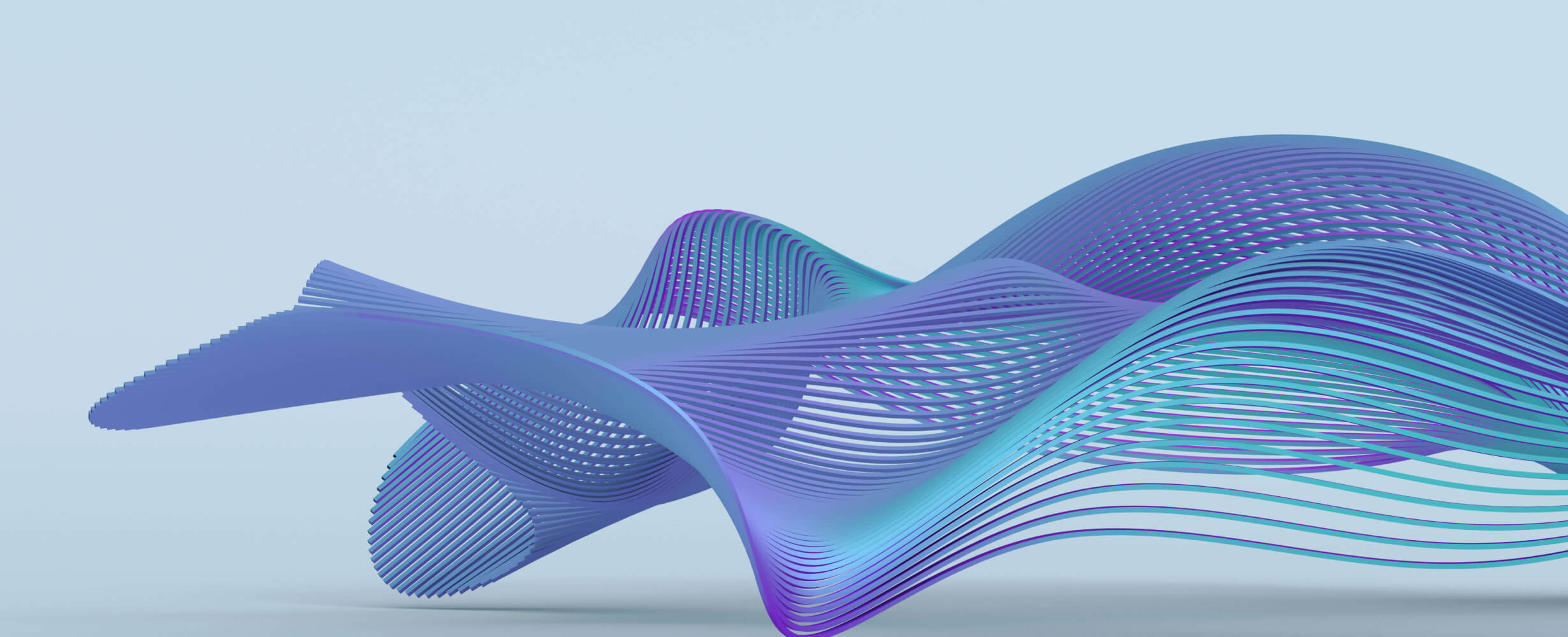
Building a CSS Tutor MCP Server
This repo contains a simple Model Context Protocol (MCP) server built with Node.js and TypeScript. It acts as a "CSS Tutor," providing personalized updates about CSS features to a connected AI client.
This server demonstrates key MCP concepts: defining Resources, Tools, and Prompts. The goal of this demonstration is to help you move on from here and build much larger and more interesting agentic capabilities.
Prerequisites
- Node.js (v18 or later recommended)
-
npm
(or your preferred Node.js package manager likeyarn
orpnpm
) - An AI client capable of connecting to an MCP server (e.g., the Claude desktop app)
- An OpenRouter API Key (for fetching live CSS updates via Perplexity)
Quick Start
Follow these steps to get the server running quickly:
-
Clone the Repository:
git clone https://github.com/3mdistal/css-mcp-server.git cd css-mcp-server
-
Install Dependencies:
npm install # Or: yarn install / pnpm install
-
Prepare API Key: The
get_latest_updates
tool requires an OpenRouter API key. Obtain your key from OpenRouter. You will provide this key to your MCP client in Step 5. -
Build the Server: Compile the TypeScript code.
npm run build # Or: yarn build / pnpm run build
-
Configure Your MCP Client: Tell your client how to launch the server and provide the API key as an environment variable. Here's an example for the Claude desktop app's
claude_desktop_config.json
:{ "mcpServers": { "css-tutor": { "command": "node", "args": [ "/full/path/to/your/css-mcp-server/build/index.js" ], "env": { "OPENROUTER_API_KEY": "sk-or-xxxxxxxxxxxxxxxxxxxxxxxxxx" } } } }
(Ensure the path in
args
is the correct absolute path to the builtindex.js
file on your system. Replace the placeholder API key.) -
Connect: Start the connection from your MCP client. The client will launch the server process (with the API key in its environment), and you can start interacting!
