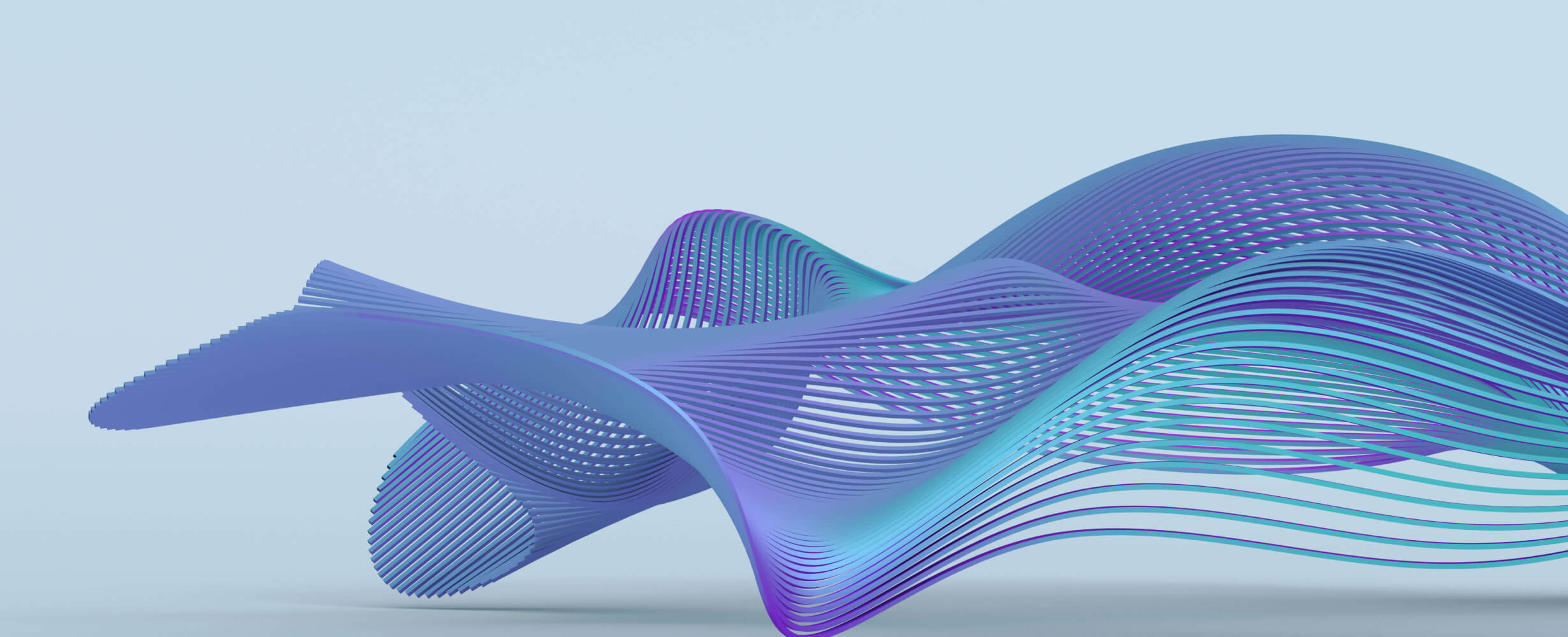
mcp-sdk
Minimalistic Rust Implementation Of Model Context Protocol from Anthropic
3 years
Works with Finder
2
Github Watches
8
Github Forks
54
Github Stars
Model Context Protocol (MCP)
Minimalistic Rust Implementation Of Model Context Protocol(MCP).
Learn more about MCP: MCP
Minimalistic approach
Given it is still very early stage of MCP adoption, the goal is to remain agile and easy to understand. This implementation aims to capture the core idea of MCP while maintaining compatibility with Claude Desktop. Many optional features are not implemented yet.
