit": 5 } </arguments> </use_mcp_tool>
-
!!! GUARANTEED ERROR WARNING !!! You MUST use a
subResource
string that is STRICTLY VALID for theparentUri
type (e.g.,'sponsored-legislation'
for members,'actions'
for bills). Providing an invalid combination WILL cause an error. Check the tool description for valid combinations.
-
Following this two-step process is ESSENTIAL for reliably getting related information.
Tool Examples:
-
Search for Bills containing "climate" (limit 5):
<use_mcp_tool> <server_name>congress-server</server_name> <tool_name>congress_search</tool_name> <arguments> { "collection": "bill", "query": "climate", "limit": 5 } </arguments> </use_mcp_tool>
-
List Members (No Congress Filter Possible Here):
-
Note: As mentioned above, filtering by
congress
directly incongress_search
is not supported by the API for the 'member' collection.<use_mcp_tool> <server_name>congress-server</server_name> <tool_name>congress_search</tool_name> <arguments> { "collection": "member", "limit": 10 // Add "query" or other filters like "type" if needed } </arguments> </use_mcp_tool>
-
-
Get Actions for Bill H.R. 3076 (117th) (Requires URI from Search or Known Info):
<use_mcp_tool> <server_name>congress-server</server_name> <tool_name>congress_getSubResource</tool_name> <arguments> { "parentUri": "congress-gov://bill/117/hr/3076", "subResource": "actions", "limit": 10 } </arguments> </use_mcp_tool>
-
Get Legislation Sponsored by Member P000197:
<use_mcp_tool> <server_name>congress-server</server_name> <tool_name>congress_getSubResource</tool_name> <arguments> { "parentUri": "congress-gov://member/P000197", "subResource": "sponsored-legislation", "limit": 5 } </arguments> </use_mcp_tool>
Linting and Formatting
-
Lint:
npm run lint
-
Format:
npm run format
Code will be automatically linted and formatted on commit via Husky and lint-staged.
相关推荐
I find academic articles and books for research and literature reviews.
Converts Figma frames into front-end code for various mobile frameworks.
Confidential guide on numerology and astrology, based of GG33 Public information
Embark on a thrilling diplomatic quest across a galaxy on the brink of war. Navigate complex politics and alien cultures to forge peace and avert catastrophe in this immersive interstellar adventure.
Advanced software engineer GPT that excels through nailing the basics.
Delivers concise Python code and interprets non-English comments
💬 MaxKB is a ready-to-use AI chatbot that integrates Retrieval-Augmented Generation (RAG) pipelines, supports robust workflows, and provides advanced MCP tool-use capabilities.
Micropython I2C-based manipulation of the MCP series GPIO expander, derived from Adafruit_MCP230xx
The all-in-one Desktop & Docker AI application with built-in RAG, AI agents, No-code agent builder, MCP compatibility, and more.
Reviews
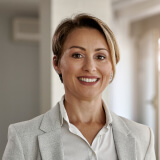
user_dd1oqyO0
The mcp-congress_gov_server by bsmi021 is a remarkable tool for anyone interested in congressional data. Highly efficient and user-friendly, it simplifies the process of fetching and analyzing legislative information. Its seamless integration and robust performance set it apart in the realm of government data applications. Highly recommended!