I craft unique cereal names, stories, and ridiculously cute Cereal Baby images.
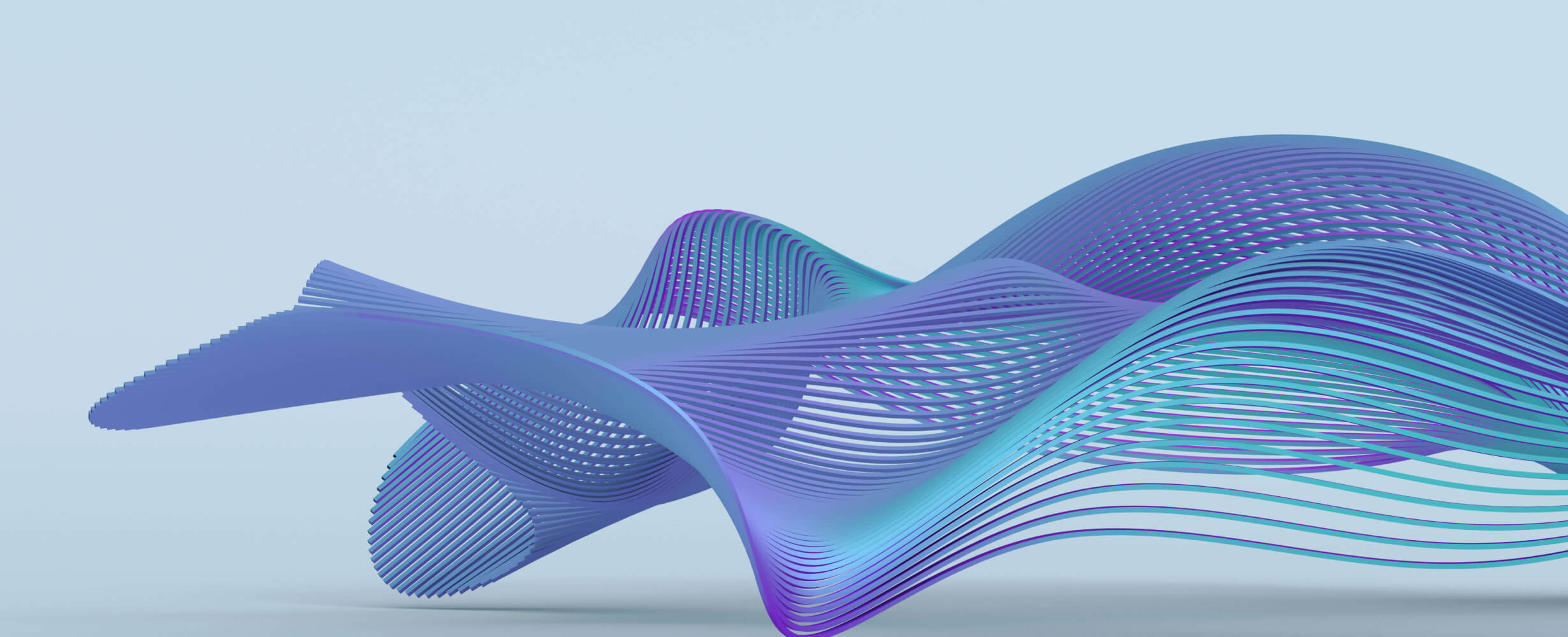
MCP-Server
A Model Context Protocol (MCP) server for PayPal API integration with improved error handling, comprehensive API coverage, and detailed documentation.
1
Github Watches
0
Github Forks
0
Github Stars
MCP Server (deprecated)
A Model Context Protocol (MCP) server that provides comprehensive integration with PayPal's APIs. This server enables seamless interaction with PayPal's payment processing, invoicing, subscription management, and business operations through a standardized interface.
Features
Payment Processing
- Orders Management: Create, update, and track orders
- Payment Processing: Process payments with various methods
- Payment Tokens: Create and manage payment tokens for future use
- Dispute Management: Handle payment disputes and resolutions
Business Operations
- Product Management: Create and manage product catalogs
- Invoicing: Generate and send professional invoices
- Payouts: Process batch payouts to multiple recipients
- Subscription Management: Create and manage recurring billing
User Management
- Identity Verification: Verify user identities
- User Information: Retrieve and manage user data
- Web Profile Management: Customize checkout experiences
Architecture
graph TB
subgraph "MCP Environment"
Client[MCP Client]
Server[PayPal MCP Server]
Validation[Input Validation]
Auth[OAuth Authentication]
Cache[Token Cache]
ErrorHandler[Error Handler]
end
subgraph "PayPal APIs"
Orders[Orders API]
Payments[Payments API]
Payouts[Payouts API]
Invoicing[Invoicing API]
Products[Products API]
Subscriptions[Subscriptions API]
Disputes[Disputes API]
Identity[Identity API]
end
Client --> |Request| Server
Server --> |Response| Client
Server --> Validation
Server --> Auth
Auth --> Cache
Auth --> |Access Token| PayPal
Server --> ErrorHandler
Server --> Orders
Server --> Payments
Server --> Payouts
Server --> Invoicing
Server --> Products
Server --> Subscriptions
Server --> Disputes
Server --> Identity
style Client fill:#f9f,stroke:#333,stroke-width:2px
style Server fill:#bbf,stroke:#333,stroke-width:2px
style Auth fill:#bfb,stroke:#333,stroke-width:2px
style Validation fill:#bfb,stroke:#333,stroke-width:2px
style Cache fill:#fbb,stroke:#333,stroke-width:2px
style ErrorHandler fill:#fbb,stroke:#333,stroke-width:2px
Installation
Prerequisites
- Node.js 16.x or later
- PayPal Developer Account with API credentials
Manual Installation
-
Clone the repository
git clone https://github.com/arbuthnot-eth/PayPal-MCP.git cd PayPal-MCP
-
Install dependencies
npm install
-
Build the project
npm run build
-
Configure PayPal credentials in your MCP settings file:
{ "mcpServers": { "paypal": { "command": "node", "args": ["path/to/paypal-mcp/build/index.js"], "env": { "PAYPAL_CLIENT_ID": "your_client_id", "PAYPAL_CLIENT_SECRET": "your_client_secret", "PAYPAL_ENVIRONMENT": "sandbox" // or "live" }, "disabled": false, "autoApprove": [] } } }
Available Tools
Payment Operations
create_payment_token
Create a payment token for future use.