Some guidelines:
- use primitive building blocks and avoid framework if possible
- keep it simple and stupid
Examples
Tools Example
Using a Tool
trait for better compile time reusability.
impl Tool for CreateEntitiesTool {
fn name(&self) -> String {
"create_entities".to_string()
}
fn description(&self) -> String {
"Create multiple new entities".to_string()
}
fn input_schema(&self) -> serde_json::Value {
json!({
"type":"object",
"properties":{
"entities":{
"type":"array",
"items":{
"type":"object",
"properties":{
"name":{"type":"string"},
"entityType":{"type":"string"},
"observations":{
"type":"array", "items":{"type":"string"}
}
},
"required":["name","entityType","observations"]
}
}
},
"required":["entities"]
})
}
fn call(&self, input: Option<serde_json::Value>) -> Result<CallToolResponse> {
let args = input.unwrap_or_default();
let entities = args
.get("entities")
.ok_or(anyhow::anyhow!("missing arguments `entities`"))?;
let entities: Vec<Entity> = serde_json::from_value(entities.clone())?;
let created = self.kg.lock().unwrap().create_entities(entities)?;
self.kg
.lock()
.unwrap()
.save_to_file(&self.memory_file_path)?;
Ok(CallToolResponse {
content: vec![ToolResponseContent::Text {
text: json!(created).to_string(),
}],
is_error: None,
meta: None,
})
}
}
Server Example
let server = Server::builder(StdioTransport)
.capabilities(ServerCapabilities {
tools: Some(json!({})),
..Default::default()
})
.request_handler("tools/list", list_tools)
.request_handler("tools/call", call_tool)
.request_handler("resources/list", |_req: ListRequest| {
Ok(ResourcesListResponse {
resources: vec![],
next_cursor: None,
meta: None,
})
})
.build();
- See examples/file_system/README.md for usage examples and documentation
Client Example
#[tokio::main]
async fn main() -> Result<()> {
#[cfg(unix)]
{
// Create transport connected to cat command which will stay alive
let transport = ClientStdioTransport::new("cat", &[])?;
// Open transport
transport.open()?;
let client = ClientBuilder::new(transport).build();
let client_clone = client.clone();
tokio::spawn(async move { client_clone.start().await });
let response = client
.request(
"echo",
None,
RequestOptions::default().timeout(Duration::from_secs(1)),
)
.await?;
println!("{:?}", response);
}
#[cfg(windows)]
{
println!("Windows is not supported yet");
}
Ok(())
}
Other Sdks
Official
Community
For complete feature please refer to the MCP specification.
Features
Basic Protocol
- Basic Message Types
- Error and Signal Handling
- Transport
- Stdio
- In Memory Channel (not yet supported in formal specification)
- SSE
- More compact serialization format (not yet supported in formal specification)
- Utilities
- Ping
- Cancellation
- Progress
Server
- Tools
- Prompts
- Resources
- Pagination
- Completion
Client
For now use claude desktop as client.
Monitoring
- Logging
- Metrics
相关推荐
I find academic articles and books for research and literature reviews.
Converts Figma frames into front-end code for various mobile frameworks.
Confidential guide on numerology and astrology, based of GG33 Public information
Embark on a thrilling diplomatic quest across a galaxy on the brink of war. Navigate complex politics and alien cultures to forge peace and avert catastrophe in this immersive interstellar adventure.
Advanced software engineer GPT that excels through nailing the basics.
Delivers concise Python code and interprets non-English comments
💬 MaxKB is a ready-to-use AI chatbot that integrates Retrieval-Augmented Generation (RAG) pipelines, supports robust workflows, and provides advanced MCP tool-use capabilities.
Micropython I2C-based manipulation of the MCP series GPIO expander, derived from Adafruit_MCP230xx
The all-in-one Desktop & Docker AI application with built-in RAG, AI agents, No-code agent builder, MCP compatibility, and more.
MCP server to provide Figma layout information to AI coding agents like Cursor
Python code to use the MCP3008 analog to digital converter with a Raspberry Pi or BeagleBone black.
Reviews
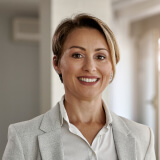
user_ZJF0GlBn
I have been using the mcp-sdk by AntigmaLabs for a while now, and it has significantly streamlined my development process. The documentation is clear and the GitHub repository is well-maintained. If you're embarking on a project that requires reliable and efficient components, I highly recommend checking out the mcp-sdk. The community support is also robust, making it easier to troubleshoot and collaborate.