Using with Cursor
Cursor is an AI-first code editor that can act as an MCP client. Setting up this server with Cursor is straightforward, but requires an extra step for the guidance prompt.
-
Configure Server in Cursor:
- Go to
Cursor Settings
>MCP
>Add new global MCP server
. - Paste in the same JSON as above in the Claude Desktop step, with all the same caveats.
- Go to
-
Create a Cursor Project Rule for the Prompt: Cursor currently does not automatically use MCP prompts provided by servers. Instead, you need to provide the guidance using Cursor's Project Rules feature.
-
Create the directory
.cursor/rules
in your project root if it doesn't exist. -
Create a file inside it named
css-tutor.rule
(or any.rule
filename). -
Paste the following guidance text into
css-tutor.rule
:You are a helpful assistant connecting to a CSS knowledge server. Your goal is to provide the user with personalized updates about new CSS features they haven't learned yet. Available Tools: 1. `get_latest_updates`: Fetches recent general news and articles about CSS. Use this first to see what's new. 2. `read_from_memory`: Checks which CSS concepts the user already knows based on their stored knowledge profile. 3. `write_to_memory`: Updates the user's knowledge profile. Use this when the user confirms they have learned or already know a specific CSS concept mentioned in an update. Workflow: 1. Call `get_latest_updates` to discover recent CSS developments. 2. Call `read_from_memory` to get the user's current known concepts (if any). 3. Compare the updates with the known concepts (if any). Identify 1-2 *new* concepts relevant to the user. **Important: They _must_ be from the response returned by `get_latest_updates` tool.** 4. Present these new concepts to the user, adding any context as needed, in addition to the information returned by the `get_latest_updates`. 5. Ask the user if they are familiar with these concepts or if they've learned them now. 6. If the user confirms knowledge of a concept, call `write_to_memory` to update their profile for that specific concept. 7. Focus on providing actionable, personalized learning updates.
-
-
Connect and Use:
- Ensure the
css-tutor
server is enabled in Cursor's MCP settings. - Start a new chat or code generation request (e.g., Cmd+K) and include
@css-tutor-rule
(or whatever you named your rule file) in your request. This tells Cursor to load the rule's content, which includes the instructions on how to use theread_from_memory
,write_to_memory
, andget_latest_updates
tools provided by the connected MCP server.
- Ensure the
Note that without the prompt/rule, Cursor will still be able to use individual tools if you ask it to. The prompt provides a workflow and order in which to call the tools and read/write from memory.
Understanding the Code
This section provides a higher-level overview of how the server is implemented.
MCP Concepts Used
-
Resource (
css_knowledge_memory
): Represents the user's known CSS concepts, stored persistently indata/memory.json
. -
Tools: Actions the server can perform:
-
get_latest_updates
: Fetches CSS news from OpenRouter/Perplexity. -
read_from_memory
: Reads the content of thecss_knowledge_memory
resource. -
write_to_memory
: Modifies thecss_knowledge_memory
resource.
-
-
Prompt (
css-tutor-guidance
): Static instructions guiding the AI client on how to interact with the tools and resource effectively.
Code Structure
The code is organized as follows:
-
data/memory.json
: A simple JSON file acting as the database for known CSS concepts. A default version is included in the repo. -
src/resources/index.ts
: Defines thecss_knowledge_memory
resource. It includes:- A Zod schema for validating the data.
-
readMemory
andwriteMemory
functions for file I/O. - Registration using
server.resource
, specifying thememory://
URI scheme and read/write permissions. The read handler returns the content ofdata/memory.json
.
-
src/tools/index.ts
: Defines the three tools usingserver.tool
:-
read_from_memory
: CallsreadMemory
. -
write_to_memory
: Takesconcept
andknown
as input (schema defined with Zod), usesreadMemory
andwriteMemory
to update the JSON file. -
get_latest_updates
: RequiresOPENROUTER_API_KEY
, calls the OpenRouter API usingnode-fetch
and theperplexity/sonar-pro
model, returns the AI-generated summary.