{
customer: {
id: string;
email_address?: string;
};
payment_source: {
card?: {
name: string;
number: string;
expiry: string;
security_code: string;
};
paypal?: {
email_address: string;
};
};
}
create_order
Create a new order in PayPal.
{
intent: 'CAPTURE' | 'AUTHORIZE';
purchase_units: Array<{
amount: {
currency_code: string;
value: string;
};
description?: string;
reference_id?: string;
items?: Array<{
name: string;
quantity: string;
unit_amount: {
currency_code: string;
value: string;
};
}>;
}>;
application_context?: {
brand_name?: string;
shipping_preference?: 'GET_FROM_FILE' | 'NO_SHIPPING' | 'SET_PROVIDED_ADDRESS';
user_action?: 'CONTINUE' | 'PAY_NOW';
};
}
capture_order
Capture payment for an authorized order.
{
order_id: string;
payment_source?: {
token?: {
id: string;
type: string;
};
};
}
create_subscription
Create a subscription for recurring billing.
{
plan_id: string;
subscriber: {
name: {
given_name: string;
surname: string;
};
email_address: string;
};
application_context?: {
brand_name?: string;
shipping_preference?: 'GET_FROM_FILE' | 'NO_SHIPPING' | 'SET_PROVIDED_ADDRESS';
user_action?: 'CONTINUE' | 'SUBSCRIBE_NOW';
payment_method?: {
payer_selected?: string;
payee_preferred?: string;
};
};
}
Business Operations
create_product
Create a new product in the catalog.
{
name: string;
description: string;
type: 'PHYSICAL' | 'DIGITAL' | 'SERVICE';
category: string;
image_url?: string;
home_url?: string;
}
create_invoice
Generate a new invoice.
{
invoice_number: string;
reference: string;
currency_code: string;
recipient_email: string;
items: Array<{
name: string;
quantity: string;
unit_amount: {
currency_code: string;
value: string;
};
description?: string;
tax?: {
name: string;
percent: string;
};
}>;
note?: string;
terms_and_conditions?: string;
memo?: string;
payment_term?: {
term_type: 'DUE_ON_RECEIPT' | 'DUE_ON_DATE' | 'NET_10' | 'NET_15' | 'NET_30' | 'NET_45' | 'NET_60' | 'NET_90';
due_date?: string;
};
}
create_payout
Process a batch payout.
{
sender_batch_header: {
sender_batch_id: string;
email_subject?: string;
recipient_type?: string;
};
items: Array<{
recipient_type: string;
amount: {
value: string;
currency: string;
};
receiver: string;
note?: string;
}>;
}
Error Handling
The server implements comprehensive error handling:
- Input Validation: Detailed validation errors with specific messages
- PayPal API Errors: Structured error responses with PayPal error details
- Network Errors: Retry logic for transient network issues
- Authentication Errors: Automatic token refresh and clear error messages
- Rate Limiting: Backoff strategies for API rate limits
Security Considerations
- All sensitive data is validated and sanitized
- OAuth 2.0 authentication with PayPal
- Secure credential management through environment variables
- Input validation for all API parameters
- Error messages don't expose sensitive information
Development
Building
npm run build
Running in Development Mode
npm run dev
Testing
npm test
Linting
npm run lint
Formatting
npm run format
Contributing
- Fork the repository
- Create a feature branch
- Commit your changes
- Push to the branch
- Create a Pull Request
License
MIT License
相关推荐
Evaluator for marketplace product descriptions, checks for relevancy and keyword stuffing.
I find academic articles and books for research and literature reviews.
Confidential guide on numerology and astrology, based of GG33 Public information
Converts Figma frames into front-end code for various mobile frameworks.
Advanced software engineer GPT that excels through nailing the basics.
Take an adjectivised noun, and create images making it progressively more adjective!
Discover the most comprehensive and up-to-date collection of MCP servers in the market. This repository serves as a centralized hub, offering an extensive catalog of open-source and proprietary MCP servers, complete with features, documentation links, and contributors.
Micropython I2C-based manipulation of the MCP series GPIO expander, derived from Adafruit_MCP230xx
Mirror ofhttps://github.com/agentience/practices_mcp_server
A unified API gateway for integrating multiple etherscan-like blockchain explorer APIs with Model Context Protocol (MCP) support for AI assistants.
The all-in-one Desktop & Docker AI application with built-in RAG, AI agents, No-code agent builder, MCP compatibility, and more.
Reviews
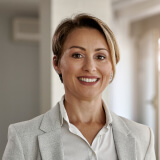
user_oG4PQfwb
I've been using the PHP MCP Protocol Server by Lucasdoreac and it has significantly improved my workflow. The server is robust and handles protocols efficiently, making it an essential tool in my development kit. Highly recommended for anyone in need of a reliable protocol server. Check it out here: https://mcp.so/server/php-mcp-protocol-server/Lucasdoreac