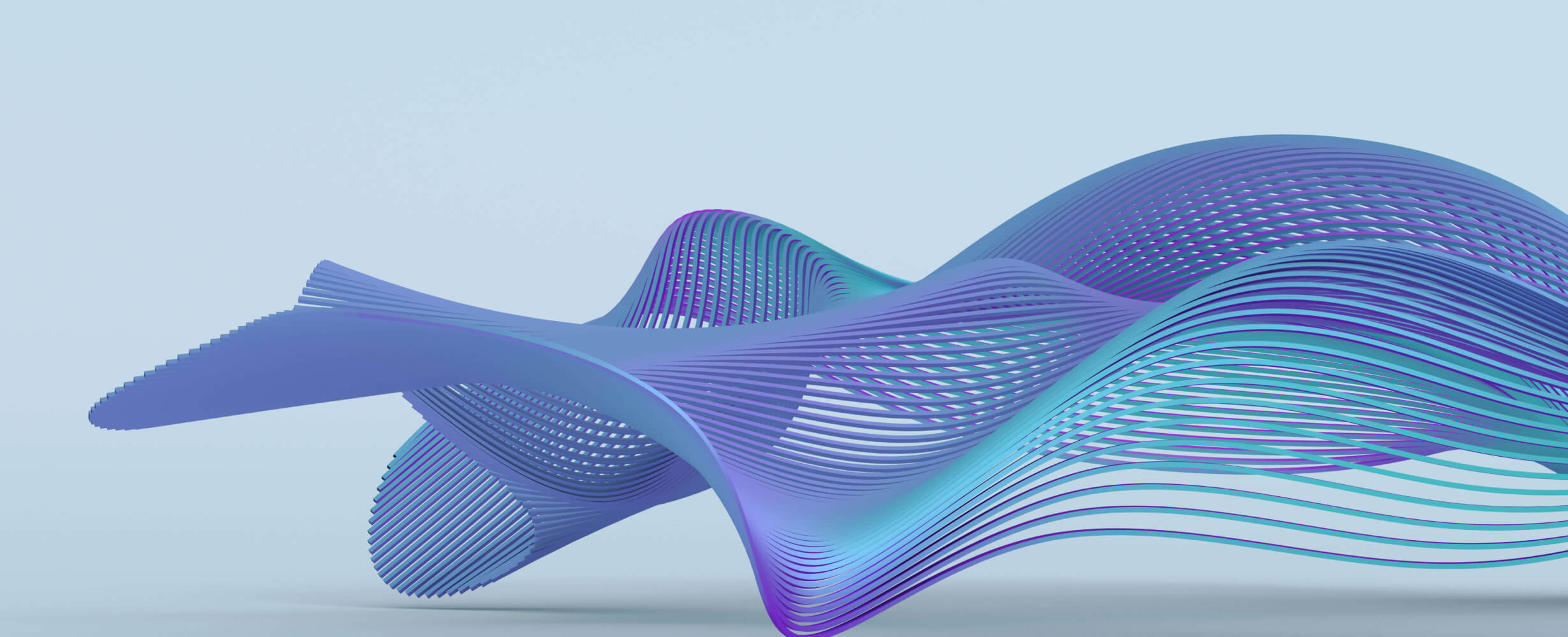
mcp-server
This is a repository for mcp servers
3 years
Works with Finder
1
Github Watches
0
Github Forks
0
Github Stars
MCP Server
A Model Context Protocol (MCP) server implementation that provides various tools for AI agents and LLMs, including search capabilities powered by SearXNG and file system operations.
Features
- Web Search: Perform web searches with customizable parameters using SearXNG
- File System Operations: Read, write, and manage files with security controls
- Extensible Architecture: Add custom tools following the MCP protocol
- Docker Support: Easy deployment with Docker and docker-compose
- Command-line Interface: Simple management through CLI
- Resources and Prompts: Full MCP specification support for resources and prompts
Requirements
- Python 3.13 (required)
- MCP SDK ≥ 1.6.0
- UV package manager for dependency management
- Docker (optional, for containerized deployment)
Implementation Approaches
This project demonstrates two approaches to implementing MCP servers:
1. FastMCP Decorator Approach (Recommended)
The simplified approach using FastMCP decorators in mcp_server/server.py
:
from mcp.server.fastmcp import FastMCP
# Create server
mcp = FastMCP("MCP Server")
# Add a tool using decorator
@mcp.tool()
def search(query: str, num_results: int = 5) -> dict:
"""Search the web"""
# Implementation...
return results
# Add a resource
@mcp.resource("greeting://{name}")
def get_greeting(name: str) -> str:
"""Get a greeting"""
return f"Hello, {name}!"
# Add a prompt
@mcp.prompt()
def search_prompt(query: str) -> str:
"""Create a search prompt"""
return f"Please search for: {query}"
2. Original Architecture (Advanced)
The original architecture in mcp_server/core/
provides a more complex implementation with:
-
ToolRegistry
for managing tool registration -
MCPTool
abstract base class for tool implementation - Singleton
MCPServer
for server management
This approach offers more flexibility for complex applications but requires more boilerplate code.