-
-
src/prompts/index.ts
: Defines the staticcss-tutor-guidance
prompt usingserver.prompt
. The prompt text is embedded directly in the code. -
src/index.ts
: The main server entry point.- Initializes the
McpServer
instance from@modelcontextprotocol/sdk
. - Imports and calls the
registerPrompts
,registerResources
, andregisterTools
functions from the other modules. - Uses
StdioServerTransport
to handle communication over standard input/output. - Connects the server to the transport and includes basic error handling.
- Initializes the
-
package.json
: Defines dependencies (@modelcontextprotocol/sdk
,dotenv
,node-fetch
,zod
) and thebuild
script (tsc
). -
.env.example
/.env
: Used for storing theOPENROUTER_API_KEY
(if using Option A for configuration). -
.gitignore
: Configured to ignorenode_modules
,build
,.env
, and the contents ofdata/
except for the defaultdata/memory.json
. -
tsconfig.json
: Standard TypeScript configuration.
Debugging with MCP Inspector
If you need to debug the server or inspect the raw JSON-RPC messages being exchanged, you can use the @modelcontextprotocol/inspector
tool. This tool acts as a basic MCP client and launches your server, showing you the communication flow.
Run the inspector from your terminal in the project root:
npx @modelcontextprotocol/inspector node ./build/index.js
Explanation:
-
npx @modelcontextprotocol/inspector
: Downloads (if needed) and runs the inspector package. -
node
: The command used to execute your server. -
./build/index.js
: The path (relative to your project root) to your compiled server entry point.
Environment Variables for Inspector:
Note that the inspector launches your server as a child process. If your server relies on environment variables (like OPENROUTER_API_KEY
for the get_latest_updates
tool), you need to ensure they are available in the environment where you run the npx
command. The .env
file might not be automatically loaded in this context. You can typically prefix the command:
# Example on Linux/macOS
OPENROUTER_API_KEY="sk-or-xxxxxxxxxx" npx @modelcontextprotocol/inspector node ./build/index.js
# Example on Windows (Command Prompt)
set OPENROUTER_API_KEY=sk-or-xxxxxxxxxx && npx @modelcontextprotocol/inspector node ./build/index.js
# Example on Windows (PowerShell)
$env:OPENROUTER_API_KEY="sk-or-xxxxxxxxxx"; npx @modelcontextprotocol/inspector node ./build/index.js
Replace sk-or-xxxxxxxxxx
with your actual key.
Wrapping up
This demo demonstrates the core steps involved in creating a functional MCP server using the TypeScript SDK. We defined a resource to manage state, tools to perform actions (including interacting with an external API), and a prompt to guide the AI client.
Hope this demo can help you understand how to build servers that are much more complex (and useful) than this one!
(Also, if you run into any 🐛bugs, feel free to open up an issue.)
相关推荐
I find academic articles and books for research and literature reviews.
Emulating Dr. Jordan B. Peterson's style in providing life advice and insights.
Confidential guide on numerology and astrology, based of GG33 Public information
Your go-to expert in the Rust ecosystem, specializing in precise code interpretation, up-to-date crate version checking, and in-depth source code analysis. I offer accurate, context-aware insights for all your Rust programming questions.
Converts Figma frames into front-end code for various mobile frameworks.
Advanced software engineer GPT that excels through nailing the basics.
Take an adjectivised noun, and create images making it progressively more adjective!
Discover the most comprehensive and up-to-date collection of MCP servers in the market. This repository serves as a centralized hub, offering an extensive catalog of open-source and proprietary MCP servers, complete with features, documentation links, and contributors.
Micropython I2C-based manipulation of the MCP series GPIO expander, derived from Adafruit_MCP230xx
The all-in-one Desktop & Docker AI application with built-in RAG, AI agents, No-code agent builder, MCP compatibility, and more.
Bridge between Ollama and MCP servers, enabling local LLMs to use Model Context Protocol tools
Fair-code workflow automation platform with native AI capabilities. Combine visual building with custom code, self-host or cloud, 400+ integrations.
🧑🚀 全世界最好的LLM资料总结(Agent框架、辅助编程、数据处理、模型训练、模型推理、o1 模型、MCP、小语言模型、视觉语言模型) | Summary of the world's best LLM resources.
Reviews
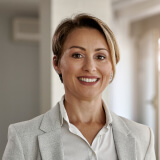
user_p2KQOLj4
I've been using css-mcp-server by 3mdistal and it’s simply amazing! It significantly enhances CSS management and has helped streamline my development process. The open-source nature and detailed documentation make it accessible for everyone. Highly recommend it! Check it out: https://github.com/3mdistal/css-mcp-server.