Tools
search
Execute web searches through SearXNG with customizable filtering.
Inputs:
-
query
(string): Search terms -
num_results
(number, optional): Number of results to return (default: 5, max: 20) -
language
(string, optional): Language code for results (e.g., "en", "fr") -
time_range
(string, optional): Time range for results (day, week, month, year) -
search_category
(string, optional): Category to search in (general, images, news, videos)
filesystem
Read, write, and manage files with secure access controls.
Operations:
-
Read File
-
path
(string): Path to the file to read -
encoding
(string, optional): Encoding to use (default: "utf-8") -
binary
(boolean, optional): Whether to read in binary mode (default: false)
-
-
Write File
-
path
(string): Path to write the file -
content
(string): Content to write -
encoding
(string, optional): Encoding to use (default: "utf-8") -
binary
(boolean, optional): Whether to write in binary mode (default: false) -
append
(boolean, optional): Whether to append to file (default: false)
-
-
List Files
-
path
(string, optional): Directory to list (default: current directory) -
recursive
(boolean, optional): Whether to list recursively (default: false) -
pattern
(string, optional): Pattern to match filenames
-
Configuration
Environment Variables
Configure your MCP server using environment variables:
# Server settings
MCP_SERVER__HOST=0.0.0.0
MCP_SERVER__PORT=8000
# SearXNG settings
MCP_SEARXNG__INSTANCE_URL=http://localhost:8080
MCP_SEARXNG__TIMEOUT=5
# Logging settings
MCP_LOG__LEVEL=INFO
# Data directory
MCP_DATA_DIR=./data
Usage with Claude Desktop
Option 1: Install Simple Example Server
# Install the simple example server directly
mcp install examples/server.py
# Or test with the MCP Inspector
mcp dev examples/server.py
Option 2: Docker Configuration
Add this to your claude_desktop_config.json
:
{
"mcpServers": {
"mcp-server": {
"command": "docker",
"args": [
"run",
"-i",
"--rm",
"-p",
"8000:8000",
"-e",
"MCP_SEARXNG__INSTANCE_URL=http://host.docker.internal:8080",
"mcp-server"
]
}
}
}
You'll need to run a SearXNG instance separately:
{
"mcpServers": {
"searxng": {
"command": "docker",
"args": [
"run",
"-i",
"--rm",
"-p",
"8080:8080",
"-e",
"BASE_URL=http://localhost:8080/",
"searxng/searxng"
]
}
}
}
Installation
Docker (Recommended)
# Build the Docker image
docker build -t mcp-server .
# Run the server and SearXNG
docker-compose up
Direct Installation
# Ensure you have Python 3.13 installed
python --version # Should show Python 3.13.x
# Install uv if you don't have it
pip install uv
# Create a virtual environment and install dependencies
uv venv --python=python3.13
uv install
# Run the server
python -m mcp_server start
Client Examples
See the examples/
directory for client examples:
-
examples/client.py
: Modern client usingClientSession
API -
examples/simple_client.py
: Simple client using direct API calls
Extending with Custom Tools
Using the FastMCP Approach
from mcp_server.server import mcp
@mcp.tool()
def my_custom_tool(param1: str, param2: int = 10) -> dict:
"""My custom tool description"""
# Implement tool functionality
return {"result": f"Processed {param1} with {param2}"}
Using the Original Architecture
See the Developer Guide for instructions on adding custom tools with the original architecture.
License
This project is licensed under the MIT License - see the LICENSE file for details.
Acknowledgements
- Model Context Protocol for the protocol specification
- SearXNG for the search engine functionality
Command to run the project
python -m mcp_server start --config config.env
相关推荐
Converts Figma frames into front-end code for various mobile frameworks.
I find academic articles and books for research and literature reviews.
Confidential guide on numerology and astrology, based of GG33 Public information
Embark on a thrilling diplomatic quest across a galaxy on the brink of war. Navigate complex politics and alien cultures to forge peace and avert catastrophe in this immersive interstellar adventure.
Advanced software engineer GPT that excels through nailing the basics.
Delivers concise Python code and interprets non-English comments
💬 MaxKB is a ready-to-use AI chatbot that integrates Retrieval-Augmented Generation (RAG) pipelines, supports robust workflows, and provides advanced MCP tool-use capabilities.
The all-in-one Desktop & Docker AI application with built-in RAG, AI agents, No-code agent builder, MCP compatibility, and more.
Micropython I2C-based manipulation of the MCP series GPIO expander, derived from Adafruit_MCP230xx
MCP server to provide Figma layout information to AI coding agents like Cursor
Reviews
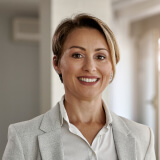
user_OVEfQKYm
MCP-Server by avinash539 is an outstanding tool for seamless server management and configuration. Its user-friendly interface and robust functionality make it a must-have for developers and IT professionals. The documentation is clear, and the support is top-notch. Highly recommended for anyone looking to streamline their server operations. Check it out [here](https://github.com/avinash539/mcp-server).